Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial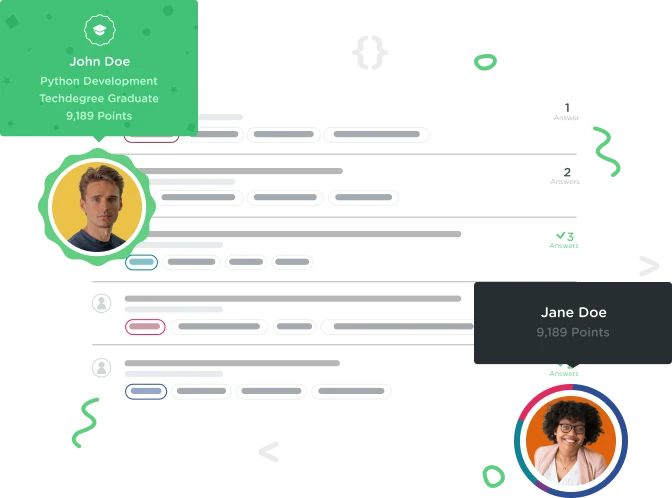
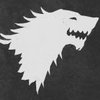
Jochem de Boer
6,536 Pointsa user can have a correctly formatted profile name: Failed assertion
Hey all,
I have a problem with the test: "a user can have a correctly formatted profile name". I get the "Failed assertion, no message given" error.
I used the new RegEx:
validates :profile_name, presence: true,
uniqueness: true,
format: {
with: /\A[a-zA-Z0-9_-]+\z/,
message: 'Must be formatted correctly.'
}
And I used dummy names for my email and profile name in the test:
test "a user can have a correctly formatted profile name" do
user = User.new(first_name: 'Mickey', last_name: 'Mouse', email: 'm.mouse@d.com')
user.password = user.password_confirmation = 'password'
user.profile_name = 'mickey'
assert user.valid?
end
I have looked around the forum, but none of the answers to this same topic have fixed it for me. Can anyone have a look and tell me if I am missing something?
Cheers
7 Answers

Gabriel Morales
11,315 Pointsvalidates :profile_name, presence: true,
uniqueness: true,
format: {
with: /\A[a-zA-Z0-9_-]+\Z/,
message: 'Must be formatted correctly.'
}
Here is what validated for me. Hope it helps.

Jason Seifer
Treehouse Guest TeacherTry changing the \z in the with regex to \Z and let us know if that helps.

Unsubscribed User
21,424 PointsThis worked for me, albeit in Rails 4

Robert Borsey
Courses Plus Student 9,636 Pointsdid you ever get it fixed because I was having the same problem I got mine to pass.
put the $ sign in any z will work don't know if this is the right thing or not but it worked for me
format: {
with: /\A[a-zA-Z0-9_-]+$\Z/,
message: "must be formatted correctly"
}

Unsubscribed User
21,424 PointsHere is my full validates
clause that appears to work:
validates :profile_name, presence: true,
uniqueness: true,
format: {
with: /\A[a-zA-Z0-9_-]+\z/,
message: "must be formatted correctly"
}
I have found Rubular to be nearly invaluable when working with regex in general, and especially with Ruby regex in particular.
I am using Ruby 2.x and Rails 4.x
Here are my tests:
test "a user should have a profile name without spaces" do
user = users(:bob)
user.profile_name = "spaced out!"
assert !user.save
assert !user.errors[:profile_name].empty?
assert user.errors[:profile_name].include?("must be formatted correctly")
end
test "a user should have be able to use a valid profile name" do
user = users(:bob)
assert user.save
assert user.errors[:profile_name].empty?
end
and here is my :bob
fixture:
bob:
first_name: "Bob"
last_name: "Barker"
profile_name: "bobb"
email: "bob.barker@example.com"
encrypted_password: "password1"
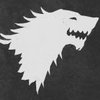
Jochem de Boer
6,536 PointsAlas, that did not help.

Tessa Davis
4,152 PointsI am having the exact same problems as this...any other suggestions?

Jason Seifer
Treehouse Guest TeacherTessa Davis can you possibly paste the error you are getting? Or a screenshot?