Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial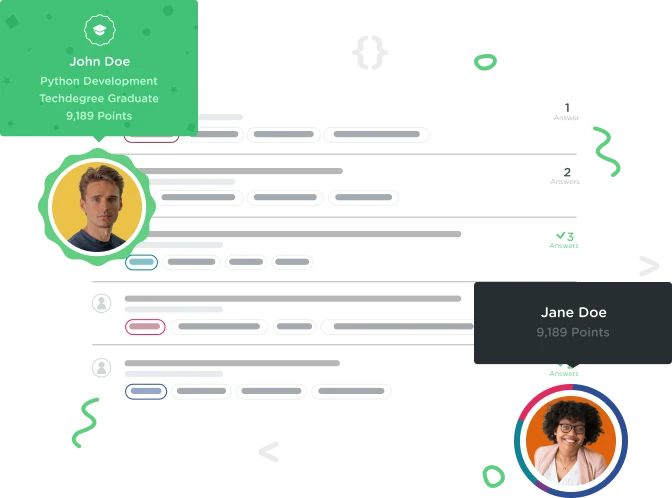
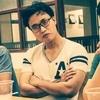
Hai Phan
2,442 PointsAbout the challenge
This is my code for the challenge:
def stringcases (string):
uppercase = string.upper()
lowercase = string.lower()
single_word = string.split()
titlecased = single_word[0]
for word in single_word[1::]:
titlecased += " " + word.capitalize()
temp = single_word[-1::-1]
reverse = " ".join(temp)
return (uppercase, lowercase, titlecased, reverse)
but I dont know why they said my reversed case is wrong. I've even tried this one to reverse every letter in a word:
list_of_string = list(string)
temp = list_of_string[-1::-1]
reverse = "".join(temp)
but it still wrong. Can someone help me to resolve this?
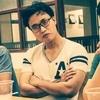
Hai Phan
2,442 PointsIt's Tuple challenge
1 Answer
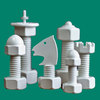
Steven Parker
231,269 PointsYour second version should handle the reversing, but you still have an issue with the title casing. The code here capitalizes each word individually except for the first word. But the challenge is expecting to see every word capitalized. Fix that, and you should pass the challenge.
But be aware that there are easier ways to accomplish both the title casing and the reversing. As the challenge hinted, "There are str
methods for all but the last one.", and for the reversing (the "last one"), slices can also be applied directly to strings, you don't need to convert them from/to lists.
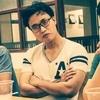
Hai Phan
2,442 PointsThank you Steve!
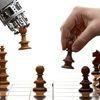
horus93
4,333 Pointsactually something to note there with the title casing, is that it's not that his code doesn't capitalize the first word, but it adds a second lower cased copy of the first word in front of the rest that have their first letter capitalized.
or, at least it does if I make a string variable and put a series of words through
Edit: nvm, steven was right about it being the first word getting missed, I just misread the situation when I changed the
for word in single_word[1::]:
# to 0 instead
for word in single_word[:]:
which just caused it to print capitalized first letters of all my string words, but also left a lower cased version of the first one at the front of the string, leaving me with an extra item for some reason
Brendan Whiting
Front End Web Development Techdegree Graduate 84,738 PointsBrendan Whiting
Front End Web Development Techdegree Graduate 84,738 PointsThis links to a video, which challenge is it?