Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial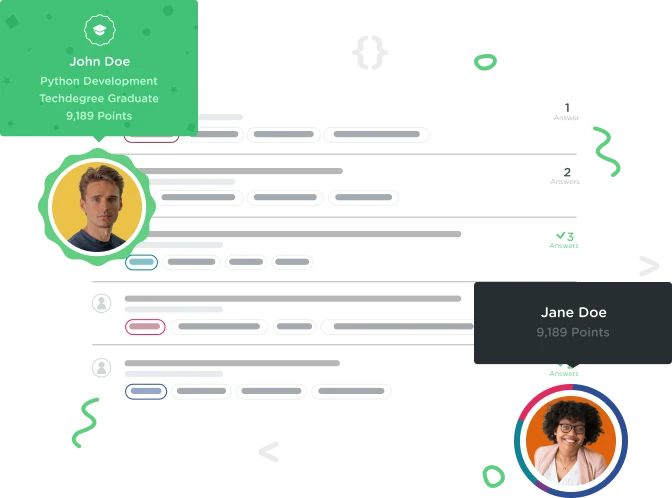
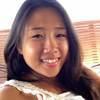
Asia Chan
11,007 PointsAdded the #username as one of the prerequisites to submit. Critique my work, please?
Here's my continuing what Andrew suggested:
//Problem: Hints are shown even when form is valid
//Solution: Hide and show span at appropriate times
//Hide hints
$("form span").hide();
var $username = $("#username");
var $password = $("#password");
var $confirmPassword = $("#confirm_password");
var $submitButton = $("#submitButton");
//Functions
function usernamePresent() {
return $username.val() !== "";
}
function isPasswordValid() {
return $password.val().length > 8;
}
function arePasswordsMatching() {
return $confirmPassword.val() === $password.val();
}
//To make sure that we can submit once the 2 conditions above (password is valid && password + confirmation pw are matching)
function canSubmit() {
return isPasswordValid() && arePasswordsMatching() && usernamePresent();
}
function passwordEvent() {
//Find out if password is valid
if (isPasswordValid()) {
//Hide hint if valid
$password.next().hide();
} else {
//else show hint
$password.next().show();
}
}
function confirmPasswordEvent() {
//Find out if password & confirmation match
if (arePasswordsMatching()) {
//Hide hint if match
$confirmPassword.next().hide();
} else {
//else show hint
$confirmPassword.next().show();
}
}
function enableSubmitEvent() {
$submitButton.prop("disabled", !canSubmit());
}
//Events to enable Submit
$username.keyup(enableSubmitEvent);
//When event happens on password input, hint shows
$password.focus(passwordEvent).keyup(passwordEvent).keyup(confirmPasswordEvent).keyup(enableSubmitEvent);
//When event happens on confirmation password input, hint shows
$confirmPassword.focus(confirmPasswordEvent).keyup(confirmPasswordEvent).keyup(enableSubmitEvent);
enableSubmitEvent();
2 Answers
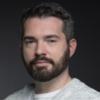
Cory Harkins
16,500 PointsI like the point of view!
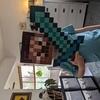
Gabbie Metheny
33,778 PointsThat's pretty much what I did, and it should work! The only thing I did differently was using the length of the username rather than comparing against an empty string. So this works, too:
function isUsernameValid() {
return $username.val().length > 0;
I also added some styling to the css to make it apparent when the button was disabled, using the 'disabled' pseudoclass:
input[type="submit"]:disabled {
background: #777;
color: #ccc;
box-shadow: 0 3px 0 0 #444;
cursor: default;
}