Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial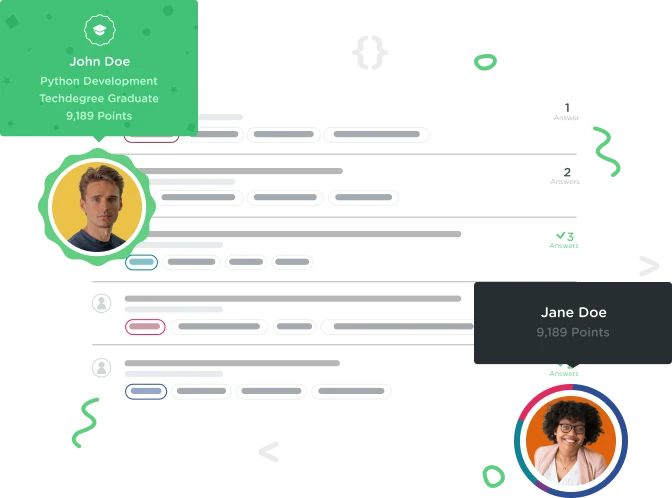
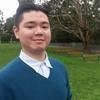
Ken Chung
17,585 PointsAdd a getInfo method to your Trout class that returns a string containing the common_name, flavor, record_weight, and sp
Challenge Task 6 of 7
I have no idea what to do :(
<?php
class Fish
{
public $common_name;
public $flavor;
public $record_weight;
function __construct($name, $flavor, $record){
$this->common_name = $name;
$this->flavor = $flavor;
$this->record_weight = $record;
}
public function getInfo() {
$output = "The {$this->common_name} is an awesome fish. ";
$output .= "It is very {$this->flavor} when eaten. ";
$output .= "Currently the world record {$this->common_name} weighed {$this->record_weight}.";
return $output;
}
}
class Trout extends Fish {
public $species;
function __construct($name, $flavor, $record, $species) {
parent::__construct($name, $flavor, $record);
$this->species = $species;
}
public getInfo() {
return = "$this->common_name tastes $this->flavor. " . "The record $this->record weighed $this->species.";
}
}
$brook_trout = new Trout("Trout", "Delicious", "14 pounds 8 ounces", "Brook");
$brook_trout = new getInfo();
?>
3 Answers
Tiffany McAllister
25,806 PointsHave a look at the getInfo() function of the Fish class above to give you an idea :)
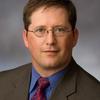
Ted Sumner
Courses Plus Student 17,967 PointsYour primary problem is that you do not use all of the variables. Also, if you use double quotes you can place the variables inside the quotes without adding concatenation because PHP evaluates the variable before the echo when using double quotes. Singles quotes will echo the variable name, not the value, so you would have to concatenate. This should pass:
<?php
public function getInfo() {
return "$this->species $this->common_name tastes $this->flavor. The record weight $this->species $this->common_name is $this->record_weight.";
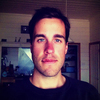
Sam Donald
36,305 PointsI got it to work but can someone give me some help understanding this a bit better.
Why do we need to set
$this->common_name
and
$this->record_weight
?
Haven't we assigned those values to $name
and $record
?