Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial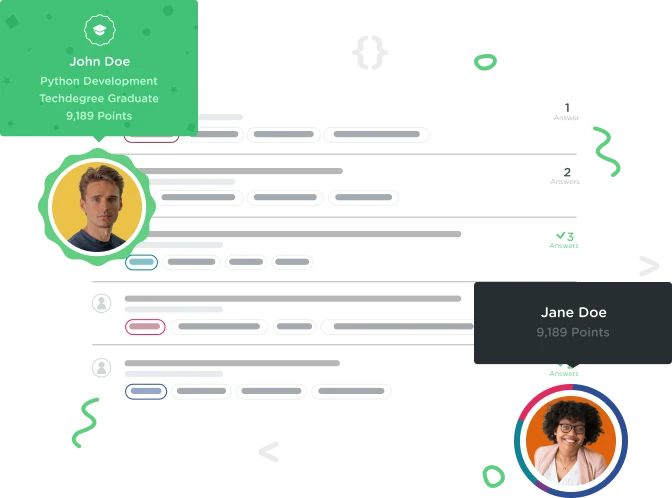

Lukasz Walczak
6,620 PointsaddForgottenVideo - index out of bounds exception
Hi, hello and welcome!
So I'm trying to solve task no1. 1st TODO seems to be fine.
2nd one is asking to add the video to (I assume) to the mVideos. Since mVideos are only declared (interface only), so I tried to initialise it and implement ArrayList interface, which is resizable, but then I get index out of bounds exception.
Any ideas, guys?
Many thanks in advance
package com.example.model;
import java.util.List;
public class Course {
private String mName;
private List<Video> mVideos;
public Course(String name, List<Video> videos) {
mName = name;
mVideos = videos;
}
public String getName() {
return mName;
}
public List<Video> getVideos() {
return mVideos;
}
}
package com.example.model;
public class Video {
private String mTitle;
public Video(String title) {
mTitle = title;
}
public String getTitle() {
return mTitle;
}
public void setTitle(String title) {
mTitle = title;
}
}
import com.example.model.Course;
import com.example.model.Video;
import java.util.Map;
import java.util.ArrayList;
import java.util.List;
public class QuickFix {
public void addForgottenVideo(Course course) {
// TODO(1): Create a new video called "The Beginning Bits"
Video video = new Video("The Beginning Bits");
// TODO(2): Add the newly created video to the course videos as the second video.
List<Video> mVideos = new ArrayList<Video>();
mVideos.add(1,video);
}
public void fixVideoTitle(Course course, String oldTitle, String newTitle) {
}
public Map<String, Video> videosByTitle(Course course) {
return null;
}
}
2 Answers

Benyam Ephrem
16,505 PointsSo what you are asked to do is add the video as the second video in the list in the course object passed in. The Course class has a field that looks like this:
private List<Video> mVideos;
It is private so we cannot directly access it with dot notation like this:
course.mVideos.add(1, video);
So we must use the getter provided to use to interface (interact) with the instance field mVideos. So what we need to do is:
- Specify the course object we want to operate on, in this case > course
- We need to get the video list for the course, in this case we use > .getVideos()
- We need to add the video to the 2nd position (index 1) (you did this right) > .add(1, video);
So all chained together here is what you need to do:
course.getVideos().add(1, video);
Java was confusing as hell for me to learn at first, but everyday you work at it, it just gets easier and easier until it all flows just like English.
Good work, keep at it

Lukasz Walczak
6,620 PointsWorked like a charm! Many thanks!