Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial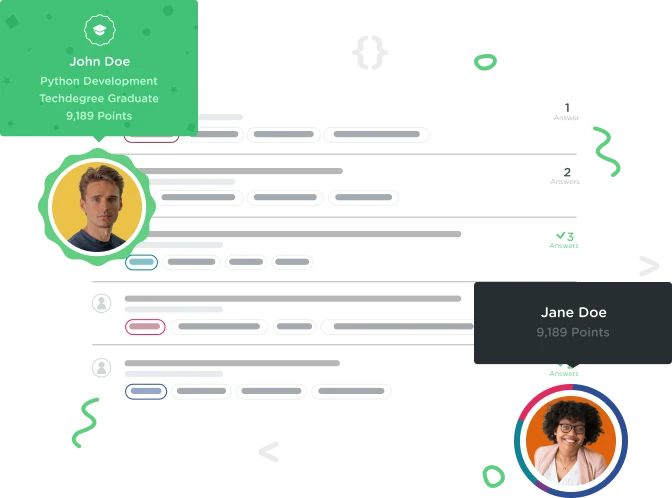

Michael Lambert
6,286 PointsAccessing global scopes
Hia,
I was happy with the below code that i put together to practice everything i've learned so far but i just watched the video that states accessing global variables from within a function is bad practice. I guess I could just pass the question number to the function as a 3rd argument but how could I have a running count on the number of correct answers within the function to use as the return value? If I initialised the variable within the function for keeping track of the number of correct answers then each time the function is called it would reset the count.
var correct = 0;
var questionCount = 0;
function questions(question, answer) {
questionCount ++; // accesses a global variable
var response = prompt(question).toLowerCase();
if(response === answer) {
q = "Correct";
correct ++; // accesses a global variable
} else {
q = "Wrong";
}
document.write('<p><strong>Question ' + questionCount + '</strong><br>' + question + ' ' + '<span>' + q + '</span><br><span class="red">' + response + '</span>' + '</p>')
}
questions('What is the capital of England?', 'london');
questions('If cats are feline, what are sheep?', 'ovine');
questions('For which fruit is the US state of Georgia famous?', 'peach');
questions('What is the third major Balearic Island with Majorca and Minorca?', 'ibiza');
questions('What is infant whale commonly called?', 'calf');
if (correct === 5) {
var prize = 'GOLD';
} else if (correct >= 3) {
prize = 'SILVER';
} else if (correct >= 1) {
prize = 'BRONZE';
} else {
prize = 'looser';
}
document.write('<p>You got ' + correct + ' correct answers and won the ' + prize.toUpperCase() + ' award</p>');
4 Answers

Leandro Botella Penalva
17,618 PointsHi Michael,
In this case it's not a bad idea at all to use a global variable inside a function because you only change its value within the function and not outside. If you plan to reuse the questions function but without the counters at some point then you should avoid the global variables. To do so, you could just increase the counter manually after each call of the questions function and return true or false whether the answer is correct or not.
This is an example:
var correct = 0;
var questionCount = 0;
function questions(question, answer) {
var response = prompt(question).toLowerCase();
if(response === answer) {
q = "Correct";
} else {
q = "Wrong";
}
document.write('<p><strong>Question ' + questionCount + '</strong><br>' + question + ' ' + '<span>' + q + '</span><br><span class="red">' + response + '</span>' + '</p>');
return response === answer;
}
if (questions('What is the capital of England?', 'london')) {
correct++;
}
questionCount++;
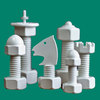
Steven Parker
231,269 PointsI'd take the advice against accessing globals "with a grain of salt".
The larger the program, the more important the concept is, but for a small program, careful use of globals can reduce program complexity and development time.
Here you could wrap you variables in a "game data" object, and pass a reference to that object to the function, but the code would not be as clear as what you have already.

Michael Lambert
6,286 PointsHi Steven,
Thanks for responding. I was kinda thinking that any other solution would be more complex than the way I originally did this so I was hoping that in some cases like this, that it is OK to use.
I'm a little unsure what you mean by wrapping variables in a "game data object, and pass a reference to that object to the function". I'm trying to visualise what that might look like and if it is something I will of covered in the first JavaScript course which I’ve just finished from within the Front End Development Track?
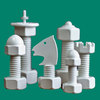
Steven Parker
231,269 PointsIt would be covered in courses about Object Oriented development or Module Patterns.

Michael Lambert
6,286 PointsThanks Steve. I'm not too far from starting the next JavaScript course 'JavaScript Loops, Arrays and Objects' just a couple of CSS / HTML courses before it so it might touch on the subject. If not then the next one is towards the end of the track 'Object-Oriented JavaScript' which should definitely cover it so I can come back to this and make the changes you mentioned.
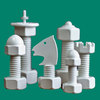
Steven Parker
231,269 PointsJust to be clear, I wasn't suggesting that as a program improvement, just as one way to eliminate referencing globals from within the function.

Michael Lambert
6,286 PointsFor sake of practice, I keep all programs from the courses then later use them again to make changes when I've advanced my knowledge. I realised from your below comments that how i currently have it coded is ok due to it's size and simplicity.
careful use of globals can reduce program complexity and development time.
the code would not be as clear as what you have already
I did take the instructor a bit too literally regarding the "bad practice" comment, so thanks for double checking I understood correctly :)

Jeff Wong
10,166 PointsUsing global variables is generally bad practice and should be avoided. In your case, there's one way to solve the problem of needing to access to global variables - use function closures. I am pretty sure the topic will be covered in the later part of the course and it is a powerful technique to eliminate the need to access global variables by a function.
Michael Lambert
6,286 PointsMichael Lambert
6,286 PointsHia Leandro,
Thanks for the feedback. I'm glad that in this particular case accessing globals from within functions is ok. I guess I took what the instructor said a bit too literally.
Your use of calling the
questions
function from within theif
statement and returning a boolean has elevated my thinking. I don't know why, but it never occurred to me to do the same, which is weird because I know I have used built-in functions within anif
statement before, so I should have known it was an option.I have just created a 2nd version of this using the method you provided for practice and all works well.
I'm not sure I fully understand what you mean with your above comment. Were you meaning that by not using global variables makes it less hassle to re-use the function in other code without messing around with creating the global variables that it depends on just to get it to work?
Leandro Botella Penalva
17,618 PointsLeandro Botella Penalva
17,618 PointsYes, basically using global variables in a function makes you create those variables when reusing the same function in another script, also, be careful using global variables inside and outside the function at the same time because you may lose values from the variable as its value is changed in several places. That's why is usually avoided using them. In your case the variable value was changed inside the function but not outside so the value never gets overwritten outside the function.
Michael Lambert
6,286 PointsMichael Lambert
6,286 PointsHi Leandro,
thanks for confirming and clarifying :)