Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial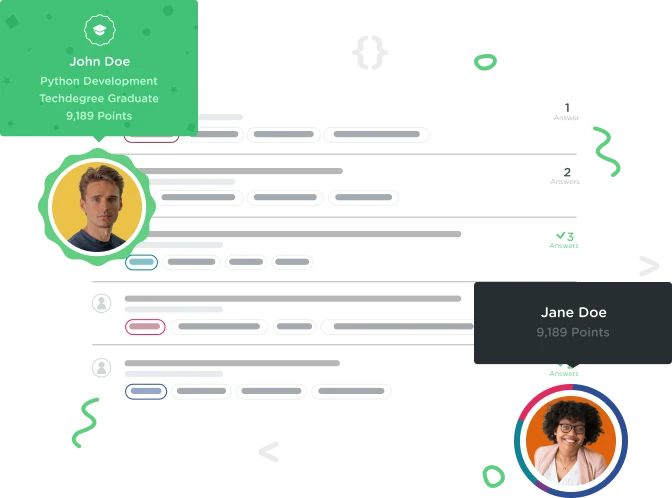

brandonlind2
7,823 PointsAccessing an objects method in a nested obj, feed back please
let obj={
log:function(){
console.log("it logged");
},
nestedObj: {
log1:function(){this.log()},/*returns error this.log is not a function,
which is understandable since this refers
to the nearest object*/
log2:function(){ obj.log()}/*returns error obj.log is not a function, I'm assuming
because I'm calling the object in its declaration so it
doesn't exit yet???? which is odd because, I thought
variable declarations are hoisted to the top leaving their
values unhoisted, so do objects not work the same way,
are they not hoisted??????????????*/
}
}
/*if I need to use an object's method in one of its nested object' s
methods, the only way I can think to do it would be like this*/
let obj={
log:function(){
console.log("it logged");
},
nestedObj: {}
}
obj.nestedObj.log2= function(){ obj.log()};
/*this seems slightly hacking though
MY QUESTIONS ARE (1)is there a reason objects aren't hoisted?
(2) The fact that I need to write it this way makes me think maybe is bad
practice, so is it bad practice?
and (3) since you cant use the this keyword in nested objects to access a
method in a parent obj, could this effect the codes reusablity?
(4)could it possibly have negative effects?
finally (5) is there possibly a better way of accomplishing this?*/
/*the reason I'm asking this is because I realized if I next any deeper object
in this currency declaration, I might lose access to the moneyConvert method,
which is a a bit odd because that makes me think there is a limit to encapsulation,
unless I'm misunderstanding something*/
let currency={
playerCopper: player.inventory.money.copper,
playerSilver: player.inventory.money.silver,
playerGold: player.inventory.money.gold,
moneyConvert: ()=>{
if(this.playerCopper<=100) {
this.playerCopper-=100;
this.playerSilver+=1;
}
else if(this.playerSilver<=100){
this.playerSilver-=100;
this.playerGold+=1;
}
},
copper: {
addCopper: (to,amount)=>{
if(to==="player"){
this.playerCopper+=amount;
}
else{
to+=amount;
}
this.moneyConvert();
}
},
silver:{
addSilver: (to,amount)=>{
if(to==="player"){
this.playerSilver+=amount;
}
else{
to+=amount;
}
this.moneyConvert();
}
},
gold:{
addGold: (to,amount)=>{
if(to==="player"){
this.playerGold+=amount;
}
else{
to+=amount;
}
this.moneyConvert();
}
}
};
1 Answer
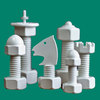
Steven Parker
231,007 Points
I got different results.
I tried calling: "obj.nestedObj.log2();
" and the console showed "it logged". So I wasn't able to replicate that error. But I agree, it's not due to hoisting, which only applies to functions and not variables.
But that's not a relative reference, which is what I believe you're looking for. For that, you might create a constructor function for the inner object that takes it's parent as an argument. Then you could call the constructor passing this
to it from the parent.