Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial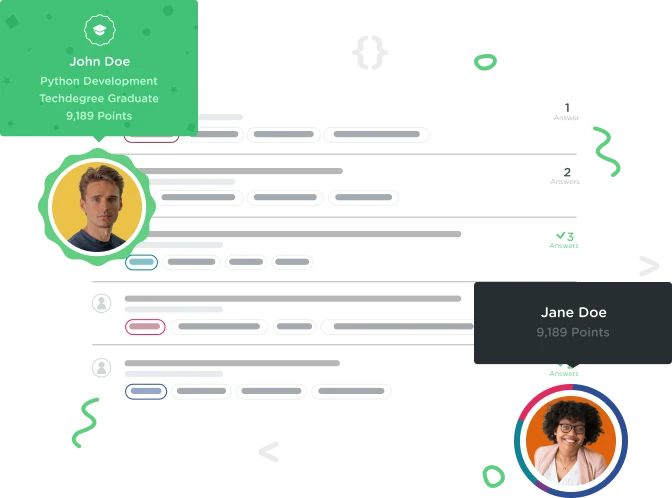

Colt Shelby
1,175 Points"actual and formal argument lists differ in length"
I'm messing around with objects and my program really does nothing right now but I'm having trouble setting up a constructor and getting the object to run. I'm trying to get this to just print the name that the user enters, but I get the error "actual and formal argument lists differ in length" I've googled it but I can't really find an answer. Thanks!
Beehive.java:
import java.util.Random;
import java.io.Console;
import java.util.Scanner;
public class Beehive {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("Your queen is establishing a nest!");
String name = scanner.nextLine();
Beehive beehive = new Beehive(name);
System.out.println(name);
}
}
Beehavior.java:
public class Beehavior{
// Declare variables
public int mBeesCount;
public int mBroodCount;
public int mAge;
public String mHiveName;
public void Beehive(String hiveName) {
mHiveName = hiveName;
}
}
1 Answer

Derek Markman
16,291 PointsTo make your code work correctly you need to first properly set up the constructor in your Beehavior class. Then, in your Beehive class you need to create the instance variable to your Beehavior class's constructor (Not the Beehive class's constructor itself).
Beehive.java
import java.util.Random;
import java.io.Console;
import java.util.Scanner;
public class Beehive {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("Your queen is establishing a nest!");
String name = scanner.nextLine();
Beehavior beehavior = new Beehavior(name); //invoking Beehavior's constructor
System.out.println(name);
System.out.println(beehavior.mHiveName); //this is an alternative, but prints out the same thing.
}
}
Beehavior.java
public class Beehavior {
// Declare variables
public int mBeesCount;
public int mBroodCount;
public int mAge;
public String mHiveName;
public Beehavior(String hiveName) { //Beehavior's constructor
mHiveName = hiveName;
}
}
Let me know if you have any other questions.