Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial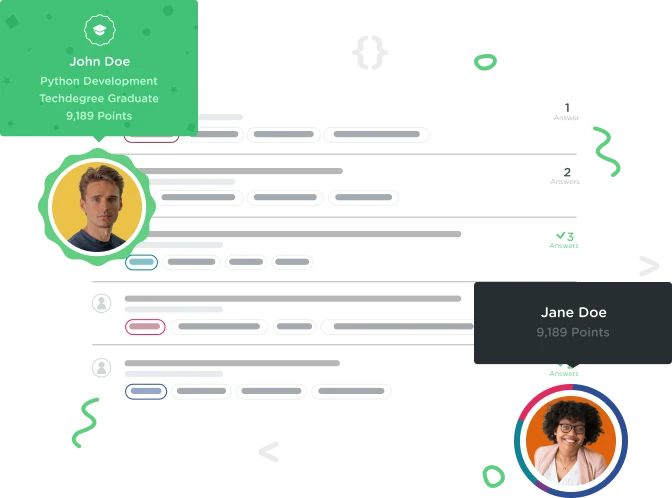

Jay Fleming
10,353 PointsActionView: MissingTemplate Question
Hello, my test is passing until I add in the "is unsuccessful with not enough content" test. At that point, I get this error:
Failure/Error: click_button "Save" ActionView: MissingTemplate;
How could the template be missing if the "is successful with valid content" passes?
require 'spec_helper'
describe "Editing todo items" do
let!(:todo_list) { TodoList.create(title: "Grocery list", description: "Groceries") }
let!(:todo_item) { todo_list.todo_items.create(content: "Milk" ) }
def visit_todo_list(list)
visit "/todo_lists"
within "#todo_list_#{todo_list.id}" do
click_link "List Items"
end
end
it "is successful with valid content" do
visit_todo_list(todo_list)
within("#todo_item_#{todo_item.id}") do
click_link "Edit"
end
fill_in "Content", with: "Lots of Milk"
click_button "Save"
expect(page).to have_content("Saved todo list item")
todo_item.reload
expect(todo_item.content).to eq("Lots of Milk")
end
it "is unsuccessful with not enough content" do
visit_todo_list(todo_list)
within("#todo_item_#{todo_item.id}") do
click_link "Edit"
end
fill_in "Content", with: "1"
click_button "Save"
expect(page).to_not have_content("Saved todo list item")
expect(page).to have_content("Content is too short ")
todo_item.reload
expect(todo_item.content).to eq("Milk")
end
end
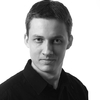
Maciej Czuchnowski
36,441 PointsOh, please paste your controller here.
5 Answers
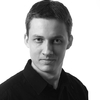
Maciej Czuchnowski
36,441 PointsOK, here's your answer, in this line:
render action: edit
You need to have a : before the edit, like this :edit

Jay Fleming
10,353 PointsThanks, Maciej. Sorry about that:
Error:
1) Editing todo items is unsuccessful with not enough content
Failure/Error: click_button "Save"
ActionView::MissingTemplate:
Missing template todo_items/#<TodoItem:0xbaabff58>, application/#<TodoItem:0xbaabff58> with {:locale=>[:en], :formats=>[
:html], :handlers=>[:erb, :builder, :raw, :ruby, :jbuilder, :coffee]}. Searched in:
* "/home/treehouse/projects/odot/app/views"
# ./app/controllers/todo_items_controller.rb:36:in `update'
# ./spec/features/todo_items/edit_spec.rb:32:in `block (2 levels) in <top (required)>'
Controller:
class TodoItemsController < ApplicationController
def index
@todo_list = TodoList.find(params[:todo_list_id])
end
def new
@todo_list = TodoList.find(params[:todo_list_id])
@todo_item = @todo_list.todo_items.new
end
def create
@todo_list = TodoList.find(params[:todo_list_id])
@todo_item = @todo_list.todo_items.new(todo_item_params)
if @todo_item.save
flash[:sucess] = "Added todo list item."
redirect_to todo_list_todo_items_path
else
flash[:error] = "There was a problem adding that todo list item."
render action: :new
end
end
def edit
@todo_list = TodoList.find(params[:todo_list_id])
@todo_item = @todo_list.todo_items.find(params[:id])
end
def update
@todo_list = TodoList.find(params[:todo_list_id])
@todo_item = @todo_list.todo_items.find(params[:id])
if @todo_item.update_attributes(todo_item_params)
flash[:success] = "Saved todo list item"
redirect_to todo_list_todo_items_path
else
flash[:error] = "That todo item could not be saved"
render action: edit
end
end
def url_options
{ todo_list_id: params[:todo_list_id] }.merge(super)
end
private
def todo_item_params
params[:todo_item].permit(:content)
end
end

Jay Fleming
10,353 PointsThanks a ton, Maciej! That was driving me insane.

Jay Fleming
10,353 PointsMaciej, another question for you if you are still around. Why would that error have only popped up with multiple unit tests? I did't have that issue with just the "is successful with valid content" test.
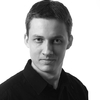
Maciej Czuchnowski
36,441 PointsBecause this line was in the else
clause, which only fired when the content was invalid. With valid content, you got
flash[:success] = "Saved todo list item"
redirect_to todo_list_todo_items_path
and it did not reach the improper line :)

Jay Fleming
10,353 Pointsahhh yes! That makes perfect sense. Thanks a ton!
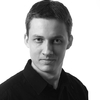
Maciej Czuchnowski
36,441 PointsYou're welcome :)
Maciej Czuchnowski
36,441 PointsMaciej Czuchnowski
36,441 PointsPlease paste the whole failure, it will tell us which line of the spec this applies to.