Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial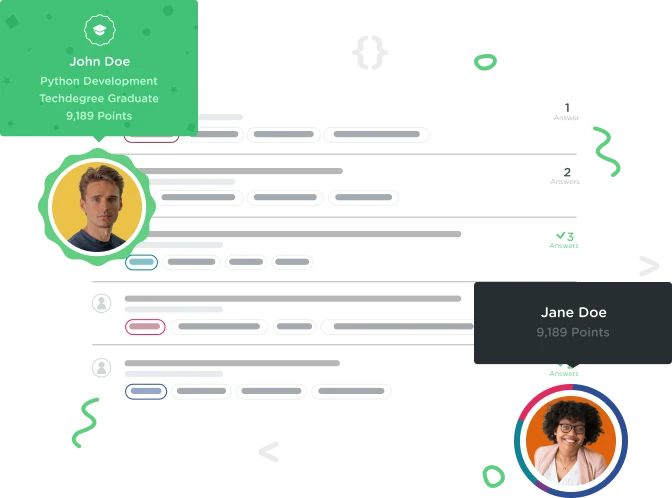
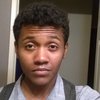
Ricky Sparks
22,249 Pointsadd code to set the member variables from the Parcel???
Not sure what I am doing wrong???
public class VideoGame implements Parcelable {
public String mTitle = "";
public int mYear = 0;
public VideoGame() {
// intentionally blank
}
// getters and setters omitted for brevity!
@Override
public int describeContents() {
return 0;
}
@Override
public void writeToParcel(Parcel dest, int flags) {
dest.writeString(mTitle);
dest.writeInt(mYear);
}
public VideoGame(Parcel in) {
// Task 1 code here!
mVideoGame = in.readDouble();
}
public static final Creator<VideoGame> CREATOR = new Creator<VideoGame>() {
@Override
public VideoGame createFromParcel(Parcel source) {
// Task 2 code here!
return new VideoGame(source);
}
@Override
public VideoGame[] newArray(int size) {
// Task 3 code here!
return new VideoGame[size];
}
};
}
1 Answer
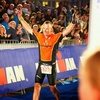
Steve Hunter
57,712 PointsHi Ricky,
The variables that need setting are mTitle
and mYear
within the class we're dealing with. They were stored in that order within the parcel. We're now using that parcel within the constructor of the VideoGame
class to create an instance of a video game.
The constructor needs to set both the variables above. But first they need extracting from the parcel which is called in
. We should extract the two variables in the same order, as above, and also make sure we use the appropriate method based on the type of the variable. mTitle
is a String, mYear
is an int. So, for example, we can get the title with in.getString();
. Building from that and completing the constructor gives you:
public VideoGame(Parcel in) {
// Task 1 code here!
mTitle = in.readString();
mYear = in.readInt();
}
I hope that makes sense.
Steve.
Ricky Sparks
22,249 PointsRicky Sparks
22,249 PointsThanks alot :D it did help make sense on referring for string and int from the code above the task.
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsCool - glad you got it sorted. :-)
Steve.