Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial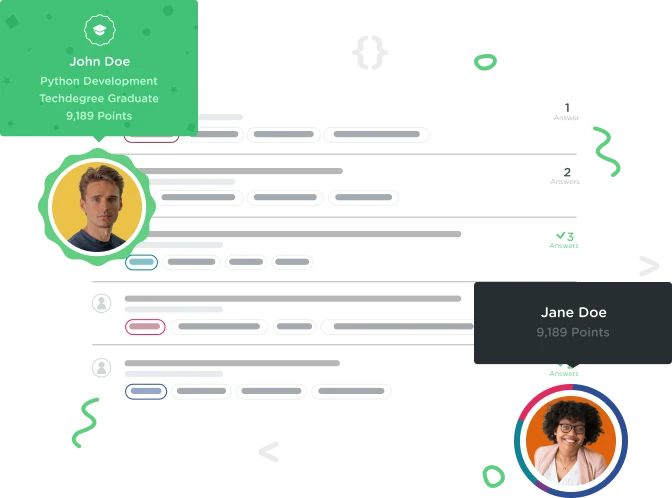
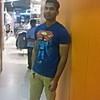
Malaya Manjula
6,028 PointsAdd a new method named ReverseNumbers that has a return type of IEnumerable<int> and uses a LINQ query to return the _nu
Querying with LINQ, Challenge task 2 of 2, Add a new method named ReverseNumbers that has a return type of IEnumerable<int> and uses a LINQ query to return the _numbers variable in reverse order.
Bummer! The first number in the result of your 'ReverseNumbers' method should be '10', since it's the greatest number, since it's the greatest number in the '_numbers' list.
using System.Collections.Generic;
using System.Linq;
namespace Treehouse.CodeChallenges
{
public class NumberAnalysis
{
private List<int> _numbers;
public NumberAnalysis()
{
_numbers = new List<int> { 2, 4, 6, 8, 10 };
}
public IEnumerable<int> NumbersGreaterThanFive()
{
return _numbers.Where(n => n > 5);
}
public IEnumerable<int> ReverseNumbers()
{
return _numbers.OrderByDescending(n=>_numbers);
}
}
}
4 Answers

James Churchill
Treehouse TeacherMalaya,
Let's take a closer look at your current solution.
public IEnumerable<int> ReverseNumbers()
{
return _numbers.OrderByDescending(n=>_numbers);
}
If we look at the documentation for the Enumerable.OrderByDescending
method, we'll see that it requires a parameter named keySelector
.
https://msdn.microsoft.com/en-us/library/bb534855(v=vs.110).aspx
The keySelector
parameter is "A function to extract a key from an element". When we're working with a collection of objects, we would pass a lambda to return the object property to sort on, like this.
OrderByDescending(x => x.Name) // assuming that the object "x" has a "Name" property
In this example though, we're working with a collection of integers. So what would we return from our lambda?
In your current solution, you're returning the field _numbers
, which is the collection that we're trying to sort. That's definitely not what the LINQ operator is expecting for a return value.
As it turns out, we just need to return each integer value that we're passed, like this.
OrderByDescending(n => n)
Looks a little weird, huh? Usually, when working with LINQ, you'll be working with a collection of objects, so you won't see these kinds of expressions very often.
I hope this helps.
Thanks ~James
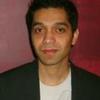
Mohammed Hossen
5,591 PointsMethod 1:
public IEnumerable<int> ReverseNumbers()
{
return _numbers.OrderByDescending(n => n >= 10);
}
Method 2:
public IEnumerable<int> ReverseNumbers()
{
return _numbers.OrderByDescending(n => n >= _numbers.Max());
}

Carel Du Plessis
Courses Plus Student 16,356 Pointshow would the Challenge task 2 of 2 code look like if we had to use OrderByDescending on the code below.
public IEnumerable<int> NumbersGreaterThanFive()
{
return _numbers.Where(n => n > 5);
}
I have tried to do it my self but I only receive compile errors

James Churchill
Treehouse TeacherCarel,
Could you please share your code?
Thanks ~James

Carel Du Plessis
Courses Plus Student 16,356 Pointsthe commented out code is me trying to use ThenByDescending key word to change the out put in a Descending order
after many failed attempts with OrderByDescending for example
_numbers.OrderByDescending(_numbers.Where(n => n > 5));
but i found a solution to replace them _numbers.Where(n => n > 5).Reverse();
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace LINQCodeChallenges
{
class Program
{
static void Main(string[] args)
{
NumberAnalysis mc = new NumberAnalysis();
IEnumerable<int> num1 = mc.NumbersGreaterThanFive();
Console.WriteLine("NumbersGreaterThanFive()");
foreach (int n in num1)
{
Console.WriteLine(n);
}
Console.WriteLine();
IEnumerable<int> num2 = mc.ReverseNumbersGreaterThanFive();
Console.WriteLine("Reverse Numbers Greater Than Five");
foreach (int n in num2) {
Console.WriteLine(n);
}
Console.ReadKey();
}
}
public class NumberAnalysis
{
private List<int> _numbers;
public NumberAnalysis()
{
_numbers = new List<int> { 2, 4, 6, 8, 10 };
}
public IEnumerable<int> NumbersGreaterThanFive()
{
return _numbers.Where(n => n > 5);
}
public IEnumerable<int> ReverseNumbersGreaterThanFive()
{
//return _numbers.OrderBy(n => n > 5).ThenByDescending(n => n);
return _numbers.Where(n => n > 5).Reverse();
}
}
}