Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial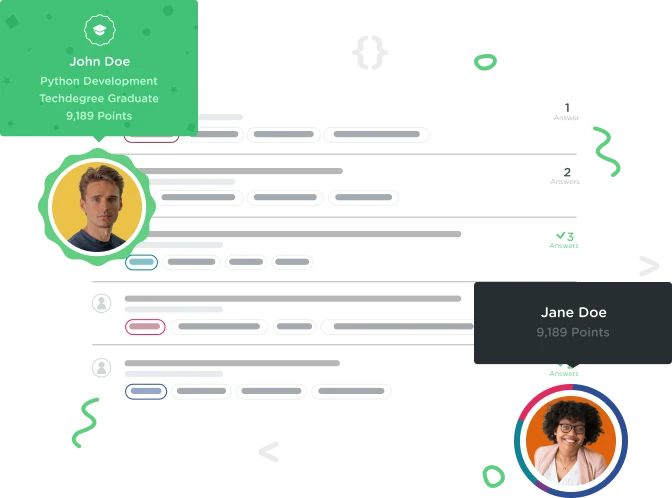

Lauren Inacio
2,871 Points'Access-Control-Allow-Origin' header has a value 'null' that is not equal to the supplied origin. Origin 'null' is there
Not sure what I'm doing wrong here, but when trying to fetch data from an API(https://jsonplaceholder.typicode.com/comments), it keeps failing for the below reason, which is my message in the console.
Failed to load https://jsonplaceholder.typicode.com/comments: The 'Access-Control-Allow-Origin' header has a value 'null' that is not equal to the supplied origin. Origin 'null' is therefore not allowed access. Have the server send the header with a valid value, or, if an opaque response serves your needs, set the request's mode to 'no-cors' to fetch the resource with CORS disabled.
Looks like there was a problem! TypeError: Failed to fetch app.js:12
I think the issue is occuring somewhere in my headers in the POST DATA commented section below is my code:
const select = document.getElementById('breeds');
const card = document.querySelector('.card');
const form = document.querySelector('form');
// ------------------------------------------
// FETCH FUNCTIONS
// ------------------------------------------
function fetchData (url){
return fetch(url)
.then(checkStatus)
.then(response => response.json())
.catch(error => console.log('Looks like there was a problem!', error))
}
//will fail if one fails..
Promise.all([
fetchData('https://dog.ceo/api/breeds/list'),
fetchData('https://dog.ceo/api/breeds/image/random')
])
.then(data => {
let breedList = data[0].message;
let randomImg = data[1].message;
generateOptions(breedList);
generateImage(randomImg);
})
//these fetchData were replaced with Promise.all
// fetchData('https://dog.ceo/api/breeds/list')
// .then(data => generateOptions(data.message))
// fetchData('https://dog.ceo/api/breeds/image/random')
// .then(data => generateImage(data.message))
// ------------------------------------------
// HELPER FUNCTIONS
// ------------------------------------------
function checkStatus (response) {
if(response.ok){
return Promise.resolve(response);
}else {
return Promise.reject(new Error(response.statusText));
}
}
function generateOptions (data) {
let options = data.map(item => `
<option value='${item}'>${item}</option>
`).join('');
select.innerHTML = options;
}
function generateImage (data) {
let html = `
<img src='${data}' alt="#">
<p>Click to view images of ${select.value}s</p>
`;
card.innerHTML = html;
}
function fetchBreedImage () {
let breed = select.value;
let img = card.querySelector('img');
let p = card.querySelector('p');
fetchData(`https://dog.ceo/api/breed/${breed}/images/random`)
.then(data =>{
img.src = data.message;
img.alt = breed;
p.textContent = `Click to view more ${breed}s`;
})
}
// ------------------------------------------
// EVENT LISTENERS
// ------------------------------------------
select.addEventListener('change', fetchBreedImage);
card.addEventListener('click', fetchBreedImage);
form.addEventListener('submit', postData);
// ------------------------------------------
// POST DATA
// ------------------------------------------
function postData (e){
e.preventDefault();
const name = document.getElementById('name').value;
const comment = document.getElementById('comment').value;
fetchData('https://jsonplaceholder.typicode.com/comments',{
methods: 'POST',
headers: {
'Content-Type': 'application/json/'
},
body: JSON.stringify({
name,
comment
})
});
}
2 Answers
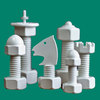
Steven Parker
231,236 PointsHave you tried the suggestion given in the error message? Add this to the init data object argument of the "fetch" call:
mode: 'no-cors',

jay aljoe
4,113 Points@Lauren Inacio you need a server. All you need to do is run your program on a live server like atom-live-server or any equivalent editor extensions.
Lauren Inacio
2,871 PointsLauren Inacio
2,871 PointsYes, I did try that and no luck...
I also tried a chrome extension for CORS issues, but nothing changed...not sure if that helps in any way getting closer to the answer, but figured I might as well mention it.
I'm probably going to facepalm myself after lol
I've also tried something like adding, Access-Control-Allow-Origin: *, to the headers....it didn't fix the problem either, but in reading the error that header is apparently set to null and because it has a value 'null' that is not equal to the supplied origin. Origin 'null' is therefore not allowed access. So, I tried to use the advice on MDN...https://developer.mozilla.org/en-US/docs/Web/HTTP/Headers/Access-Control-Allow-Origin
no luck so far :\