Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial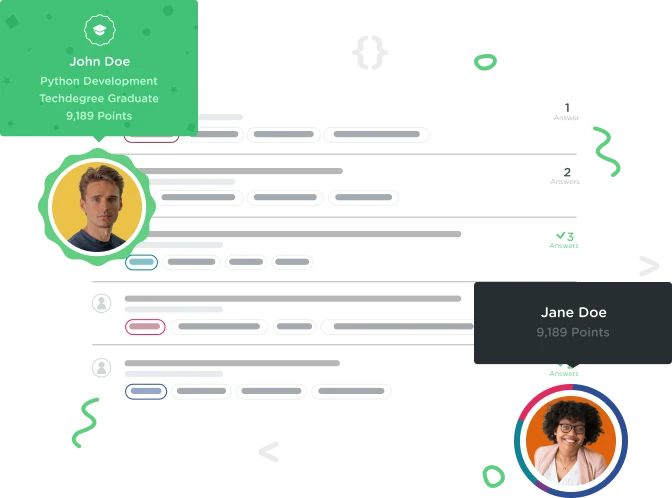

Lana Toogood
10,158 PointsAccessing Firestore document data with JavaScript
Hi, I've written some code that creates a new Firestore document from user input, then takes that Firestore doc, parses it into a JS object and adds it to an array called 'stock' on a stock object. When Firestore adds a new doc, it automatically generates a doc id accessed via doc.data.id. In the code I've written below, I'm not sure how to access the newly created Firestore doc in order to get its id, since the doc is created dynamically. All of the other parts of my code seem to work perfectly. Thanks!
const addItemsForm = document.querySelector("#addItemsForm");
const firestoreStock = db.collection('stock');
/**
* Listens for submit on `#addItemsForm`;
* Adds new document to Firestore with form input data;
* Calls addItemToStock() on stock object using Firestore document data.
*/
addItemsForm.addEventListener('submit', (e) => {
e.preventDefault();
var itemNo = addItemsForm.itemNo.value;
var storageRef = storage.ref('stockImages/' + itemNo + '.jpg');
storageRef.getDownloadURL().then(function(url){
firestoreStock.add({
name: addItemsForm.name.value,
description: addItemsForm.description.value,
itemNo: addItemsForm.itemNo.value,
size: addItemsForm.size.value,
price: addItemsForm.price.value,
imageURL: url
}).then(function() {
console.log('New doc successfully added to Firestore');
clearForm();
window.location.reload();
}).then(function(){
firestoreStock./*doc data id of doc just added to Firestore*/.get().then(function(doc){
if (doc.exists) {
stock.addItemToStock(doc);
console.log('new item object successfully added to stock array');
} else {
console.log('error with storageRef - document does not exist')
};
});
}).catch(function(error) {
console.log('Error getting document:', error);
});
})
})