Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial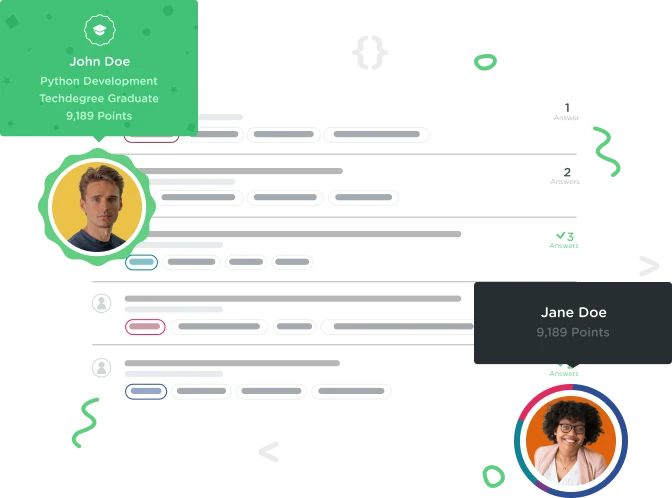
Guy Claude Ngbwa
1,593 Pointsactivity_main cannot be resolved or is not a field
i keep getting this error " activity_main is not a field" . what am I doing wrong?
package com.example.crystalball;
import android.app.Activity;
import android.os.Bundle;
import android.view.Menu;
import android.view.View;
import android.widget.Button;
import android.widget.TextView;
public class MainActivity extends Activity {
private CrystalBall mCrystalBall = new CrystalBall();
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// Declare our View variables and assign them the Views from the layout file
final TextView answerLabel = (TextView) findViewById(R.id.textView1);
Button getAnswerButton = (Button) findViewById(R.id.button1);
getAnswerButton.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
String answer = mCrystalBall.getAnAnswer();
// Update the label with our dynamic answer
answerLabel.setText(answer);
}
});
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
getMenuInflater().inflate(R.menu.activity_main, menu);
return true;
}
}
15 Answers

Mark Melin
4,239 PointsWhat does your manifest file look like? Check to see if the source is something along the lines like com.example.MainActivity. I'm building an android game for school (I get the same error sometimes too.) Sometimes I tend to think android is still a relatively young OS just like the ADT is, sometimes the errors don't make sense when they're perfectly fine.

Ben Jakuben
Treehouse TeacherR.layout.main refers to a layout XML file in your "res/layout" directory. It's not straightforward without and explanation, though. :)
So in an Android project, all your resources like images and layouts are stored in the "res" directory (short for "resources"). When your project is built in Eclipse, a special Java class called the R class is generated. It's a simple class that maps all those files in your "res" folder. Then you can reference them in code easily by using the R class. So R.layout refers to your layout folder, R.drawable refers to drawable items like images, etc.
It sounds like your file was simply named differently when you first created the project, so instead of R.layout.activity_main you had to use R.layout.main.
Glad you got it working!
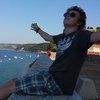
Ziga Klemencic
2,569 Pointsi have the same problem but can't resolve it. package com.zigax.crystalball2;
import android.R; import android.app.Activity; import android.os.Bundle; import android.view.Menu; import android.view.View; import android.widget.Button; import android.widget.TextView;
public class MainActivity extends Activity {
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_list_item);
//Declare our view variables
final TextView anwserLabel = (TextView) findViewById(R.id.text1);
Button getAnwserButton = (Button) findViewById(R.id.button1);
getAnwserButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View arg0) {
String anwser = "Yes";
anwserLabel.setText(anwser);
}
});
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.main, menu);
return true;
}
tried to do whole project again but i cant make it work.
Please help me. Thanks

Ben Jakuben
Treehouse TeacherYour 'setContentView()' line is looking for R.layout.activity_list_item
. Is that file (activity_list_item.xml) in your res/layout directory? That normally should be "activity_main" for the Crystal Ball project.
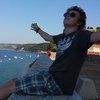
Ziga Klemencic
2,569 Pointsno, in res/layout directory i have activity_main.xml. But if i set R.layout.activity_list_item. to R.layout.activity_main its error again and i fix it and it puts in activity_list_item.
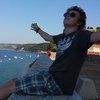
Ziga Klemencic
2,569 Pointsactivity_main cannot be resolved or is not a field and then it says : 1 quick fix avaliable : change to activiti_list_item.

Ben Jakuben
Treehouse TeacherInteresting. Can you try changing the line to this:
setContentView(R.layout.activity_main);
And then clean your project with Project > Clean (from the Eclipse menu bar at the top). It sounds like there is an issue with your R.java file, which is generated when you build the project. It can happen pretty easily. If the clean doesn't fix it then we'll have to look at the files in your res directory. (The R.java file is built from everything in the res directory.)
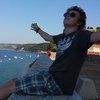
Ziga Klemencic
2,569 PointssetContentView(R.layout.activity_main);----> i can fix that to activiti_list_item but i cant fix : getMenuInflater().inflate(R.menu.activity_main, menu);-----> cant fix it to anything just says:rename in file.
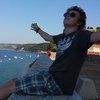
Ziga Klemencic
2,569 Pointsno i didn't fix it. D:
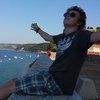
Ziga Klemencic
2,569 Pointsnow i got 2 errors:
- setContentView(R.layout.activity_main); 2.getMenuInflater().inflate(R.menu.activity_main, menu);

Ben Jakuben
Treehouse TeacherOkay, this should be closer, though. The menu one (R.menu) corresponds to the folder res/menu. What files do you have in there? I'm guessing you have menu.xml, in which case you need to revert that line to R.menu.main. That is the file that's generated by Eclipse when you start a new project. It's used for the menu in the Action Bar (which we don't use in the project).
So change that, and then paste in your XML code for activity_main.xml. It seems like there might be an error there.
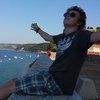
Ziga Klemencic
2,569 Pointsunder problems--->type it says Java Problem, for both of them.
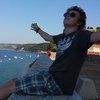
Ziga Klemencic
2,569 Pointsres/menu---> file main.xml

Ben Jakuben
Treehouse TeacherOkay, good. So change that back (getMenuInflater().inflate(R.menu.main, menu);
) and then paste in your XML code for activity_main.xml and we can see if there are any errors there.
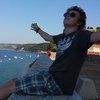
Ziga Klemencic
2,569 Pointsok changed to getMenuInflater().inflate(R.menu.main, menu);
i'm sorry i dont understand what to do next?and then paste in your XML code for activity_main.xml

Ben Jakuben
Treehouse TeacherI meant to paste it in a reply here. :) You can format it as code by adding three backticks (`) on new lines before and after the code. But don't worry too much about that - I'll format it as long as you paste the code in. Just select all the text in activity_main.xml and paste it in a reply here.
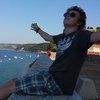
Ziga Klemencic
2,569 Points<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingBottom="@dimen/activity_vertical_margin"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
tools:context=".MainActivity" >
<Button
android:id="@+id/button1"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_centerHorizontal="true"
android:layout_centerVertical="true"
android:text="Enlighten me!" />
</RelativeLayout>

Ben Jakuben
Treehouse TeacherOkay, I think I see your error. Your XML file doesn't have a TextView with an ID of text1
. But in your Java file, you are trying to reference such a TextView with this line:
final TextView anwserLabel = (TextView) findViewById(R.id.text1);
Check out the part in the video where we add a TextView and once you have that in the layout you should be able to run your project. Make sure you either give the TextView the same ID as in your code (text1
) or update your code to match the ID from your layout file. Those IDs are how the two files work together.
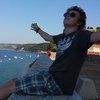
Ziga Klemencic
2,569 Pointspackage com.zigax.crystalball2;
import android.R;
import android.app.Activity;
import android.os.Bundle;
import android.view.Menu;
import android.view.View;
import android.widget.Button;
import android.widget.TextView;
public class MainActivity extends Activity {
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
//Declare our view variables
final TextView anwserLabel = (TextView) findViewById(R.id.text1);
Button getAnwserButton = (Button) findViewById(R.id.button1);
getAnwserButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View arg0) {
String anwser = "Yes";
anwserLabel.setText(anwser);
}
});
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.main, menu);
return true;
}
}
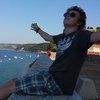
Ziga Klemencic
2,569 PointsI did it :D:D I'm so totaly happy right now :D hehe
Ben THANKS FOR ALL THE HELP AND TIME. YOU'RE THE MAN!!!!!

Ben Jakuben
Treehouse TeacherHooray! It can be so frustrating, but it's an awesome feeling when you get it, isn't it? Glad I could help.
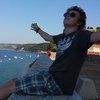
Ziga Klemencic
2,569 PointsYes, it was really annoying :D but now in past it :D:D Really gratefoul for your help and your respond time :D Thanks again :D
Guy Claude Ngbwa
1,593 PointsGuy Claude Ngbwa
1,593 PointsI did fix it, I do not how. I replaced "activity_main" by just "main" and it worked fine. Still do not understand what was the problem and how "main" just fixed it.