Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial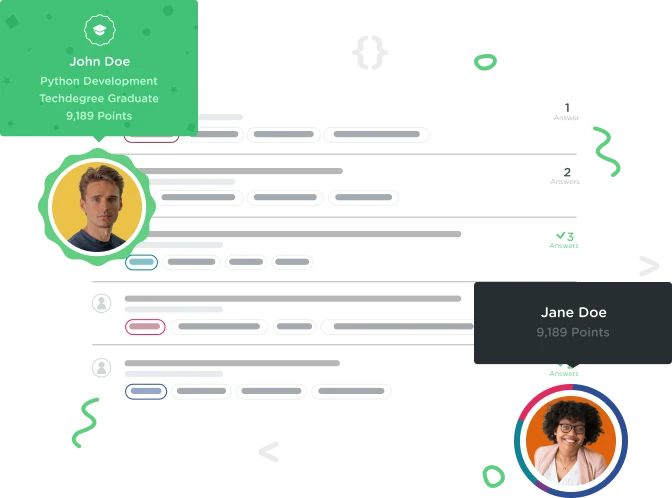

Jeremy Woodbridge
25,278 PointsAdd Express’s static server middleware as a second argument to the app.use(‘/static’) function, with the path to the pub
I always get a bummer: _dirname is not defined. What am I doing wrong?
'use strict';
var express = require('express'),
posts = require('./mock/posts.json');
var app = express();
app.use("/static", express.static(_dirname + "/public"))
app.set('view engine', 'jade');
app.set('views', __dirname + '/templates');
app.get('/', function(req, res){
res.render('index');
});
app.get('/blog/:title?', function(req, res){
var title = req.params.title;
if (title === undefined) {
res.status(503);
res.send("This page is under construction!");
} else {
var post = posts[title] || {};
res.render('post', { post: post});
}
});
app.listen(3000, function() {
console.log("The frontend server is running on port 3000!");
});
3 Answers
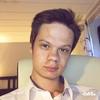
Edvin Erikson
4,859 PointsHi. This is simply caused by a miss spell. __dirname
is the correct name.
You can see all the globals defined by Node here: https://nodejs.org/api/globals.html#globals_dirname

Jeremy Woodbridge
25,278 PointsI didn't see that needed 2 underscore characters. I feel silly, thank you very much sir

Ary de Oliveira
28,298 PointsDry Answer ... Challenge Task 1 of 2 Call Express’s use method, with the parameter “/static”. This is the URL that the static server will be mounted to.
app.use("/static", express.static(__dirname + "/public"))

larsberg
58,676 PointsThe correct answer uses two underlines, otherwise you'll get an error. app.use("/static", express.static(__dirname + "/public"))
Jeremy Woodbridge
25,278 PointsJeremy Woodbridge
25,278 PointsThis line specifically is the issue
app.use("/static", express.static(_dirname + "/public"))