Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial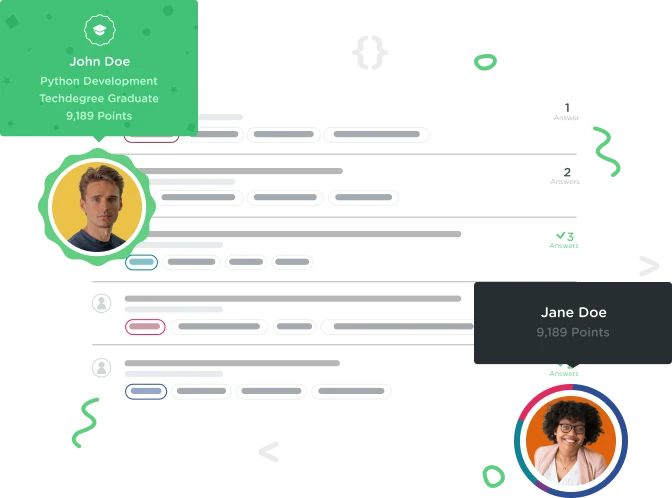

vincent batteast
51,094 PointsAdd an Equals method to the VocabularyWord class that returns true if the words of two VocabularyWord objects are the sa
VocabularyWord.cs(4,18): warning CS0659: Treehouse.CodeChallenges.VocabularyWord' overrides Object.Equals(object) but does not override Object.GetHashCode()
VocabularyWord.cs(20,34): error CS0118:
Treehouse.CodeChallenges.VocabularyWord' is a type' but a
variable' was expected
VocabularyWord.cs(20,34): error CS0119: Expression denotes a type', where a
variable', value' or
method group' was expected
Compilation failed: 2 error(s), 1 warnings
using System;
namespace Treehouse.CodeChallenges
{
public class VocabularyWord
{
public string Word { get; private set; }
public VocabularyWord(string word)
{
Word = word;
}
public override string ToString()
{
return Word;
}
public override bool Equals(object word)
{
if (Word.Equals(word) && VocabularyWord != null )
{
return false;
}
else
{
return true;
}
}
}
}
8 Answers
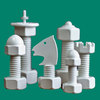
Steven Parker
229,744 PointsIt looks like you missed my first hint last time.
I'll repeat it for you in this batch:
-
if (!(obj is Word))
"Word" is not a type (but "VocabularyWord" would be!)
-
that.Equals(Word)
"that" is the whole object — but that.Word is the string
And you almost got the formatting, but instead of 3 apostrophes ( ' ), you need 3 accents ( ` ).
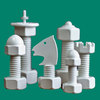
Steven Parker
229,744 PointsIn my hints I gave you direct substitutions for the problem terms. If you just exchange them as shown you will pass the challenge.

vincent batteast
51,094 PointsThanks i'm sorry. I don't know why I just didn't get that the first time around.

vincent batteast
51,094 Pointslike this
public override bool Equals(object obj)
{
if (Word.Equals(obj) && VocabularyWord != null )
{
return false;
}
else
{
return true;
}
}
}
}
this didn't work.
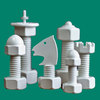
Steven Parker
229,744 PointsThat's essentially the same as the code I commented on, you just renamed the argument. You're still using "VocabularyWord" like a variable, and testing it against null. And still using the Equals method of the string Word to compare it directly to the object.
I added some hints to my answer.

vincent batteast
51,094 Pointspublic override bool Equals(object obj) { if(!(obj is Word)) { return false; } else if (obj.Equals(word)) { return true; } }
this is were I'm at now. I think I'm over looking some part. I just not understand why this is not working.

vincent batteast
51,094 PointsI'm so sorry I'm still not getting it. do you have some article that explain this or some code that I can learn form. I'm just missing something and I don't know what that is.
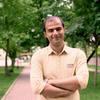
S.Amir Nahravan
4,008 Pointspublic override bool Equals(object obj)
{
if (!(obj is VocabularyWord))
{
return false;
}
VocabularyWord that = obj as VocabularyWord;
return this.Word == that.Word;
}
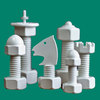
Steven Parker
229,744 PointsYou have a few issues:
- VocabularyWord is a type but you used it as a variable
- you use the Equals method of a string to compare it with a VocabularyWord object
- you may want to compare the new string with the one the object contains instead
Here's a few hints:
- remember that compiler errors can be seen in the
Preview
- you may first want to test that the argument is a VocabularyWord
- then you might test for equality with the word inside the argument object
- you can return the result of your evaluation directly instead of using an If to explicitly return true or false
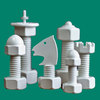
Steven Parker
229,744 PointsSo, based on your latest code:
-
if (!(obj is Word))
"Word" is not a type, but "VocabularyWord" would be
-
if (obj.Equals(word))
you don't have "word" anymore, you changed it to "obj", right?
You'll probably want to compare your own "Word" (capital "W") property to the one of the object passed as an argument. To be able to get to it, you might need to apply a cast.
And please use the instructions for code formatting in the Markdown Cheatsheet pop-up below the "Add an Answer" area.

vincent batteast
51,094 Pointshere were I'm at now. Thank you for the help with this.
'''C# public override bool Equals(object obj) {
if(!(obj is Word))
{ return false;
}
var that = obj as VocabularyWord;
return (that.Equals(Word));
} '''