Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial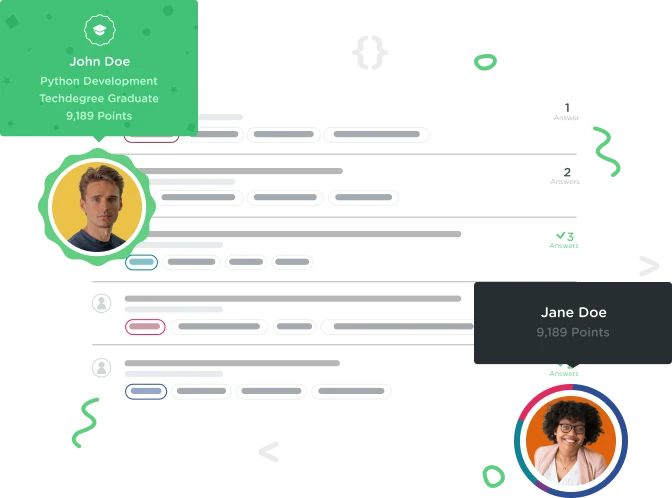

Daniel Salvatori
2,658 PointsAdding a method to multiple objects within an array
Hello, I need help with a task.
Say i have an array which contains a large number of objects. How can I create function which will add a method to multiple (or even all) objects with in the array?
For example a simple print method.
Thank you
2 Answers
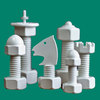
Steven Parker
231,275 PointsIf these objects are all instances of a particular class, you can add the method to the class prototype which will give it to every item at once:
// add a "print" method to every instance of "thing":
thing.prototype.print = function (arg) {
/* your method body code */
}
If you need more specific help, please show your code.

Daniel Salvatori
2,658 PointsGreat, one question remaining would be how to access the values inside the "actors" array and get them to print using this. method?
I've created a function- getActors(title) - to print these values previously but i do not know how to apply it to the context of this method.
here is the code for getActors if interested
var actorNameArray = [];
var actorFirstObj = '';
function getActors (title){
for ( var i = 0; i < movieData.length; i ++){
if (title === movieData[i].title){
for(var j = 0; j < movieData[i].actors.length; j++)
actorFirstObj += movieData[i].actors[j].firstName + ' ' +movieData[i].actors[j].lastName + ', ';
actorNameArray.push(actorFirstObj);
for(var k = 0; k < actorNameArray.length; k++){
console.log(actorNameArray[k]);
}
}
}
}
getActors('Back to the Future');
using getActors.this(title) prints every actor from every movie for each instance.
Thanks in advance.
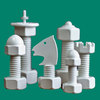
Steven Parker
231,275 PointsThe "getActors" is already an example of the kind of function I was suggesting. If you want the output to go to the page you could substitute "document.write
" for "console.log
":
document.write(actorNameArray[k]);
I tested it with the previously supplied data:
getActors("The Lord of the Rings: The Two Towers");
Daniel Salvatori
2,658 PointsDaniel Salvatori
2,658 PointsHi Steven,
thanks for the reply.
The Array looks like this:
And I want to have the method print all the details of each movie.
Steven Parker
231,275 PointsSteven Parker
231,275 PointsThese are anonymous objects. So you could create a definition for them, and then define the data as new instances of that type, or you could create the prototype method on the generic object "Object":
Also, instead of a method you could create an ordinary function that takes one of these objects as an argument:
Daniel Salvatori
2,658 PointsDaniel Salvatori
2,658 PointsI appreciate your help Steven, thank you!