Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial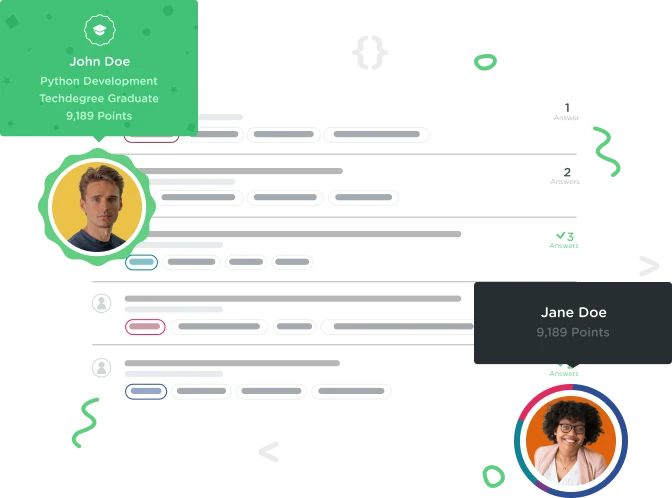

Larry Coonrod
5,041 Points.addeventlistener code works with 'var' but not 'const'
I am coding a To-Do-List page with Brackets using the technique from Javascript and the dom. If I use const to set the variable the Chrome console log shows these three error messages:
1.main.js:1 Uncaught SyntaxError: Identifier 'input' has already been declared at main.js:1 (anonymous) @ main.js:1
2 Uncaught TypeError: Cannot read property 'addEventListener' of null at main.js:9
If I replace "const"with "var" the code runs fine and changes the list name. This is the exact coding from 'Javascript and the Dom' tutorial.
I have spent many frustrating hours on this. If someone could explain why var works when const doesn't, I would be eternally grateful.
This doesn't work:
const input = document.querySelector('input');
const p = document.querySelector('p.description');
const button = document.querySelector('button');
button.addEventListener ('click',() => {
p.textContent = input.value;
});
This works:
var input = document.querySelector('input');
var p = document.querySelector('p.description');
var button = document.querySelector('button');
button.addEventListener ('click',() => {
p.textContent = input.value;
});
'''
```html
<!doctype html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<link rel="stylesheet" href="styles.css">
<script src="main.js"></script>
</head>
<body>
<h1 id="myHeading">To Do List</h1>
<p class="description">The Name of My List</p>
<input type="text" class="description">
<button class="description">Name Your List</button>
<ul>
<li>Clean House</li>
<li>Mow Lawn</li>
<li>Wash Dishes</li>
<li>Mop Floor</li>
</ul>
<script src="main.js"></script>
</body>
</html>
3 Answers

Leandro Botella Penalva
17,618 PointsHi Larry,
First of all, you are importing the script twice, at the head and the end of the body so the script is run twice. According to your code, if you run the script in the head it will fail no matter if you use var or const because the DOM of your webpage is not loaded yet at that point. However, it works using var because the second time the script is ran at the end of the body. With var the variables are declared again overriding the null values from the first time. With const fails because const means constant so once it is declared no other value can be assigned so the second time the script runs it fails to assign the values again and therefore they keep their null values from the first run of the script.
Removing the script import from your head should work both, using var and const.
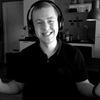
Rune Andreas Nielsen
5,354 PointsHi, Larry.
The issue is that you load your script two times. Inside of the head tag and just before the body tag ends.
If you use const, you cannot overwrite the value after it has been set, it does this in your code, because you load the script to times, but using var it is allowed, therefore your code works fine using var.
If you remove the loading of main.js from the head tag, and set the values to const, your application should work. :-)

Larry Coonrod
5,041 PointsThanks so much Rune and Leandro. Guess I had tunnel vision. Just started using brackets and didn't realize its HTML template was automatically putting the JS script in the head.