Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial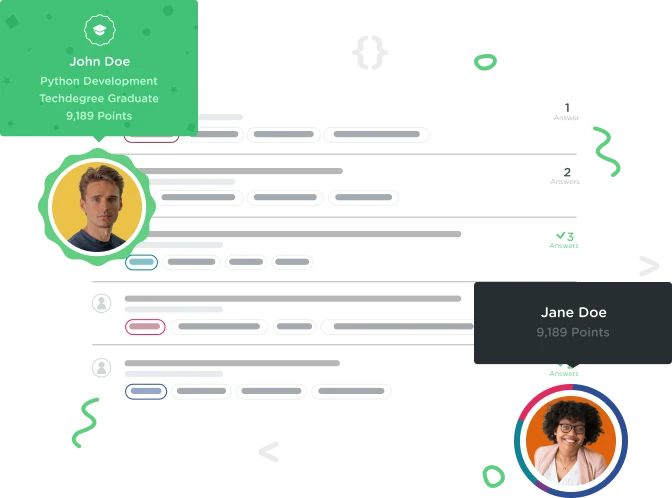
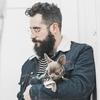
Alexander D
8,685 PointsAdding a random RGB generator function when clicking on the button. It doesn't work!
Hello there,
I'll be honest here and I am running ahead. I am looking at my past notes and I finally wanted to implement a random RGB generator function in the DOM. However, it doesn't work. My code is the following:
var red= " ";
var green = '';
var blue = '';
var rgbColor = " ";
red = Math.floor(Math.random() * 256 );
green = Math.floor(Math.random() * 256 );
blue = Math.floor(Math.random() * 256 );
function RandomRGB () {
rgbColor = 'rgb(' + red + ',' + green + ',' + blue + ')';
return rgbColor
}
const myHeading = document.getElementById("myHeading");
const myTextInput = document.getElementById("myTextInput");
const myButton = document.getElementById("myButton");
myButton.addEventListener("click", () => {
myHeading.style.color = RandomRGB ();
});
There's something about this code that doesn't make the program work. Even if I restore "myHeading.style.color = myTextInput.value; " the program doesn't run. There's something about the function, specifically about the string " rgbColor = 'rgb(' + red + ',' + green + ',' + blue + ')' " that is blocking the program. Not sure what that is!
Thanks!
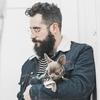
Alexander D
8,685 PointsHello Sean T. Unwin, you're correct! I've updated my code down below!
Thanks for your time!
5 Answers
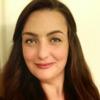
Jennifer Nordell
Treehouse TeacherHi, Alexander D! I would be willing to bet that every time you refresh the page, it's a different color, am I correct? The reason for this is that you generate the random colors once when the JS is loaded. Every time the function is called, it runs the function again, but it does not re-run the code that was used to generate those colors.
Try placing the definitions for the red
, green
and blue
variables inside your function just before the rgbColor
definition and give it a whirl!
Hope this helps!
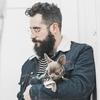
Alexander D
8,685 PointsHello Jennifer,
Thanks for your support!
The idea is to have a random color generated every time I click. With the code Guil explained, I can type any color in the text field and the H1 gets updated. So I thought I would add my random RGB function to have a random code at every click.
I saw this around before in CodePens before, but I wanted to replicate this. I'd be happy if I got any random color every time I refresh, but it's not happening.
This the updated code:
function RandomRGB () {
var red = Math.floor(Math.random() * 256 );
var green = Math.floor(Math.random() * 256 );
var blue = Math.floor(Math.random() * 256 );
var rgbColor = 'rgb(' + red + ',' + green + ',' + blue + ')';
return rgbColor
}
const myHeading = document.getElementById("myHeading");
const myTextInput = document.getElementById("myTextInput");
const myButton = document.getElementById("myButton");
myButton.addEventListener("click", () => {
myHeading.style.color = rgbColor;
});
I also change the very last string myHeading.style.color = rgbColor; with myHeading.style.color = RandomRGB () ; but I am not getting anywhere.
Thanks for looking after this!
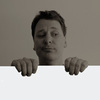
Sean T. Unwin
28,690 PointsThe function should be called, not the variable within the function, also the parentheses are missing when calling the function
myHeading.style.color = rgbColor;
should be myHeading.style.color = RandomRGB();
inside the Listener.
You had this part correct in your OP, actually.
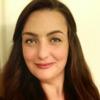
Jennifer Nordell
Treehouse TeacherI see Sean edited the comment to include the part about the function name
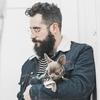
Alexander D
8,685 PointsGuys,
Thanks for your support and patience! I really appreciate it, however, It's still not working.
I followed your suggestions to call the function with parenthesis as shown below. I kept the first part unchanged, hence I didn't post it here. When I click on the button, I do not generate any random color.
const myHeading = document.getElementById("myHeading");
const myTextInput = document.getElementById("myTextInput");
const myButton = document.getElementById("myButton");
myButton.addEventListener("click", () => {
myHeading.style.color = myTextInput.value;
});
Is there anything I am doing wrong?
The interesting part is that when I replace the last part with "myHeading.style.color = myTextInput.value;" and ignore the function without referencing it all, the program doesn't work.
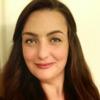
Jennifer Nordell
Treehouse TeacherAlexander D At this point, we would need to see your HTML to know for sure. Also, can you confirm that you are not using Internet Explorer?` Internet Explorer does not support arrow functions. Are you receiving any errors in your console in the developer tools?
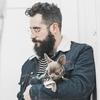
Alexander D
8,685 PointsHello Jennifer Nordell ,
The HTML is the following:
<!DOCTYPE html>
<html>
<head>
<title>JavaScript and the DOM</title>
<link rel="stylesheet" href="css/style.css">
</head>
<body>
<h1 id="myHeading">JavaScript and the DOM</h1>
<input type="text" id="myTextInput">
<button id="myButton">Change heading color</button>
<p>Making a web page interactive</p>
<script src="app.js"></script>
</body>
</html>
There's something that I can't comprehend. This is the complete code below:
//function RandomRGB () {
// var red = Math.floor(Math.random() * 256 );
// var green = Math.floor(Math.random() * 256 );
// var blue = Math.floor(Math.random() * 256 );
//
// var rgbColor = 'rgb(' + red + ',' + green + ',' + blue + ')';
// return rgbColor
//}
const myHeading = document.getElementById("myHeading");
const myTextInput = document.getElementById("myTextInput");
const myButton = document.getElementById("myButton");
myButton.addEventListener("click", () => {
myHeading.style.color = myTextInput.value;
});
This works fine only when the function is invisible. Once I remove the / / signs from the function and I make it visible, the program stops working. If I make the function active, and I call the function in the last line myHeading.style.color = RandomRGB (); it doesn't work.
It seems there's something about this function that is driving the program crazy.
I appreciate your patience!
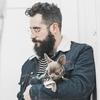
Alexander D
8,685 PointsJennifer Nordell Sean T. Unwin
The code works as a charm on code pen. For some reasons the workspace is not accepting this. Thanks again for your time!
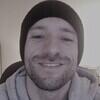
Paul Brubaker
14,290 PointsHmm looks like this thread hasn't been active for a while but oh well here's how I did it. Recommend using string interpolation rather than doing all that concatenating, it's really very difficult and not enjoyable at all to look for a typo in a monster string like that.
const myHeading = document.getElementById('myHeading');
const changeColor = document.getElementById('change-color');
const randInt = (int) => {
return Math.floor(Math.random() * int)
};
changeColor.addEventListener('click', () => {
myHeading.style.color = `rgb(${randInt(256)}, ${randInt(256)}, ${randInt(256)})`;
})
Sean T. Unwin
28,690 PointsSean T. Unwin
28,690 PointsAre you hoping for a different (random) color every button press?