Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial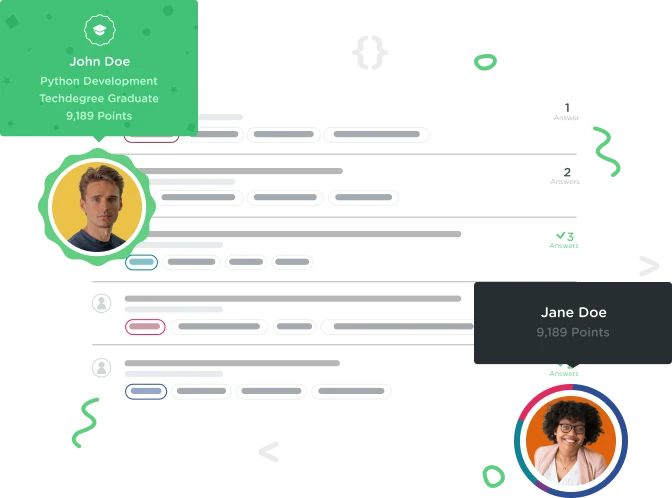
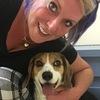
bevinwg
5,196 PointsAdd the code to send the AJAX request
I am having a complete air brain moment here. Can someone help me understand what I am doing wrong?
At first it kept coming back with a response: It looks like the 1st answer is no longer accurate (even though I didn't change it at all). Now I get a response asking: Did you call send?
var request = new XMLHttpRequest();
request.onreadystatechange = function () {
if (request.readyState === 4) {
document.getElementById("footer").innerHTML = request.responseText;
}
};
request.open('GET','footer.html');
function sendAJAX() {
request.send();
document.getElementsByID('load').style.display = "none";
}
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>AJAX with JavaScript</title>
<script src="app.js"></script>
</head>
<body>
<div id="main">
<h1>AJAX!</h1>
</div>
<div id="footer"></div>
</body>
</html>
3 Answers
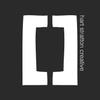
Angelica Hart Lindh
19,465 PointsHi Bevin,
Henrik's answer is correct, you have placed the .send() method within a named function but haven't invoked it in the snippets you have shown. Below is an example for you with XHR call.
request = new XMLHttpRequest();
request.open("GET", "footer.html");
request.send();
request.onreadystatechange = function() {
if(request.readyState === 4) {
if(request.status === 200) {
console.log(request.responseText);
document.getElementById("footer").innerHTML = request.responseText;
}
}
}
You maybe interested as well to see the new fetch API in JavaScript. This is implemented to make working with HTTP requests and responses easier. While not fully supported in all browsers you can start using it today with cross-browser support with polyfill.
I've written a little jsfiddle example, here.
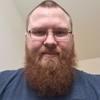
Henrik Christensen
Python Web Development Techdegree Student 38,322 PointsThe reason it ask you "Did you call send?" might be because your "request.send();" is inside a function and you don't call the function anywhere :-)
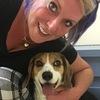
bevinwg
5,196 Pointsahhhhhhh! Ok, thanks for pointing me in the right direction. Let me review it again with fresh mind and see if I can get it. Thank you both!
bevinwg
5,196 Pointsbevinwg
5,196 PointsThank you for these links as well. I have marked them to check out and follow to gain more info.
Very appreciated!