Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial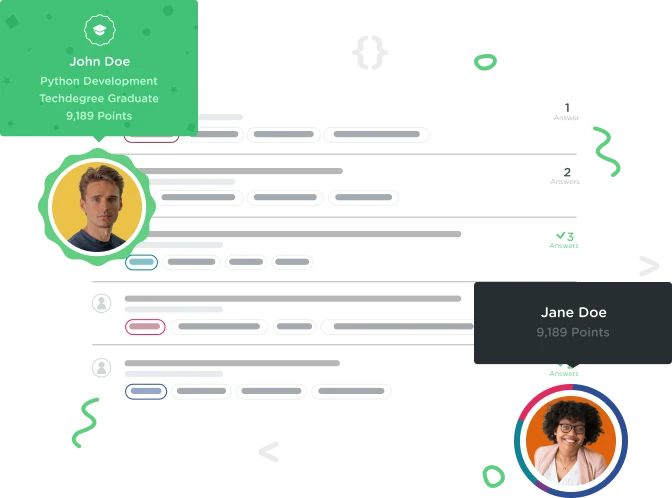

Matthew Paek
2,420 PointsAdd a second parameter to the constructor named reactionTime after the existing parameter. What am I doing wrong?
Add a second parameter to the constructor named reactionTime after the existing parameter and use it to initialize the value of the ReactionTime field.
I keep getting this message:
Frog.cs(17,16): error CS1520: Class, struct, or interface method must have a return type
I created a constructor to initialize my variables, so I'm a little confused as to why I'm getting an error message.
namespace Treehouse.CodeChallenges
{
class Frog
{
public readonly int TongueLength;
public Frog(int tongueLength)
{
TongueLength = tongueLength;
}
public bool EatFly(int distanceToFly)
{
return TongueLength >= distanceToFly;
}
public readonly int ReactionTime;
public Reaction(int reactionTime)
{
ReactionTime = reactionTime;
}
}
}
2 Answers

Chris Jones
Java Web Development Techdegree Graduate 23,933 PointsThe constructor is the same name as the class name. So, public Frog(int tongueLength)
is the constructor for the Frog
class. You need to add a new parameter to the constructor to initialize the new field, ReactionTime.
Just best practice, but I would put the all class fields directly below the class, so:
class Frog
{
public readonly int TongueLength;
public readonly int ReactionTime;
// class methods here...
}

Matthew Paek
2,420 PointsThank you!! It took me a while to figure it out after I looked at it, but I realized that there cannot be two constructors for one class (I wrote it myself: initialize variables, can't believe I didn't piece it together).

Chris Jones
Java Web Development Techdegree Graduate 23,933 PointsWell, you can have multiple constructors that take different parameters, but they all build the same object. So, for instance you could do:
public Frog(int tongueLength)
{
TongueLength = tongueLength;
}
public Frog()
{
}
The first constructor will create a Frog object and requires an argument to be passed to the tongueLength
parameter. The second constructor does not require an argument because it does not have a parameter and creates a new Frog object without any fields being initialized.
Let me know if you have any more questions.