Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial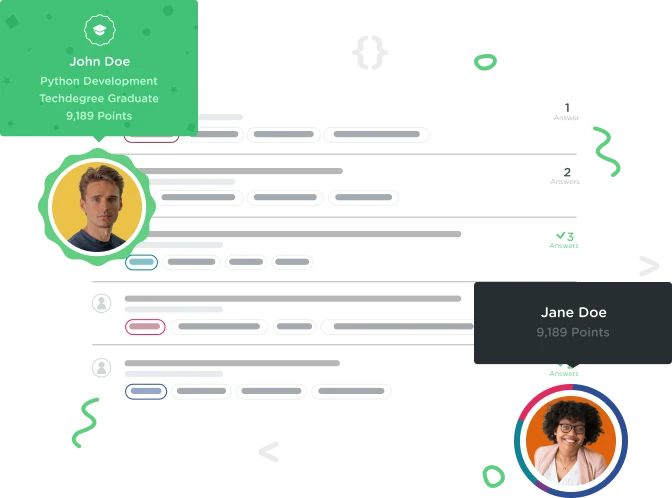
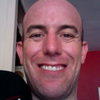
James Whiting
39,124 PointsAdding a method test?
This does work, just not in this test. Why not?
class Store:
open = 9
close = 9
def hours(open=open,close=close):
return "We're open from {} to {}.".format(open,close)
2 Answers
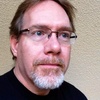
Chris Freeman
Treehouse Moderator 68,454 PointsA method needs to have the instance reference as its first argument:
class Store:
open = 9
close = 9
def hours(self, open=open,close=close):
return "We're open from {} to {}.".format(open,close)
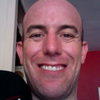
James Whiting
39,124 PointsThis has to do with reassigning the variables. It is a local vs global scope understanding.
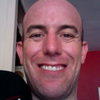
James Whiting
39,124 PointsThis is what is really going on.
Example:
class Store:
open = 9
close = 9
def hours(openhours=open,closehours=close):
return "We're open from {} to {}.".format(openhours,closehours)
[MOD: added ```python formatting -cf]
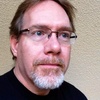
Chris Freeman
Treehouse Moderator 68,454 PointsI've updated my previous answer with notes on not using an instance parameter.
James Whiting
39,124 PointsJames Whiting
39,124 PointsCan the instance reference ever be the (First Position)+1 ... argument? Thanks for all your help today.
Chris Nelson
4,603 PointsChris Nelson
4,603 PointsI don't understand why you need (self, open=open, close=close) as the arguments in the method. Can someone please explain?
Chris Freeman
Treehouse Moderator 68,454 PointsChris Freeman
Treehouse Moderator 68,454 PointsFor a bound method (meaning the method is defined within the class definition), the first parameter is expected to be a placeholder for the instance of that class for regular methods or a placeholder for the class in a
classmethod
.These placeholders are used to hold an pointer to the specific instance (or class) the method belongs to. This is to allow methods a "hook" to use when operating on this instance (or class). The name of the placeholder can be anything, such a "this", "bob", etc. but the standard in Python is to use "self". This placeholder must be the first parameter. This first parameter is assigned this pointer.
Using your original code:
As to Chris' comment, you don't have to define the
hours
method using the 'open' and 'close' parameters. Two options:Option 1 using 'open' and 'close' parameters
By using the 'open' and 'close' parameters and assigning them the defaults of the attributes
open
andclose
, these values can be used directly in the format statement....".format(open, close)
Option 2 omitting the 'open' and 'close' parameters
By not using the 'open' and 'close' parameters, these values must be obtained from the instance using the
self
instance pointer for use in the format statement....".format(self.open, self.close)
. This passes the challenge:Both options could be also be done to allow the method to accept an argument that overrides the instance value.