Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial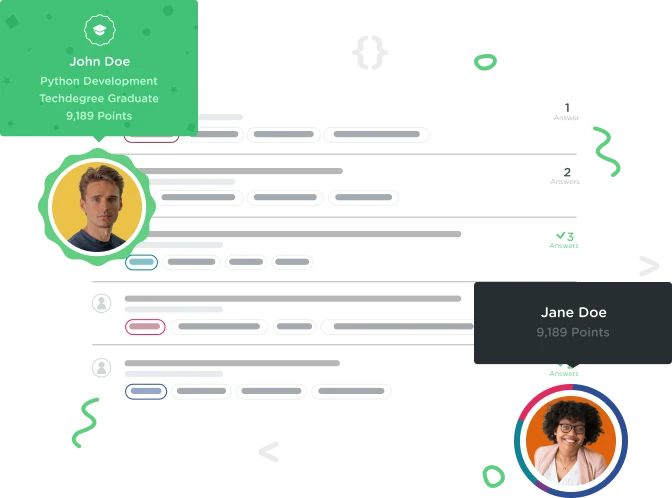

Jacob Brantley
124 PointsAccessing comments from the post_controller
You have shown how to retrieve and use post params from the comments controller. Is there a way to retrieve the params of comments (for multiple comments of an associated post) from the post controller?
4 Answers
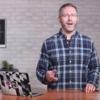
Jay McGavren
Treehouse TeacherAh, OK, so you're looking to work with the attributes of your model objects, not parameters from a form submission.
Your code is actually close to working. The problem is with the call to all?
:
@post.comments.all? {|booleanAttribute| true?}
The value passed to the block is not an attribute of the comment, it's the Comment
object itself. (Each Comment
in @post.comments
gets passed to the block, one at a time.) So you need to do something like this:
@post.comments.all? {|comment| comment.boolean_attribute}
...Where boolean_attribute
is a column you've set up in the comments
database table. (It could also be any method on a Comment
that returns true or false, but I'm guessing you want a table column.)
all?
will return true only if the block returns true for all members of the collection. So if boolean_attribute
returns true for all comments on the post, this call to all?
will return true.
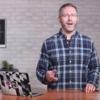
Jay McGavren
Treehouse TeacherTo make sure we're using the same terms:
Params: data from form fields submitted as part of a POST or PUT request.
Attributes: instance variables belonging to a model object, usually accessed through methods on that object.
You can definitely use parameters for a comment via the posts controller, or vice-versa. Just add additional fields to your form, and give them whatever names and ids you'd like. You'll then be able to access the submitted fields via the params
value within the controller's methods. You can take that form data, and use it to set attributes on any model object you'd like. You can look up or create Post
instances from the CommentsController
, or Comment
instances from the PostsController
; there's no limitations.

Jacob Brantley
124 PointsJay, thank you for the response and the clarification. Please let me elaborate to better describe the intent of my question.
I used the term params based on this from your code:
class CommentsController < ApplicationController
# No `new` action because form is provided by PostsController#show
def create
@post = Post.find(params[:post_id])
My understanding of the above: From the comments controller (which belongs_to the post object) you are retrieving the post_id attribute from the post object. I would like to know how to do something similar. From within the post controller (which has_many comment objects), retrieve a comment attribute from the comment object. Specifically I'd like to search through boolean attributes of all comments associated with a specific post and check if all results are True. Below is an example of something I tried that didn't work:
class CommentsController < ApplicationController
def findAllTrue
@post = Post.find(params[:id])
if @post.comments.all? {|booleanAttribute| true?}
puts "All True"
else
puts "Found False"
end
end
I would very much appreciate the help to round out my understanding of the module.

Jacob Brantley
124 PointsJay,
Thank you so much for the help! Still struggling with this for some reason.
I tried this:
Attempt:
def findAllTrue
@post = Post.find(params[:id])
if @post.comments.all? {|comment| comment.boolean_attribute?}
puts "All True"
else
puts "Found False"
end
end
And received this error: NoMethodError: undefined method `comments' for nil:NilClass

Jacob Brantley
124 PointsI actually had a typo. That solved it. Thanks!!