Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial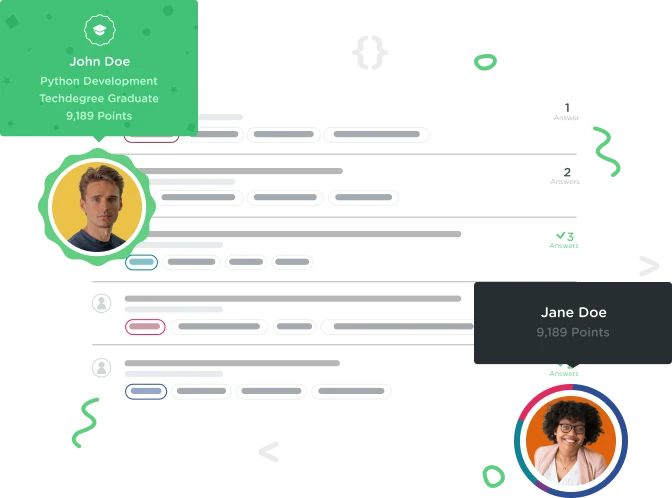

chris slaats
5,996 PointsAdd button not working properly
hey for this Todo app im up to the part of adding new tasks. Unfortunately my add button isn't working properly. Every time i press the add button i am getting an error code.
Uncaught TypeError: undefined is not a function.
In regards these two functions, createNewTaskElement on line 12 and addTask on line 51
I'm sure it is just something small that ive over looked and any help would be greatly apreciated, thank you.
//problem: user interation doesnt provide desired results
//soltuion: add interactivity so the user can manage daily tasks
var taskInput = document.getElementById("new-task"); //new-task
var addButton = document.getElementsByTagName("button")[0]; //firs button
var incompleteTaskHolder = document.getElementById("incomplete-tasks"); //incomplete-tasks
var completeTasksHolder = document.getElementById("completed-tasks"); //completed-tasks
//new task list item
var createNewTaskElement = function (taskString) {
//create list item
var listItem = Document.createElement("li");
//input (checkbox)
var checkBox = Document.createElement("input"); //checkbox
//label
var label = Document.createElement("label");
//input (text)
var editInput = Document.createElement("input");
//button.edit
var editButton = Document.createElement("button");
//button.delete
var deleteButton = Document.createElement("button");
//each element needs modifying
checkBox.type = "checkbox";
editInput.type = "text";
editButton.innerText = "Edit";
editButton.class = "edit";
deleteButton.innerText = "Delete";
deleteButton.class = "delete";
label.innerText = taskString;
//each element needs appending
listItem.appendChild(checkBox);
listItem.appendChild(label);
listItem.appendChild(editInput);
listItem.appendChild(editButton);
listItem.appendChild(deleteButton);
return listItem;
}
//add a new task
var addTask = function () {
console.log("add task...");
//create a new list item with the text from the #new-task
var listItem = createNewTaskElement(taskIncomplete.value);
//append listItem to incompleteTaskHolder
incompleteTaskHolder.appendChild(listItem);
bindTaskEvents(listItem, taskCompleted)
}
//edit an existing task
var editTask = function (){
console.log("edit task...");
//when the edit button is pressed
//if the class of the parent is .editMode
//switch from .editMode
//label text become the inputs value
//else
//switch to .editMode
//input value becomes the labels text
//toggel .editMode on the parent
}
//delete an existing task
var deleteTask = function(){
console.log("delete task...");
var listItem = this.parentNode;
var ul = listItem.parentNode;
//remove the parent list item from the ul
ul.removeChild(listItem);
}
//mark a task as complete
var taskCompleted = function() {
console.log("task complete...");
//append the task list item to the #completed-tasks
var listItem = this.parentNode
completeTasksHolder.appendChild(listItem);
bindTaskEvents(listItem, taskIncomplete);
}
//mark a task as incomplete
var taskIncomplete = function(){
console.log("task incomplete...");
//append the list item to the #incomplete-tasks
var listItem = this.parentNode
incompleteTaskHolder.appendChild(listItem);
bindTaskEvents(listItem, taskCompleted);
}
var bindTaskEvents = function (taskListItem, checkBoxEventHandler) {
console.log("Bind list item events");
//select taskListItem's children
var checkBox = taskListItem.querySelector("input[type=checkbox]");
var editButton = taskListItem.querySelector("button.edit");
var deleteButton = taskListItem.querySelector("button.delete");
//bind the editTask to edit button
editButton.onclick = editTask;
//bind the deleteTask to delete button
deleteButton.onclick = deleteTask;
//bind the checkBoxEventHandler to checkbox
checkBox.onchange = checkBoxEventHandler;
}
//set the click handler to the addTask function
addButton.onclick = addTask;
//cycle over incompleteTaskHolder ul list items
for (i = 0; i < incompleteTaskHolder.children.length; i++) {
//bind events to list items children (taskCompleted)
bindTaskEvents(incompleteTaskHolder.children[i], taskCompleted);
}
//cycle over completeTasksHolder ul list items
for (i = 0; i < completeTasksHolder.children.length; i++) {
//bind events to list items children (taskIncompleted)
bindTaskEvents(completeTasksHolder.children[i], taskIncomplete);
}
2 Answers
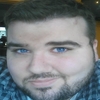
Marcus Parsons
15,719 PointsWell, taking a closer look at your code, I changed the "Document" references to "document" when creating those new elements and now the add button works; however, it's going to return "undefined" until you wire up the "editTask" function.
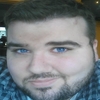
Marcus Parsons
15,719 PointsHey chris slaats,
I think at least one part of the error you're seeing is from your 4th line down, when you initialize your completeTasksHolder. In the string portion of getElementById where you are naming the id you'd like to get, you have spaces in between "completed-tasks" when there should be none. Delete that space in between "completed-" and "tasks" and see if that did the trick.

chris slaats
5,996 Pointsthanks for the comment but unfortunately that wasnt the problem as that was an accident when posting the code and wasnt actually in my program :\
chris slaats
5,996 Pointschris slaats
5,996 Pointsthat was it thank you very much
Marcus Parsons
15,719 PointsMarcus Parsons
15,719 PointsNo problem, Chris! If you get too frustrated on anything else, especially about this program, you can post back here, or make a new question. Happy coding!