Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial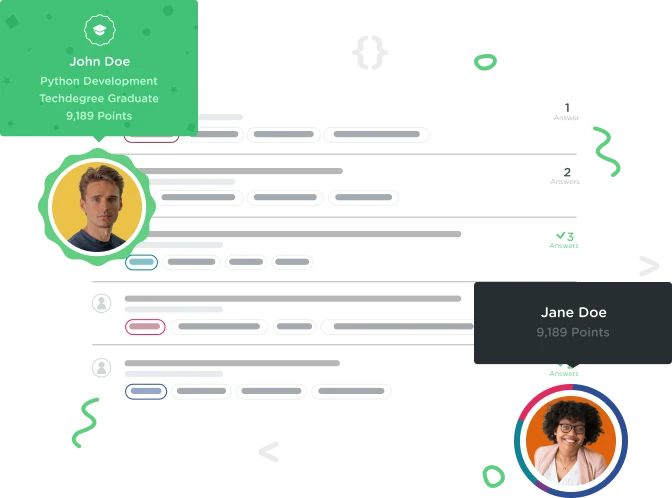

Tuff Kay
1,506 PointsAccounting for other potential user errors:
I ran into a few other potential user errors and I did my best to account for them. Was there a better way to handle either of these?
User Error #1: The user enters a dollar sign ($) as a part of their answer to question 1. In this case, the float function is unable to coerce the string and an error is returned.
I solved this by adding some additional code:
total_due = (input("What is the total? "))
total_due = float(total_due.replace('$', '')
total_due = float(total_due)
Essentially I told the script to replace any dollar signs with an empty character, removing them, and then I converted this new dollar-sign-less value to a float. I could combine them all into one line but I thought it looked cleaner and clearer this way.
User Error #2: The user answers question #2 (how many people?) as a string. The way the code is written by the end of the video, "too much" will display this error message back to us:
(could not convert string to float: 'too much')
Which might be confusing for the user. Even more confusing is if we say something like "20 people":
(invalid literal for int() with base 10: '20 people')
My solution to this was to establish a new variable (show_err) at the top of my code as False by default:
import math
show_err = False
Then I branched the Value Error exception code:
except ValueError as err:
print("Oh no! That's not a valid value. Try again...")
if show_err == True:
print("({})".format(err))
else:
print("(Please type value as a number.)")
Now we retain the original messaging for if a number less than or equal to 1 is entered, and in all other cases we are told to type the value as a number. (I used number as a colloquial instead of integer but there is still probably a better way to phrase this.)
I can't think of any other ways you could format your input for question 2 incorrectly so I believe this works. This was all the result of trial and error and my own research so please let me know if there is a more elegant solution!
1 Answer
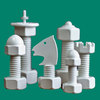
Steven Parker
230,274 PointsWhen does "show_err" get set to True?
Also, you never need to compare a boolean value to "True", you can simply name it:
if show_err: