Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial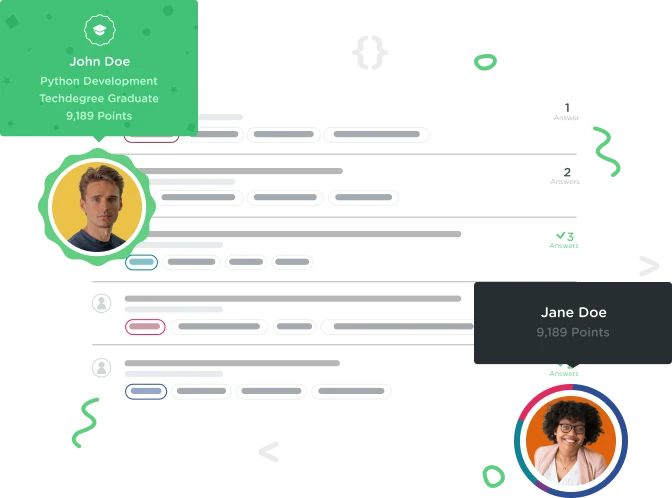

kelake
4,223 PointsAccess data in this array of class instances
This is the first time I have seen an array of this type. By now accessing and manipulating arrays is not conceptually a big deal but if we just have an array comprised of objects from single class how can we access the data contained within. Example code below:
class Cars {
var model: String
var fuelType: String
var cost: Int
var type: String
init(model: String, fuelType: String, cost: Int, type: String) {
self.cost = cost
self.fuelType = fuelType
self.model = model
self.type = type
}
}
var carCollection = [Cars(model: "Benz", fuelType: "Gas", cost: 100000, type: "luxury"), Cars(model: "Mercedes", fuelType: "Gas", cost: 110000, type:"sports")]
As an example, how can I access each Cars "fuelType" ? Also, when creating this array (or the array in the video) in Xcode it won't autocomplete.
1 Answer
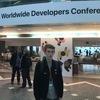
Jari Koopman
Python Web Development Techdegree Graduate 29,349 PointsHi Kelake,
Accessing something in an array of custom types, works the same as accessing a builtin type in an array. So, to get the fuel type of all cars, you could do something like this:
for car in carCollection {
print(car.fuelType)
// Do more things with the car
}
or, if you wanted to get the fuel type of, say, the first car, you could do this:
carCollection[0].fuelType
For your auto complete issues, Xcode is known to be a bit weird at times... For me personally, 90% of the times, a machine restart fixes the issues.
Hope this has helped enough,
Regards, Jari
kelake
4,223 Pointskelake
4,223 PointsThank you for your reply. This was my understanding. I was getting errors and I was stumped. Perhaps some silly mistake.
Jari Koopman
Python Web Development Techdegree Graduate 29,349 PointsJari Koopman
Python Web Development Techdegree Graduate 29,349 PointsNo problem! Glad I could help :)