Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial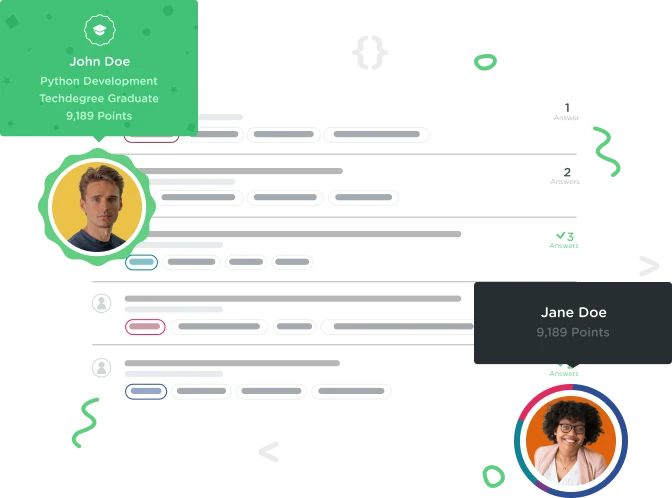

MUZ140348 Sympathy Makururu
10,209 PointsAdd a new test named test_not_in_anagrams and use self.assertNotIn() to make sure "code" isn't in the anagrams for "tree
Add a new test named test_not_in_anagrams and use self.assertNotIn() to make sure "code" isn't in the anagrams for "treehouse".
import unittest
from string_fun import get_anagrams
class AnagramTests(unittest.TestCase):
def test_in_anagrams(self):
self.assertIn("house", get_anagrams('Treehouse'))
def test_not_in_anagrams(self):
with self.assertNotIn('code', get_anagrams('Treehouse')):
get_anagrams('Treehouse')
import itertools
def is_palindrome(yarn):
"""Return whether or not a string is a palindrome.
A palindrome is a word/phrase that's the same in
both directions.
"""
return yarn == yarn[::-1]
def get_anagrams(*yarn):
"""Return a list of anagrams for a string."""
# If only one letter came in, return it
if yarn:
if len(yarn[0]) <= 1:
return list(yarn)
else:
raise ValueError("Must provide at least two letters")
# Get all of the words from the dictionary
words = set([
w.strip().lower() for w in open('words.txt')
])
output = set()
for thread in yarn:
thread = thread.lower()
# Find all possible anagrams
for i in range(2, len(thread)):
fibers = set(
[''.join(w) for w in itertools.permutations(thread, i)]
)
output.update(fibers.intersection(words))
# Finally, return all of the combinations that are in the dictionary
return sorted(list(output))
1 Answer

Iain Simmons
Treehouse Moderator 32,305 PointsYou don't need to use with
.
You're pretty much using the same code as the assertIn
, but with assertNotIn
and "code"
instead of "house"
.
MUZ140348 Sympathy Makururu
10,209 PointsMUZ140348 Sympathy Makururu
10,209 PointsI GOT IT RIGHT NEVER MIND:
def test_not_in_anagrams(self): with self.assertNotIn("code", get_anagrams('Treehouse')): pass