Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial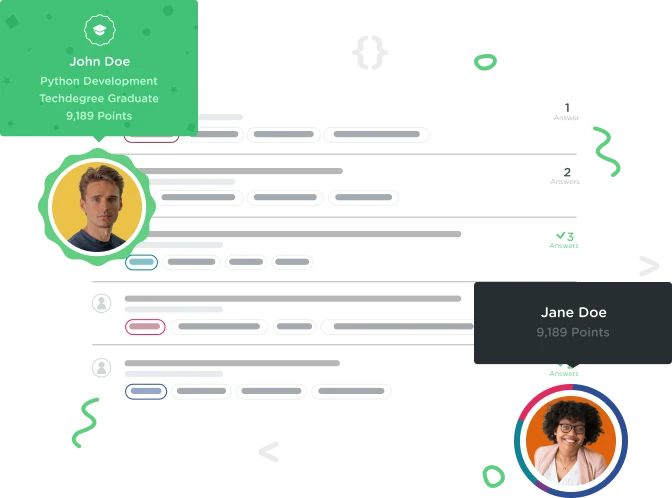
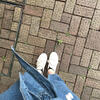
Tani Huang
6,952 PointsAbout removeChild
<div class="container">
<ul class="list reset">
<li>a</li>
<li>b</li>
<li>c</li>
<li>d</li>
<li>e</li>
</ul>
<button class="btn">Delete</button>
</div>
var container = document.querySelector (".container");
var list = document.querySelector(".list");
var li = document.querySelectorAll(".list li");
var btn = document.querySelector(".btn");
container.addEventListener ("click", (e) => {
if (e.target.tagName === "LI") {
let li = e.target;
list.removeChild(li);
}
});
btn.addEventListener ("click",() => {
list.removeChild(li);
});
After practicing "Event Bubbling" and back to try simple btn.addEventListener to control removeChild it always got fail, it show "The node to be remove is not a child of node"...but li is under ul, why is it not working ? I got confuse ..
1 Answer
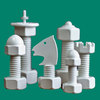
Steven Parker
230,274 PointsThe "querySelectorAll" function returns a collection, not a single element, so what's in "li" is not a valid argument to "removeChild".
If you change it to "querySelector" instead, the button will remove the first item.
And if you move the assignment into the handler, it will continue to remove the topmost item on subsequent clicks.
Tani Huang
6,952 PointsTani Huang
6,952 PointsThank you Steven, If I change it to "querySelector" it works, but why it only remove once.
Is that mean addEventListener always active once ? If it need to keep acting should always combine with for loop or event bubbling?
Steven Parker
230,274 PointsSteven Parker
230,274 PointsIt only removes the first item because "li" is assigned only once when the program loads. That's why I suggested moving that assignment into the handler where it will continue to pick the topmost item on each click.