Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial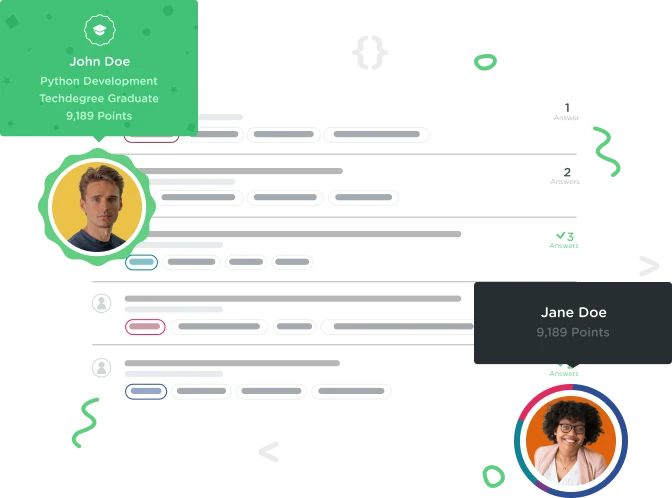
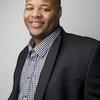
Sahmad Nakumbe
3,423 PointsAdd a final else clause to this conditional statement. If both the isAdmin and isStudent variables are false, the value
const isAdmin = false;
const isStudent = false;
let message;
if ( isAdmin ) {
message = 'Welcome admin';
} else if ( isStudent ) {
message = 'Welcome student';
} else ( isAdmin + isStudent === false ) {
message = 'Access denied';
}
10 Answers
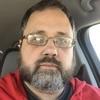
Mark Sebeck
Treehouse Moderator 37,776 PointsYou don’t need and can’t have a condition on the else. The else will run if the other 2 conditions are false. So just remove the condition and it should work.

Sonia Nitu
Front End Web Development Techdegree Student 10,852 PointsThis works perfectly in the browser, but not in the challenge. Finally, I have realized the final DOT after "Access denied" was misplaced INSIDE the quote marks, which made all the difference. Frustrating :(. `const isAdmin = false; const isStudent = false; let message;
if ( isAdmin ) { message = 'Welcome admin'; } else if ( isStudent ) { message = 'Welcome student'; } else { message = 'Access denied.'; }`
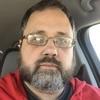
Mark Sebeck
Treehouse Moderator 37,776 PointsHi Sonia. Yes in challenges strings have to be exact or they won't pass. "Access denied" !== "Access denied."
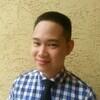
Tom Nguyen
33,500 PointsThis is what I used to pass:
const isAdmin = false;
const isStudent = false;
let message;
if ( isAdmin ) {
message = 'Welcome admin';
} else if ( isStudent ) {
message = 'Welcome student';
} else {
message = "Access denied";
}
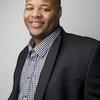
Sahmad Nakumbe
3,423 Pointscondition = ()
Thank you!
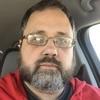
Mark Sebeck
Treehouse Moderator 37,776 PointsYou are welcome. Yes conditions are only for if and else if. Then the else will run if all conditions are false.
if (condition)
else if (condition)
else
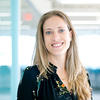
Shanna vick-Morris
6,683 Pointsconst isAdmin = false;
const isStudent = false;
let message;
if ( isAdmin ) {
message = 'Welcome admin';
} else if ( isStudent ) {
message = 'Welcome student';
}
{isAdmin + isStudent = false} {
message = 'Access denied';
}
I am getting and ERROR: "Did you add an 'else' clause to the end of a conditional statement"?
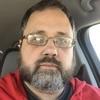
Mark Sebeck
Treehouse Moderator 37,776 PointsHi Shanna. You need to add an else to the end of the conditional statement. :). Hope that helps
...
} else
{
message = 'Access denied';
}

Khatia Bagaturia
2,050 PointsHi I am struggling about this exact tusk right now . looks like I am doing everything alright . can you explain my mistake ( isAdmin + isStudent === false ); } else { message = 'Access denied';
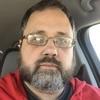
Mark Sebeck
Treehouse Moderator 37,776 PointsHi Khatia. So the challenge gives us:
const isAdmin = false;
const isStudent = false;
let message;
if ( isAdmin ) {
message = 'Welcome admin';
} else if ( isStudent ) {
message = 'Welcome student';
}
So I see what you are doing. It's a good try. You are making sure both conditions are false. But you can't conditions like that. The good news is you don't need to. If your code makes it to the else then all conditions above had to be false. So delete this part:
( isAdmin + isStudent === false );
and simply use your else. And don't forget to close your bracket at the end.
Hope this helps.

Khatia Bagaturia
2,050 Points( isAdmin + isStudent === false ); } else { message = 'Access denied'; }
is this what you mean ? or I do not need to write message = ' Access denied ' ;

Khatia Bagaturia
2,050 Pointsokay I deleted the one you told me and my code looks like this : } else message = 'Access denied'; } still does not run .

Khatia Bagaturia
2,050 PointsThat is how everything looks like right now when I am trying to finish it .
const isAdmin = false; const isStudent = false; let message;
if ( isAdmin ) { message = 'Welcome admin'; } else if ( isStudent ) { message = 'Welcome student'; } } else { message = 'Access denied'; }
and the result is can not read property 1 null
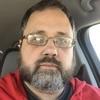
Mark Sebeck
Treehouse Moderator 37,776 PointsYou have an extra close bracket before the else. Delete that and it should work.
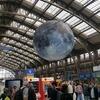
Amadou Sow
6,082 Pointsconst isAdmin = false; const isStudent = false; let message;
if ( isAdmin ) { message = 'Welcome admin'; } else if ( isStudent ) { message = 'Welcome student'; } else {message = 'Access denied'; }
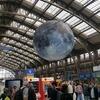
Amadou Sow
6,082 PointsThat's the correct answer.
Sahmad Nakumbe
3,423 PointsSahmad Nakumbe
3,423 Pointsconst isAdmin = false; const isStudent = false; let message;
if ( isAdmin ) { message = 'Welcome admin'; } else if( isStudent ) { message = 'Welcome student'; } ( isAdmin + isStudent === false ) { message = 'Access denied'; }
Sahmad Nakumbe
3,423 PointsSahmad Nakumbe
3,423 Pointscondition?
Mark Sebeck
Treehouse Moderator 37,776 PointsMark Sebeck
Treehouse Moderator 37,776 PointsYou just need the else like this
the condition is what you had in () after the else