Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial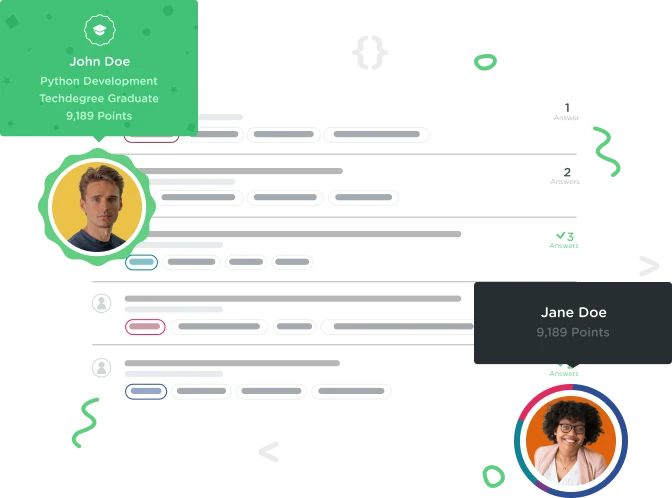

thomas shellberg
11,594 PointsA way to abstract the creation of the buttons(more DRY?)
Not a question but thought I'd share how I abstracted the creation of the buttons a bit in this code. I created a method which returned an element that has a class and textContent based on the parameters passed.
addElement = ( type, className, textContent ) => {
let element = document.createElement(type);
element.className = className;
element.textContent = textContent;
return element;
}
So, inside of a for loop for the children of the UL element, you can add a button with one line:
let removeButton = addElement('button', 'remove', 'X');
Where 'button' is the element type that the addElement method will create, 'remove' is the className added to the button(which our JS is listening for), and 'X' is just the text within the <button></button> element as a visual indicator to remove the element.
Hope that helps someone and always happy to hear feedback if this approach could be improved. :)
3 Answers
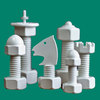
Steven Parker
231,846 PointsExcellent concept, and good execution! I would only suggest adding "var" or "const" to the creation of "addElement" instead of relying on the implicit global declaration.

RODRIGO MARTINEZ
5,912 PointsAswesome short solution. I understood this one much better than the one explained in the lesson. I'll certainly use this aproach and add it to my toolkit. Thanks a lot for helping out a begginer!
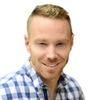
professionalb
Full Stack JavaScript Techdegree Student 5,082 PointsJust wanted to add that I love this line of thinking. I was watching a YT video last night about the "best way to learn JS'" and this was exactly what was suggested. The creator said it's helpful for you to sit down and right functions to create varying elements on a page. I theorize that we're writing these individual statements over and over because the course is utilizing repetition as a learning technique.
It'd be a cool 'challenge' to analyze a project first and create a dozen or so functions that would be useful in the creation of the main code body, essentially creating a mini library.