Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial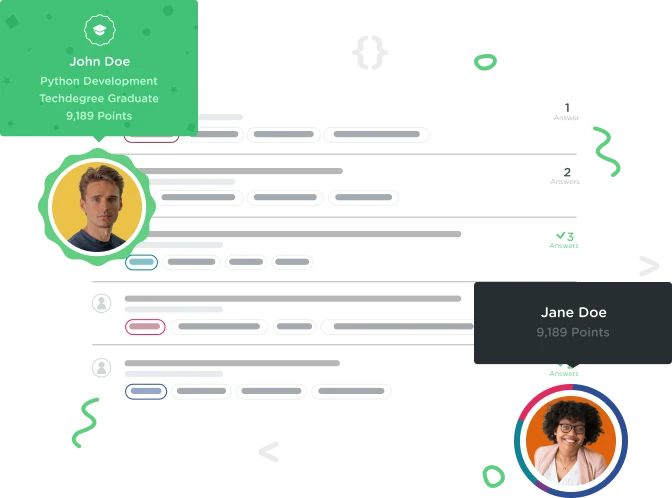

Marcio Porto
6,834 PointsAction Bar not showing
The action bar is not showing on my app when I run it. Maybe this has to do with the fact that I am using a more recent SDK and Android Studio. Anyway, does anyone know what I should do? Here is my code:
public class EditFriendsActivity extends ListActivity {
public static final String TAG = EditFriendsActivity.class.getSimpleName();
protected List<ParseUser> mUsers;
protected ParseRelation<ParseUser> mFriendsRelation;
protected ParseUser mCurrentUser;
@Override
protected void onCreate(Bundle savedInstanceState) {
// Set loading animation (progress bar)
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_edit_friends);
getListView().setChoiceMode(ListView.CHOICE_MODE_MULTIPLE);
}
@Override
protected void onResume() {
super.onResume();
mCurrentUser = ParseUser.getCurrentUser();
mFriendsRelation = mCurrentUser.getRelation(ParseConstants.KEY_FRIENDS_RELATION);
// Show set progress bar
ParseQuery<ParseUser> query = ParseUser.getQuery();
query.orderByAscending(ParseConstants.KEY_USERNAME);
// Add search feature later. Look at the Parse documentation regarding this section
query.setLimit(1000);
query.findInBackground(new FindCallback<ParseUser>() {
@Override
public void done(List<ParseUser> users, ParseException e) {
// Hide the progress bar
if (e == null) {
// Success
mUsers = users;
String[] usernames = new String[mUsers.size()];
int i = 0;
for (ParseUser user : mUsers) {
usernames[i] = user.getUsername();
i++;
}
ArrayAdapter<String> adapter = new ArrayAdapter<String>(
EditFriendsActivity.this,
android.R.layout.simple_list_item_checked,
usernames);
setListAdapter(adapter);
addFriendCheckmarks();
} else {
Log.e(TAG, e.getMessage());
AlertDialog.Builder builder = new AlertDialog.Builder(EditFriendsActivity.this);
builder.setMessage(e.getMessage())
.setTitle(R.string.error_title)
.setPositiveButton(android.R.string.ok, null);
AlertDialog dialog = builder.create();
dialog.show();
}
}
});
}
private void addFriendCheckmarks() {
mFriendsRelation.getQuery().findInBackground(new FindCallback<ParseUser>() {
@Override
public void done(List<ParseUser> friends, ParseException e) {
if (e == null) {
// list returned. Look for a match
for (int i = 0; i < mUsers.size(); i++) {
ParseUser user = mUsers.get(i);
for (ParseUser friend : friends) {
if (friend.getObjectId().equals(user.getObjectId())) {
getListView().setItemChecked(i, true);
}
}
}
} else {
Log.e(TAG, e.getMessage());
}
}
});
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
// Handle action bar item clicks here. The action bar will
// automatically handle clicks on the Home/Up button, so long
// as you specify a parent activity in AndroidManifest.xml.
int id = item.getItemId();
//noinspection SimplifiableIfStatement
if (id == R.id.action_settings) {
return true;
}
return super.onOptionsItemSelected(item);
}
@Override
protected void onListItemClick(ListView l, View v, int position, long id) {
super.onListItemClick(l, v, position, id);
if (getListView().isItemChecked(position)) {
// add friend
mFriendsRelation.add(mUsers.get(position));
} else {
// remove friend
mFriendsRelation.remove(mUsers.get(position));
}
// Saves local changes made above on the back-end
mCurrentUser.saveInBackground(new SaveCallback() {
@Override
public void done(ParseException e) {
if (e != null) {
Log.e(TAG, e.getMessage());
}
}
});
}
}