Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial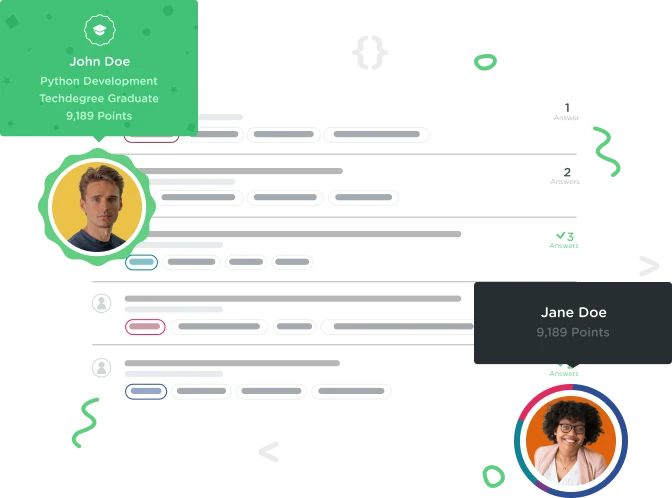

ianedwards4
1,576 PointsAdding a "back" button to the "treetunes" player
Does anyone know how you would add a "back" button to the interface of the treetunes fake media player? I understand how to add a new button in the html and change app.js, but what code would you use in playlist.js?
Here is the 'next' code from playlist.js, which is what needs to be modified:
Playlist.prototype.next = function() {
this.stop();
this.nowPlayingIndex++;
if(this.nowPlayingIndex === this.songs.length) {
this.nowPlayingIndex = 0;
}
this.play();
};
I think this part for a back button would be something like...
Playlist.prototype.back = function() {
this.stop();
this.nowPlayingIndex = ???;
if(this.nowPlayingIndex === 0) {
this.nowPlayingIndex = ???;
}
this.play();
};
Any ideas? Thanks.
2 Answers

James Anwyl
Full Stack JavaScript Techdegree Graduate 49,960 PointsHere what I came up with:
Playlist.prototype.back = function() {
this.stop();
this.nowPlayingIndex--;
if(this.nowPlayingIndex <= -1) {
this.nowPlayingIndex += this.songs.length;
}
this.play();
};
Some explanation:
this.nowPlayingIndex--;
Subtract 1 from the index.
this.nowPlayingIndex += this.songs.length;
Says: when the index equals -1 (i.e when you press back on the first song in the list), set nowPlayingIndex equal to 0 + length of songs array (which will take us to the last song in the list)
Hope this helps :)
EDIT: also fixed the formatting of your code, see here for a guide to using markdown on the forums

Chris Dyer
18,518 PointsThis is a bit more verbose for new learners. Syntactically James is correct. This just reads a bit easier for me.
Playlist.prototype.back = function() {
// Stop the current track.
this.stop();
// If the index is equal to zero, move index to the element at the end of the list.
if (this.nowPlayingIndex === 0) {
this.nowPlayingIndex = (this.songs.length - 1);
// Otherwise, we are safe to decrement.
} else {
this.nowPlayingIndex--;
}
// Kick back on the tunes.
this.play();
}
ianedwards4
1,576 Pointsianedwards4
1,576 PointsThanks for this answer, that totally works. Thanks a lot !
James Anwyl
Full Stack JavaScript Techdegree Graduate 49,960 PointsJames Anwyl
Full Stack JavaScript Techdegree Graduate 49,960 PointsIn what way does it break the interface?
Are you including this line as well:
I tested it in Chrome, Firefox and Edge and it works fine for me.
Hope this helps :)
EDIT: Ah awesome, glad you got it working :)