Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial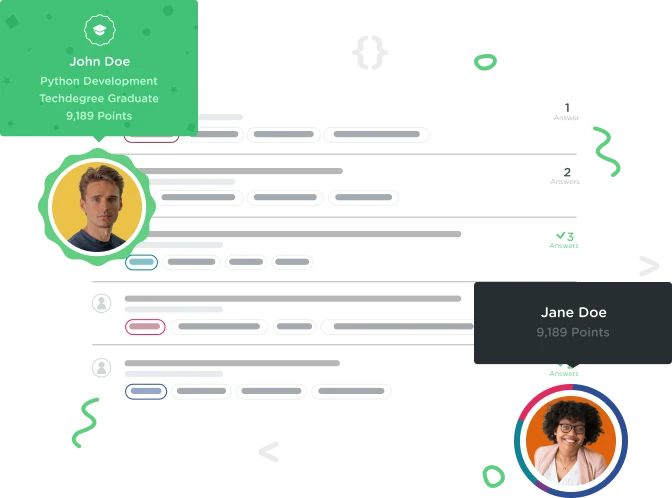

Sampath Kumar Maka
2,840 PointsAble to get the expected output in Playground but the same code is giving errors in the compiler
I have instantiated the objects as well and the same code compiled in the playground and I got the output as well but where as in the compiler here, it is throwing the errors.
Can anyone help me out please.
class Point {
var x: Int
var y: Int
init(x: Int, y: Int) {
self.x = x
self.y = y
}
}
class Machine {
var location: Point
init() {
self.location = Point(x: 0, y: 0)
}
func move(direction: String) {
print("Do nothing! I'm a machine!")
}
}
class Robot: Machine {
override func move(direction: String) {
switch direction {
case "Up" :
location.y += 1; break
case "Down":
location.y -= 1; break
case "Left" :
location.x -= 1; break
case "Right" :
location.x += 1; break
default:
break
}
}
}
3 Answers
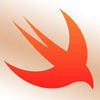
Jeff McDivitt
23,970 PointsYou only need a break statement at the end
class Point {
var x: Int
var y: Int
init(x: Int, y: Int) {
self.x = x
self.y = y
}
}
class Machine {
var location: Point
init() {
self.location = Point(x: 0, y: 0)
}
func move(_ direction: String) {
print("Do nothing! I'm a machine!")
}
}
class Robot: Machine{
override func move(_ direction: String) {
switch direction {
case "Up": location.y += 1
case "Down": location.y -= 1
case "Right": location.x += 1
case "Left": location.x -= 1
default:
break
}
}
}
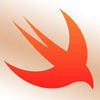
Jeff McDivitt
23,970 PointsHi Sampath - The code I posted will pass the challenge and is correct, it could also be because you have semicolons at the end of each return case statement.

Sampath Kumar Maka
2,840 PointsThanks Jeff, it worked.

Dhanish Gajjar
20,185 PointsSampath Kumar Maka The solution Jeff McDivitt gave, is correct. When the challenge loads, the function direction in the class Machine, has the external name omitted with an underscore. While overriding the same function in the class Robot, it also has to be omitted. Xcode hints at that error, however the challenge editor has no features like that. I don't understand why you removed it from the original class of Machine as well. Hope it helps.

Sampath Kumar Maka
2,840 PointsThanks Danish, it worked and I passed the challenge too.
Sampath Kumar Maka
2,840 PointsSampath Kumar Maka
2,840 PointsHi Jeff, thanks for the reply, but I have tried with single break also, even then it didnt pass the challenge, where as the same code was giving the expected output in the play ground.