Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial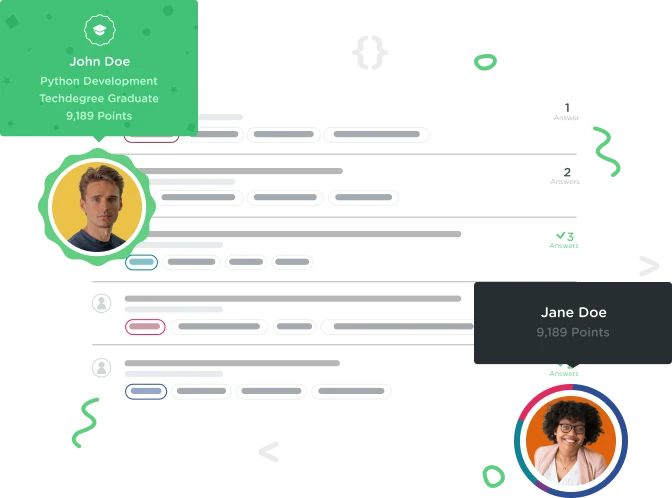
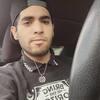
Joseph Escobedo
2,146 PointsAccessing props in React
As im doing the ReactJs track and on the React By Example part, ive noticed that instead of using this.props.guest.name, we are just using guests.name. I thought you had to refer back to the props in order to connect with the state and its properties? Also in the functional component of Guests and GuestList, its const Guest = props => and then the JSX. Ive always seen it like const Guests = props => { return () } or just a return statement. How are we able to return jsx without the statement?
1 Answer
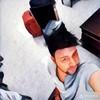
Ari Misha
19,323 PointsHello there! React and JavaScript has evolved a lot. Not many people realize that JavaScript has implicit returns. And JS takes this inspiration from Ruby syntax. Here are a couple of examples of implicit returns:
import React, { Component } from 'react';
class Person implements Component{
constructor (props) {
super(props);
}
render() {
const { name, dob } = this.props; // destructing props
return <SomeComponent name={name} ;
}
}
const Human = props => <Person name={props.name} dob={props.dob} />; // implicit return
OR
const Human = props => {
const { name, dob } = props; // don't need 'this' context in functional components
return <Person name={name} dob={dob} />; // explicit return but curly braces needed
}
OR
const Human = ({ name, dob }) => <Person name={name} dob={dob} />; // implicit return and destructuring props
OR
const Human = ({ name, dob }) => (<Person name={name} dob={dob} />); // still implicit return
OR
const someClass = ({ specificProps, ...rest }) => ({ someMap: () => <SomeOtherClass specificProps={specificProps} rest={rest} /> }); // implicit return with an Object
I hope it helped!
~ Ari