Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial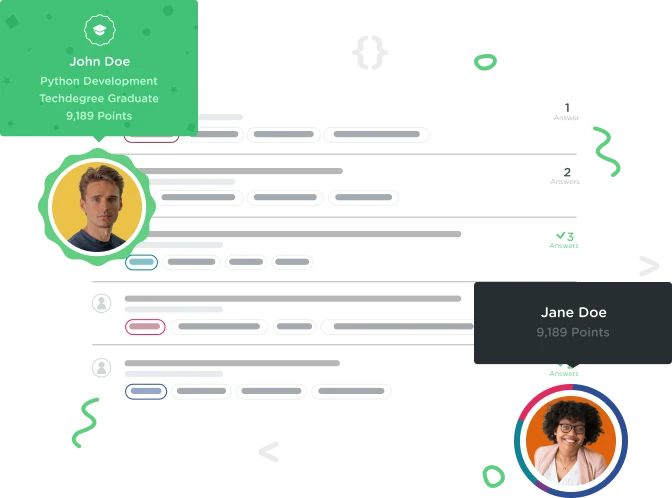
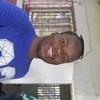
Innocent Ngwaru
7,554 PointsAdd current_user check
I have been stuck for a while with this Task 2. I introduced if.current_user.is_authenticated() but its telling that it didnt find the right link. Please help where am I getting it wrong. I am now frustrated.
from flask import Flask, g, render_template, flash, redirect, url_for
from flask.ext.bcrypt import check_password_hash
from flask.ext.login import LoginManager, login_user, current_user, login_required, logout_user
import forms
import models
app = Flask(__name__)
app.secret_key = 'this is our super secret key. do not share it with anyone!'
login_manager = LoginManager()
login_manager.init_app(app)
login_manager.login_view = 'login'
@login_manager.user_loader
def load_user(userid):
try:
return models.User.select().where(
models.User.id == int(userid)
).get()
except models.DoesNotExist:
return None
@app.before_request
def before_request():
g.db = models.DATABASE
g.db.connect()
g.user = current_user
@app.after_request
def after_request(response):
g.db.close()
return response
@app.route('/register', methods=('GET', 'POST'))
def register():
form = forms.SignUpInForm()
if form.validate_on_submit():
models.User.new(
email=form.email.data,
password=form.password.data
)
flash("Thanks for registering!")
return render_template('register.html', form=form)
@app.route('/login', methods=('GET', 'POST'))
def login():
form = forms.SignUpInForm()
if form.validate_on_submit():
try:
user = models.User.get(
models.User.email == form.email.data
)
if check_password_hash(user.password, form.password.data):
login_user(user)
flash("You're now logged in!")
else:
flash("No user with that email/password combo")
except models.DoesNotExist:
flash("No user with that email/password combo")
return render_template('register.html', form=form)
@app.route('/secret')
@login_required
def secret():
return "I should only be visible to logged-in users"
@app.route('/logout')
def logout():
logout_user()
return redirect(url_for('login'))
@app.route('/')
def index():
return render_template('index.html')
<!doctype html>
<html>
<head>
<title>Lunch</title>
</head>
<body>
<nav>
{% if current_user.is_authenticated %}
<a href="{{ url_for('logout') }}" class="icon-power">Sign Out</a>
{% else %}
<a href="{{ url_for('login') }}" class="icon-power">Sign In</a>
<a href="{{ url_for('register') }}" class="icon-power">Sign Up</a>
{% endif %}
</nav>
<div class="messages">
{% with messages = get_flashed_messages() %}
{% for message in messages %}
<div>{{ message }}</div>
{% endfor %}
{% endwith %}
</div>
{% block content %}{% endblock %}
</body>
</html>
2 Answers

Myers Carpenter
6,421 PointsI introduced if.current_user.is_authenticated() but its telling that it didnt find the right link.
You are really close. In layout.html you have
{% if current_user.is_authenticated %}
What is is_authenticated
? A variable? A callable (AKA a function or a method)? If it's a callable do you want to call it?
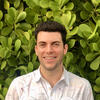
justlevy
6,326 PointsI don't see my username when I'm logged in. I followed the notes stating to remove the parentheses after is_authenticated
.
Code snippet:
<header>
<div class="row">
<div class="grid-33">
<a href="{{ url_for('index') }}" class="icon-logo"></a>
</div>
<div class="grid-33">
<!-- Say Hi -->
<h1>Hello{% if current_user.is_authenticated %} {{ current_user.username }} {% endif %}</h1>
</div>
<div class="grid-33">
<!-- Log in/Log out -->
{% if current_user.is_authenticated %}
<a href="{{ url_for('logout') }}" class="icon-power" title="Log out"></a>
{% else %}
<a href="{{ url_for('login') }}" class="icon-power" title="Log in"></a>
<a href="{{ url_for('register') }}" class="icon-profile" title="Register"></a>
{% endif %}
</div>
</div>
</header>
V K
5,237 PointsV K
5,237 PointsI had the same issue. My guess is it's a callable therefore you need to add () to it. The reason some people may have a problem is due to the notes stating
"In this video and the others in this course, you'll see me using {{ current_user.is_authenticated() }}. At the time of filming, this was the correct way to use the is_authenticated() method on the UserMixin from flask-login. BUT, as is often the case in open source, things have changed. You'll now want to always use it as a property instead. So, use {{ current_user.is_authenticated }} instead. No parentheses!"
I thought that I would not need to add () due to the notes...but alas.
{% if current_user.is_authenticated() %}
was the solution.