Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial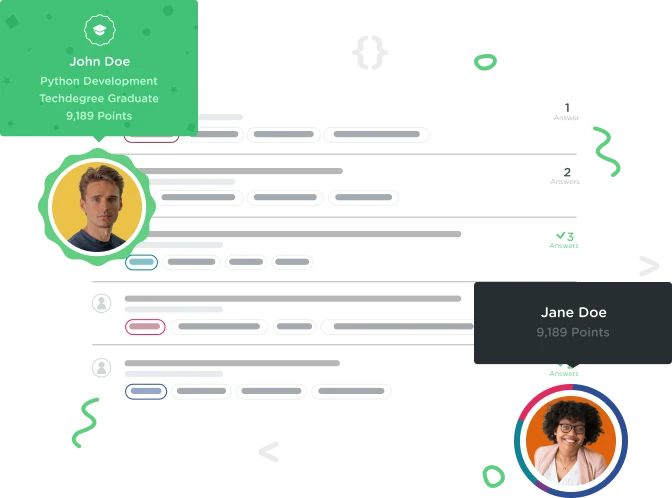

Alberto Chonillo
2,719 PointsAdded 'undefined' to preview.
My code seems to be working but when I click preview, there's an 'undefined' at the beginning of the list of questions I got right or wrong. What am I doing wrong here?
var counter = 0;
var correctAnswer = 0;
var incorrectAnswer = 0;
var questionsRight;
var questionsWrong;
function print(message) {
document.write(message);
}
var quiz = [
[ 'What color is the sky?', 'blue' ],
[ 'What is the capital of New York?', 'albany' ],
[ 'What state is Disney World located in?', 'florida' ]
];
for (var i = 0; i < quiz.length; i += 1) {
var answer = prompt(quiz[i][0]);
if (answer.toLowerCase() !== quiz[i][1] ) {
questionsWrong += '<li>' + quiz[i][0] + '</li>';
} else {
questionsRight += '<li>' + quiz[i][0] + '</li>';
correctAnswer +=1;
}
}
print('<p>You got ' + correctAnswer + ' question(s) right.</p>');
print('<p><b>You got these questions correct: </b></p><p><ol>' + questionsRight + '</ol></p>');
print('<p><b>You got these questions wrong: </b></p><p><ol>' + questionsWrong + '</ol></p>');
1 Answer
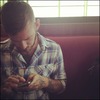
Erik McClintock
45,783 PointsAlberto,
This seems to be happening because you're not setting your variables equal to anything prior to appending content to them. At the top of your document, you have declared the variables, but not assigned them anything. Then, down in your for
loop, you are using the addition assignment operator to add any given correct or incorrect question to the appropriate variables. This is adding values to those variables now, but in addition to what was already there, which in this case, was an undefined value/type. The simplest fix here is to set both of your variables equal to empty strings. This way, you will be adding values to nothing, rather than to undefined.
So, at the top of your file, just do this:
var questionsRight = '';
var questionsWrong = '';
And you'll be good to go, regardless of whether either variable ends up having content or not! Say someone ends up getting all the questions correct, and thus the questionsWrong variable remains empty? That's just fine, and it will print nothing to the screen, because the value stored inside that variable is an empty string. If you leave the variable as simply a declaration without any value assigned to it, JavaScript sees it as being undefined, because, well...it is undefined :)
Erik
Alberto Chonillo
2,719 PointsAlberto Chonillo
2,719 PointsDoh! How did I not see that? I'm pretty sure I'll never do that again. Thank you for the answer!