Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial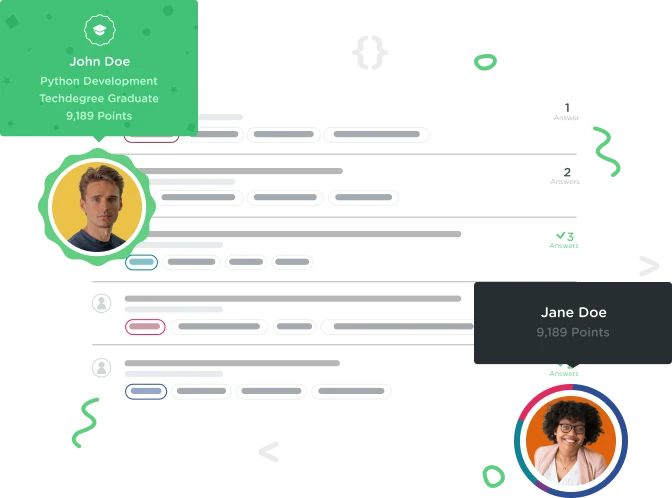
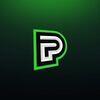
Pratham Mishra
14,191 PointsAdded a minor but a helpful feature for the Hangman game from Java Objects
When I was playing this game, I got an idea about the input word, which you've to guess. I thought why not add a feature which can actually hide the word you enter for the other person to guess, so there's no way possible he could cheat in the game.
Here's what my code looks like:-
The Hangman.java file: import java.io.*; import java.util.Scanner; public class Hangman {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
Console cs = System.console();
System.out.print("Please enter your name: ");
String name = sc.nextLine();
System.out.printf("Hi there %s, get started by entering the secret word: ", name);
char[] secretWord = cs.readPassword();
System.out.println();
String word = String.valueOf(secretWord);
Game game = new Game(word);
Prompter prompter = new Prompter(game);
while(game.getRemainingTries() > 0 && !game.isWon()) {
prompter.displayProgress();
prompter.promptForGuess();
}
prompter.displayOutcome();
}
}
The Game.java file:-
public class Game { public static final int MAX_MISSES = 7; private String answer; private String hits; private String misses;
public Game(String answer) {
this.answer = answer.toLowerCase();
hits = "";
misses = "";
}
public String getAnswer() {
return answer;
}
private char normalizedGuess(char letter) {
if (! Character.isLetter(letter)) {
throw new IllegalArgumentException("A letter is required");
}
letter = Character.toLowerCase(letter);
if (misses.indexOf(letter) != -1 || hits.indexOf(letter) != -1) {
throw new IllegalArgumentException(letter+" has already been guessed");
}
return letter;
}
public boolean applyGuess(String letters) {
if(letters.length() == 0) {
throw new IllegalArgumentException("No letter found");
}
return applyGuess(letters.charAt(0));
}
public boolean applyGuess(char letter) {
letter = normalizedGuess(letter);
boolean isHit = answer.indexOf(letter) != -1;
if(isHit) {
hits += letter;
} else {
misses += letter;
}
return isHit;
}
public int getRemainingTries() {
return MAX_MISSES - misses.length();
}
public String getCurrentProgress() {
String progress = "";
for (char letter: answer.toCharArray()) {
char display = '_';
if (hits.indexOf(letter) != -1) {
display = letter;
}
progress += display;
}
return progress;
}
public boolean isWon() {
return getCurrentProgress().indexOf('_') == -1;
}
}
The Prompter.java file:-
import java.util.*;
public class Prompter { private Game game;
public Prompter(Game game) {
this.game = game;
}
public boolean promptForGuess() {
Scanner sc = new Scanner(System.in);
boolean isHit = false;
boolean isAcceptable = false;
do {
System.out.print("Enter a letter: ");
String guessInput = sc.nextLine();
System.out.println();
try {
isHit = game.applyGuess(guessInput);
isAcceptable = true;
} catch (IllegalArgumentException iae) {
System.out.printf("%s. Please try again. %n", iae.getMessage());
}
} while(! isAcceptable);
return isHit;
}
public void displayProgress() {
System.out.printf("You have %d tries left to solve: %s%n", game.getRemainingTries(), game.getCurrentProgress());
}
public void displayOutcome() {
if(game.isWon()) {
System.out.println("----------------");
System.out.printf("Word: %s %n", game.getAnswer());
System.out.println("----------------");
System.out.printf("Congratulations you won with %d tries remaining. %n", game.getRemainingTries());
System.out.println();
} else {
System.out.printf("Bummer the word was %s. :( %n", game.getAnswer());
}
}
}
I thought this would be helpful and could give you guys an idea about the modifications you can make within the code.
I'll be more than happy to see any new ideas or modifications that can be made to this code.