Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial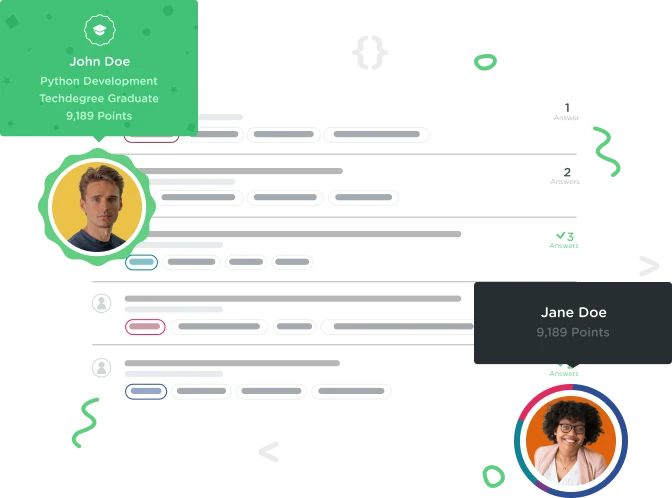

Zachary Vacek
11,133 PointsAdded a "Difficulty" Option to Guessing Game
Hello all,
I decided to attempt to add a difficulty option to the number guessing game. The user can select "EASY" - where the user guesses a number between 1 and 10 - or "HARD" - where the user has to guess a number between 1 and 20.
Let me know what you think! Any suggestions?
import random
guessed_nums = []
allowed_guesses = 5
difficulty = 0
#Difficulty
while True:
selection = raw_input("Select EASY or HARD")
if selection == "EASY":
difficulty = 10
break
else:
if selection == "HARD":
difficulty = 20
break
rand_num = random.randint(1, difficulty)
while len(guessed_nums) < allowed_guesses:
#make sure this section updates based on difficulty selection.
guess = input("Guess a number between 1 and {}.".format(difficulty)) #user guesses number
try:
player_num = int(guess)
except:
print("That's not a whole number!")
break
#make sure this section updates based on difficulty selection.
if not player_num > 0 or not player_num <= difficulty:
print("Please guess a number between 1 and {}".format(difficulty))
continue
guessed_nums.append(player_num)
if player_num == rand_num:
print("You Win! My number was {}.".format(rand_num))
print("It took you {} tries.".format(len(guessed_nums)))
break
else:
if rand_num > player_num:
print("Nope! My number is higher than {}. Guess #{}".format(player_num, len(guessed_nums)))
else:
print("Nope! My number is lower than {}. Guess #{}".format(player_num, len(guessed_nums)))
continue
if not rand_num in guessed_nums:
print("Sorry... My number was {}".format(rand_num))