Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial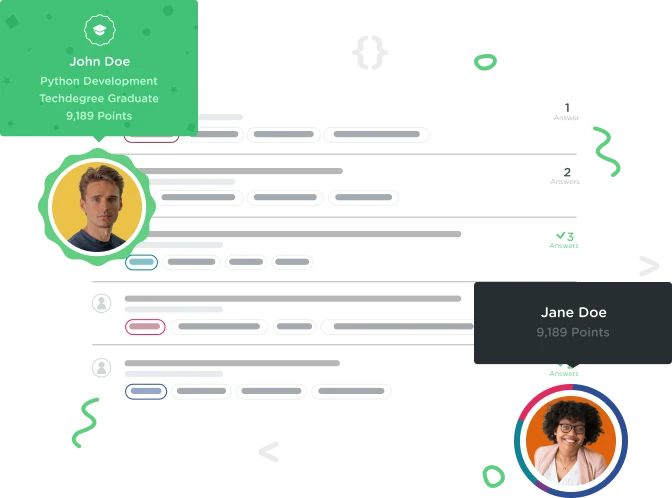

Eric Drake
6,339 PointsActivityMainBinding class not resolving in the Weather app project
I'm working on the "Plugging in the Data" section of the "Build a weather app" project. The project calls for using data binding to link data from an object to the TextViews that correspond to it. I get an error "cannot find symbol ActivityMainBinding" in my MainActivity.java file. My understanding is that this class was supposed to be auto-generated, but I guess I missed a step.
Here is my MainActivity.java file
package com.doublel.stormy;
import android.content.Context;
import android.net.ConnectivityManager;
import android.net.NetworkInfo;
import android.os.Bundle;
import android.text.method.LinkMovementMethod;
import android.util.Log;
import android.widget.TextView;
import android.widget.Toast;
import androidx.appcompat.app.AppCompatActivity;
import androidx.databinding.DataBindingUtil;
import org.jetbrains.annotations.NotNull;
import org.json.JSONException;
import org.json.JSONObject;
import java.io.IOException;
import okhttp3.Call;
import okhttp3.Callback;
import okhttp3.OkHttpClient;
import okhttp3.Request;
import okhttp3.Response;
public class MainActivity extends AppCompatActivity {
public static final String TAG = MainActivity.class.getSimpleName();
private CurrentWeather currentWeather;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
final ActivityMainBinding binding = DataBindingUtil.setContentView(MainActivity.this, R.layout.activity_main);
TextView darkSky = findViewById(R.id.darkSkyAttribution);
darkSky.setMovementMethod(LinkMovementMethod.getInstance());
double latitude = 42.52972;
double longitude = -113.799639;
String apiKey = "6d869eb5c52787b691e69ffeca85d3af";
String forecastURL = "https://api.darksky.net/forecast/" + apiKey + "/" + latitude + "," + longitude;
if(isNetworkAvailable()) {
OkHttpClient client = new OkHttpClient();
Request request = new Request.Builder().url(forecastURL).build();
Call call = client.newCall(request);
call.enqueue(new Callback() {
@Override
public void onFailure(@NotNull Call call, @NotNull IOException e) {
Log.e(TAG, "Response failed with error: ", e);
e.printStackTrace();
}
@Override
public void onResponse(@NotNull Call call, @NotNull Response response) throws IOException {
try {
String jsonData = response.body().string();
Log.v(TAG, jsonData);
if (response.isSuccessful()) {
currentWeather = getCurrentDetails(jsonData);
CurrentWeather displayWeather = new CurrentWeather(
currentWeather.getLocationLabel(),
currentWeather.getIcon(),
currentWeather.getTime(),
currentWeather.getTemperature(),
currentWeather.getHumidity(),
currentWeather.getPrecipChance(),
currentWeather.getSummary(),
currentWeather.getTimezone()
);
binding.setWeather(displayWeather);
} else {
alertUserAboutError();
}
} catch (IOException e) {
Log.e(TAG, "IO Exception caught: ", e);
} catch (JSONException e){
Log.e(TAG, "JSON Exception caught: ", e);
}
}
});
} else {
Toast.makeText(this, getString(R.string.network_unavailable_message), Toast.LENGTH_LONG).show();
}
Log.d(TAG, "Main UI code is running.");
}
private CurrentWeather getCurrentDetails(String jsonData) throws JSONException {
JSONObject forecast = new JSONObject(jsonData);
JSONObject currently = forecast.getJSONObject("currently");
CurrentWeather currentWeather = new CurrentWeather();
currentWeather.setHumidity(currently.getDouble("humidity"));
currentWeather.setTemperature(currently.getDouble("temperature"));
currentWeather.setPrecipChance(currently.getDouble("precipProbability"));
currentWeather.setLocationLabel("Burley, Idaho");
currentWeather.setSummary(currently.getString("summary"));
currentWeather.setTime(currently.getLong("time"));
currentWeather.setIcon(currently.getString("icon"));
currentWeather.setTimezone(forecast.getString("timezone"));
Log.d(TAG, "Current Time: " + currentWeather.getFormattedTime());
return currentWeather;
}
private boolean isNetworkAvailable() {
ConnectivityManager manager = (ConnectivityManager) getSystemService(Context.CONNECTIVITY_SERVICE);
NetworkInfo networkInfo = manager.getActiveNetworkInfo();
boolean isAvailable = false;
if(networkInfo != null && networkInfo.isConnected()){
isAvailable = true;
}
return isAvailable;
}
private void alertUserAboutError() {
AlertDialogFragment dialog = new AlertDialogFragment();
dialog.show(getFragmentManager(), "error_dialog");
}
}
Here is my content_main.xml file
<?xml version="1.0" encoding="utf-8"?>
<layout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools">
<data>
<variable name="weather" type="com.doublel.stormy.CurrentWeather" />
</data>
<androidx.constraintlayout.widget.ConstraintLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="@color/colorAppBackground"
tools:context=".MainActivity"
tools:showIn="@layout/activity_main">
<TextView
android:id="@+id/temperatureValue"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:fontFamily="sans-serif-black"
android:text="100"
android:textColor="@color/colorTextColor"
android:textSize="150sp"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<ImageView
android:id="@+id/degreeImageView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="20dp"
app:layout_constraintLeft_toRightOf="@id/temperatureValue"
app:layout_constraintTop_toTopOf="@id/temperatureValue"
app:srcCompat="@drawable/degree" />
<TextView
android:id="@+id/timeValue"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:fontFamily="sans-serif-black"
android:text="@string/label_default_time"
android:textColor="@color/colorHalfWhite"
android:textSize="30sp"
app:layout_constraintBottom_toTopOf="@id/temperatureValue"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent" />
<TextView
android:id="@+id/locationValue"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginBottom="24dp"
android:fontFamily="sans-serif-black"
android:text="@string/label_default_location"
android:textColor="@color/colorTextColor"
android:textSize="30sp"
app:layout_constraintBottom_toTopOf="@id/timeValue"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent" />
<ImageView
android:id="@+id/iconImageView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginBottom="32dp"
app:layout_constraintBottom_toTopOf="@id/locationValue"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent"
app:srcCompat="@drawable/cloudy_night"
tools:layout_editor_absoluteY="158dp" />
<androidx.constraintlayout.widget.Guideline
android:id="@+id/guideline3"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:orientation="vertical"
app:layout_constraintGuide_percent="0.33" />
<androidx.constraintlayout.widget.Guideline
android:id="@+id/guideline4"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:orientation="vertical"
app:layout_constraintGuide_percent="0.66" />
<TextView
android:id="@+id/humidityLabel"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="@string/label_humidity"
android:textColor="@color/colorTextColor"
android:textSize="24sp"
app:layout_constraintLeft_toLeftOf="@id/guideline3"
app:layout_constraintRight_toRightOf="@id/guideline3"
app:layout_constraintTop_toBottomOf="@id/temperatureValue" />
<TextView
android:id="@+id/humidityValue"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="0.88"
android:textAlignment="center"
android:textColor="@color/colorHalfWhite"
app:layout_constraintLeft_toLeftOf="@id/guideline3"
app:layout_constraintRight_toRightOf="@id/guideline3"
app:layout_constraintTop_toBottomOf="@id/humidityLabel" />
<TextView
android:id="@+id/precipitationLabel"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="@string/precip_label"
android:textColor="@color/colorTextColor"
android:textSize="24sp"
app:layout_constraintLeft_toLeftOf="@id/guideline4"
app:layout_constraintRight_toRightOf="@id/guideline4"
app:layout_constraintTop_toBottomOf="@id/temperatureValue" />
<TextView
android:id="@+id/precipitationValue"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="70%"
android:textAlignment="center"
android:textColor="@color/colorHalfWhite"
app:layout_constraintLeft_toLeftOf="@id/guideline4"
app:layout_constraintRight_toRightOf="@id/guideline4"
app:layout_constraintTop_toBottomOf="@id/precipitationLabel" />
<TextView
android:id="@+id/summaryValue"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="32dp"
android:textColor="@color/colorTextColor"
android:text="@{weather.summary}"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintTop_toBottomOf="@id/precipitationValue" />
<TextView
android:id="@+id/darkSkyAttribution"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginBottom="32dp"
android:text="@string/darksky_message"
android:textColor="@color/colorTextColor"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent" />
</androidx.constraintlayout.widget.ConstraintLayout>
</layout>
Please help my understand where I went wrong. Thanks.
2 Answers

Lauren Moineau
9,483 PointsHi Eric. The problem is that your XML file name is content_main.xml
but you call activity_main.xml
in your code.
You have 2 options:
- rename your XML file to
activity_main.xml
- In
onCreate()
, modify your generated binding class as such (usingcontent_main.xml
):
final ContentMainBinding binding = DataBindingUtil.setContentView(MainActivity.this,
R.layout.content_main);
That should fix it :)

Remy Benza
6,259 PointsTry rebuilding your project with 'Build -> Rebuild Project'. Your xml data tags are set correctly.

Eric Drake
6,339 PointsThanks for the suggestion. I tried it this morning, and the ActivityMainBinding class still doesn't resolve. Does this have something to do with my using the androidx support library instead of android.support?