Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial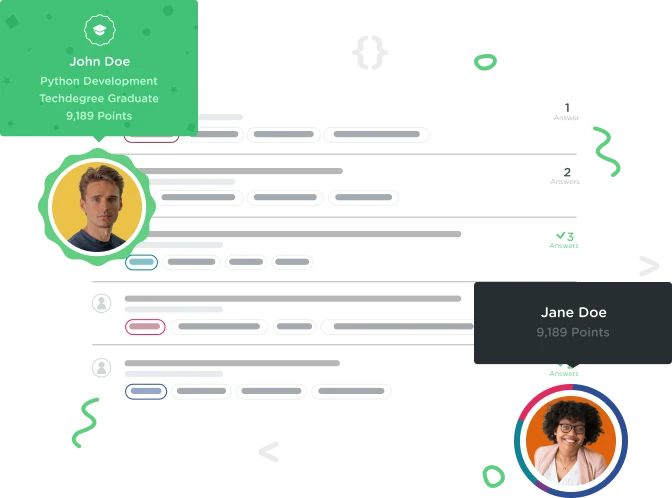

Desmond Dallas
6,985 PointsAdd remove button to each table row
Hi all, Im having difficulty here. Im trying to add a remove button to each table row in the tbody but no luck. Would be great if you could explain where Im going wrong.
<!DOCTYPE html> <html lang="en"> <head> <title>Scrum App!</title> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no"> <link rel="stylesheet" type="text/css" href="Scrum_App_test_2.css"> <script src="scrum_app_test_2.js"></script> </head> <body>
<form method="POST" action="" name="add"">
<fieldset>
<h1>Scrum App!</h1>
<label>Name</label><br>
<input type="text" id="name" placeholder="Name" required>*<span id="name-trim"></span><br />
<label>Email</label><br>
<input type="text" id="email" placeholder="Email" onkeyup="email_validate(this.value);" required>*<span id="error-email"></span><br />
<label>Phone Number</label><br>
<input type="text" id="phonenumber" placeholder="Phone Number" onkeyup="checkPhone(this.value);" required>*<span id="error-phone"></span><br />
<button value="Add" id="addToTable" onclick="add_update(event);" >Add</button>
<button onclick="remove_update(event)">Remove</button>
</fieldset>
</form>
<table id"table" border="1"
<tr>
<td>Name</td>
<td>Email</td>
<td>Phone</td>
<td></td>
</tr>
<tbody id="after_add">
<td></td>
<td></td>
<td></td>
</table>
</div>
</body>
</html>
<script> // email validation var flag=0; function name_trim(str) { str = str.replace(/^\s+|\s+$/g, ''); document.getElementById("name-trim").innerHTML = str; }
function email_validate(email) { var regMail = /^([_a-zA-Z0-9-]+)(.[_a-zA-Z0-9-]+)*@([_a-zA-Z0-9-]+.)+([a-zA-Z]{2,3})$/;
if (regMail.test(email) == false) {
document.getElementById("error-email").innerHTML = "<span>Please enter a valid email address</span>";
flag=1;
}
else {
document.getElementById("error-email").innerHTML = "<span>You have entered a valid email address</span>";
flag=0;
}
}
function checkPhone(phone) {
var regPhone = /^[0-9]{11}$/;
if (regPhone.test(phone) == false)
{
document.getElementById("error-phone").innerHTML = "<span>Please enter a valid number</span>";
flag=1;
}
else
{
document.getElementById("error-phone").innerHTML = "<span>Thank you, you have enetered a valid Phone Number</span>";
flag = 0;
}
}
var i; var count = 3; var array = new Array; var newObj;
function add_update(event) { event.preventDefault();
var name = document.getElementById("name").value;
var email = document.getElementById("email").value;
var phone = document.getElementById("phonenumber").value;
newObj ={
"name":name,
"email":email,
"phone":phone,
};
for (var k in newObj) {
array.push(newObj [k]);
}
var tr = document.createElement('tr');
tr.setAttribute("id","Row");
var td1 = document.createElement('td');
var td2 = document.createElement('td');
var td3 = document.createElement('td');
var name = document.createElement('name');
var email = document.createElement('email');
var phonenumber = document.createElement('phonenumber');
td1.appendChild(name);
td2.appendChild(email);
td3.appendChild(phonenumber);
tr.appendChild(td1);
tr.appendChild(td2);
tr.appendChild(td3);
after_add.appendChild(tr);
text="<tr>";
for(i=1; i <=count; i++)
{
text+="<td>"+array[i-1]+"</td>";
if((i%3==0)&&(i!=0))
{
text+="</tr>";
}
}
document.getElementById("after_add").innerHTML =text;
count=count+3;
document.forms['add'].reset()
}
function remove_update(event) { event.preventDefault(); let fc = after_add.lastChild;
after_add.removeChild( fc );
fc = after_add.firstChild;
Len = array.length-3;
array.splice(0, Len);
count=count-3;
}
</script> </table> </body> </html>
1 Answer
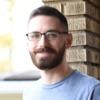
Justin Cantley
18,068 PointsSo this is the part of the code I believe you're referring to:
<table id"table" border="1"
<tr>
<td>Name</td>
<td>Email</td>
<td>Phone</td>
<td></td>
</tr>
<tbody id="after_add">
<td></td>
<td></td>
<td></td>
</table>
To start, there are a few errors in the syntax. The opening table tag needs a closing arrow as well as an = sign between id and "table". Also, you must have a closing <tbody> tag. All of that implemented will look like this:
<table id="table" border="1"> // closing arrow and equal sign
<tr>
<td>Name</td>
<td>Email</td>
<td>Phone</td>
<td></td>
</tr>
<tbody id="after_add">
<td></td>
<td></td>
<td></td>
</tbody> // closing tbody tag
</table>
Now on to your original question. I don't see a table row inside of the tbody, but I do see one in the table element as its first child element. Adding a remove button to that is simple:
<tr>
<button onclick="remove_update(event)">Remove</button>
<td>Name</td>
<td>Email</td>
<td>Phone</td>
<td></td>
</tr>
That's all there is to it. You can move the position of the button anywhere within that row to your liking. Hope this helps!
Neil McPartlin
14,662 PointsNeil McPartlin
14,662 PointsHi Desmond. Whilst an 'eagle eyed' experienced developer might be able to speed read your code as shown, us fellow students would be put off as the code is not displaying well for some reason. It looks to be a single file e.g. index.html, which is fine, but I'd like to be able to copy/paste it into a code editor such as Visual Studio Code, which automatically highlights some of the more basic coding mistakes.
Please try posting your code again like so...
```html
paste all your code in one go, here
```