Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial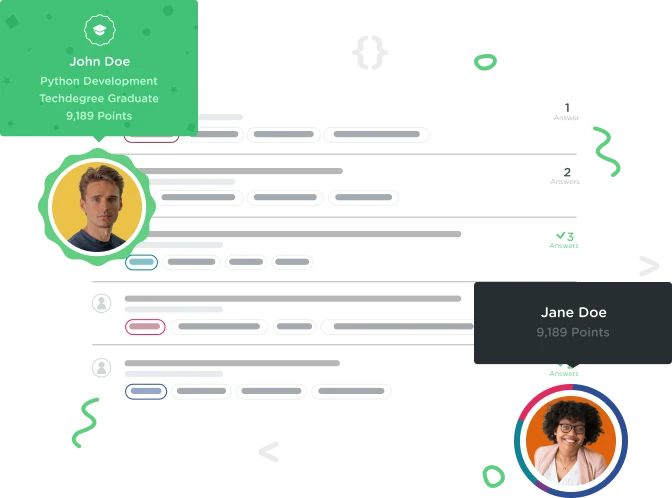
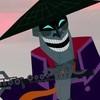
John Paige
7,436 PointsAccessor Method Challenge
Frog.cs(10,19): error CS1014: A get or set accessor expected
Compilation failed: 1 error(s), 0 warnings
This is the error message I'm getting. I'd appreciate some insight.
namespace Treehouse.CodeChallenges
{
class Frog
{
private int _numFliesEaten;
public int NumFliesEaten
{
public GetNumFliesEaten()
{
return _numFlieEaten;
}
public void SetNumFliesEaten(NumFliesEaten value)
{
_numFliesEaten = value;
}
}
}
2 Answers
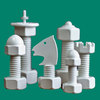
Steven Parker
229,788 Points
I spotted 5 issues:
- on line 8 you appear to still have the original "NumFliesEaten" definition (but without the ";")
- on line 9 you have an open brace starting a unneeded block that is never closed
- on line 10 you forgot to indicate the return type of GetNumFliesEaten()
- on line 12 you spelled "_numFlieEaten" without an "s"
- on line 15 you used "NumFliesEaten" as the type of "value" (which should probably be int)

mattmilanowski
15,323 PointsFirst count your brackets { } I think you have an extra } close bracket. After this I believe it will be expecting you to have the words 'get' and 'set' in place of existing methods like 'SetNumOfFliesEaten'.
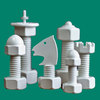
Steven Parker
229,788 Points
This challenge is about accessor methods for fields.
The keywords "get" and "set" refer to properties which will be discussed in a later video.
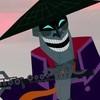
John Paige
7,436 PointsI went ahead and double checked my brackets, then swapped the "GetNumFliesEaten" and "SetNumFliesEaten" terms for "get" and "set". I actually corrected the brackets prior to posting my code because the "unexpected symbol: }" error popped.
namespace Treehouse.CodeChallenges
{
class Frog
{
private int _numFliesEaten;
public int NumFliesEaten
{
get
{
return _numFlieEaten;
}
set
{
_numFliesEaten = value;
}
}
}
//On to the get and set methods, after i changed the methods to
// simply "get" and "set", it came up with this exception:
Frog.cs(22,246): error CS1525: Unexpected symbol `end-of-file'
Compilation failed: 1 error(s), 0 warnings
Then i added a '}'
namespace Treehouse.CodeChallenges
{
class Frog
{
private int _numFliesEaten;
public int NumFliesEaten
{
get
{
return _numFlieEaten;
}
set
{
_numFliesEaten = value;
}
}
}
}
//Now this exception:
Frog.cs(12,17): error CS0103: The name `_numFlieEaten' does not exist in the current context
Compilation failed: 1 error(s), 0 warnings
John Paige
7,436 PointsJohn Paige
7,436 PointsThanks. Here is my revised code. Unfortunately, It still isn't passing.
Steven Parker
229,788 PointsSteven Parker
229,788 PointsYou're almost there.
In task 1, you were supposed to change NumFliesEaten into a private field. You created the private field, but you forgot to remove the public one.
On line 15 you added "int", but you forgot to remove "NumFliesEaten".
John Paige
7,436 PointsJohn Paige
7,436 PointsThanks. My final revision passed the challenge.