Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial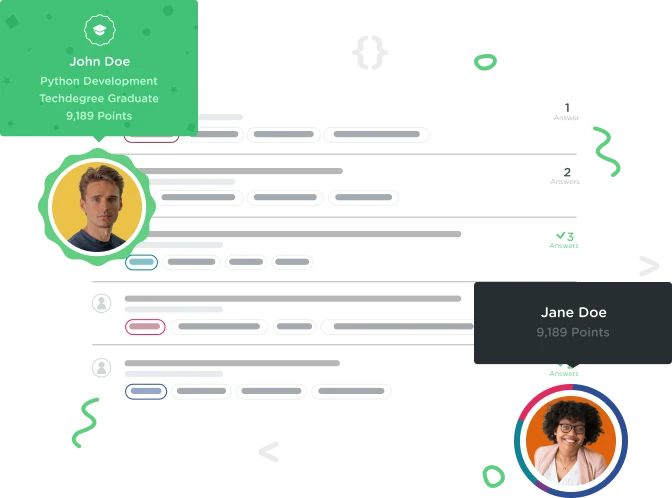

Mahmud Khan
4,505 PointsAdd a view named multiply. Give multiply a route named /multiply. Make multiply() return the product of 5 * 5.
Add a view named multiply. Give multiply a route named /multiply. Make multiply() return the product of 5 * 5. Remember, views have to return strings.
What is wrong with my code?
from flask import Flask
app = Flask(__name__)
@app.route("/multiply")
@app.route("/multiply/<num1>/<num2>")
def multiply(num1=5, num2=5)
R = num1 * num2
return str(R)
2 Answers
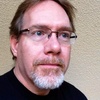
Chris Freeman
Treehouse Moderator 68,423 PointsFirst, there is a typo. You need a colon (:) at the end of the def
statement. This will get you through Task 1.
The second error is less obvious. When the code is tested without num1
and num2
arguments, they get the default value of 5. But when the code is tested with /multiply/10/10
, the value assigned to both num1
and num2
is the string "10". all URL arguments are passed in as strings. An error is thrown because you can't multiply two string.
Change the code to convert to strings. This will get you through Task 2
from flask import Flask
app = Flask(__name__)
@app.route("/multiply")
@app.route("/multiply/<num1>/<num2>")
def multiply(num1=5, num2=5): # <-- add missing colon
R = int(num1) * int(num2)
return str(R)

Mahmud Khan
4,505 PointsThank you for your help, it worked. I should have checked for typos.