Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial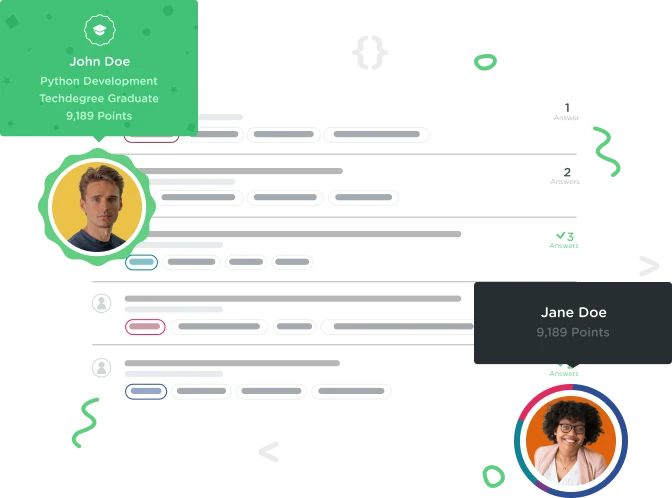

Jon Simeon
3,037 PointsAdd a ton of songs to playlist?
I noticed I was repeating myself a lot when I had more than 2 songs to add to playlist. I.e. :
var song1 = new Song('Origin of Love', 'Hedwig and the Anry Inch', '3:45');
var song2 = new Song('I Don\'t Care Much', 'Cabaret', '1:40');
var song3 = new Song('Song for Someone', 'U2', '3:46');
var song4 = new Song('Want U', 'Lo-fi-fnk', '4:40');
//etc.
playlist.add(song1);
playlist.add(song2);
playlist.add(song3);
playlist.add(song4);
//etc.
Is there any way to use a for loop to add all or several songs to the playlist?
4 Answers
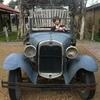
Brendan Moran
14,052 PointsThere is a thing called the "arguments object" that all functions have. Use it inside your playlist.add method that you are defining in the playlist.js file. The arguments object is an array-like object, but not technically an array. It does not have any array properties, except for length. It CAN be used inside a for loop within your method. Here's what I did:
Playlist.prototype.add = function(songs) {
for (i = 0; i < arguments.length; i++)
this.songs.push(arguments[i]);
};
Then, in my app.js file:
playlist.add(hereComesTheSun, walkingOnSunshine);
When I go to the console and type playlist.songs, it shows me an array with both song objects in it. It works!
Notice that although I defined a "songs" parameter for my Playlist.prototype.add method, I am not using it anywhere in the body of the function. The parameter name there is just to remind me that some arguments will be passed in. You could write "arguments" there, or just leave it blank. The arguments object exists independently of any parameters you create. I could delete the songs parameter, and as long as I am passing those two song objects in as arguments when I call the method, my arguments object will have access to those two arguments!
javaScript is not strict about arguments, you can pass them in to any function, in any amount or data type. The arguments object inside your function will give you access to whatever arguments may have been passed in, even if they weren't expected. It is good for situations where you think someone might try to pass in a variable amount of arguments. I think there is a good use for it here.
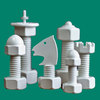
Steven Parker
230,274 PointsYou could use a loop if they were in an array, but why not just add them directly without the intermediate variable?
playlist.add(new Song('Origin of Love', 'Hedwig and the Anry Inch', '3:45'));
playlist.add(new Song('I Don\'t Care Much', 'Cabaret', '1:40'));
playlist.add(new Song('Song for Someone', 'U2', '3:46'));
playlist.add(new Song('Want U', 'Lo-fi-fnk', '4:40'));
//etc.

Jon Simeon
3,037 PointsThe whole .add method from the video added them to an array in the playlist object, that's why putting variable names to an array felt a little redundant to me.
I like your solution if I were to simply just add all the songs, but I guess I was thinking more if I wanted to add some songs. Like if i had 20 songs and I only wanted to add the last 5 songs or maybe just a couple.

Jon Simeon
3,037 PointsNever mind, I figured it out. Yeah just placing each new song I log into an array without and intermediate variable works.
var collection = [new Song('Origin of Love', 'Hedwig and the Anry Inch', '3:45'), new Song('I Don\'t Care Much', 'Cabaret', '1:40'), new Song('Song for Someone', 'U2', '3:46'), new Song('Want U', 'Lo-fi-fnk', '4:40')];
for (var i = 0, len = collection.length; i < len; i++) {
playlist.add(collection[i]);
}
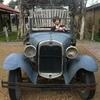
Brendan Moran
14,052 PointsCheck out the solution I came up with. You can do it with or without an intermediate variable, and you won't need to create an array in your app.js. Turns out there is an opportunity to take advantage of something javaScript is doing behind the scenes when you call your playlist.add method.

amourief
5,469 PointsSomething like this could work too: an array of song objects - then iterate through them with a for loop. It would require further changes to add each song property (e.g. name, artist etc) in place in the HTML.
var songs = [
{
name: "Love is Blindness",
artist: "U2",
duration: "2:34",
index: 0
},
{
name: "Say Goodbye",
artist: "Madonna",
duration: "2:09",
index: 1
},
{
name: "The Prayer",
artist: "Josh Groban",
duration: "4:09",
index: 2
},
{
name: "Jimmy Thing",
artist: "Dave Matthews Band",
duration: "2:09",
index: 3
}
];
for (var song in songs) {
playList.add(songs[song]);
//console.log(songs[song]);
}