Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial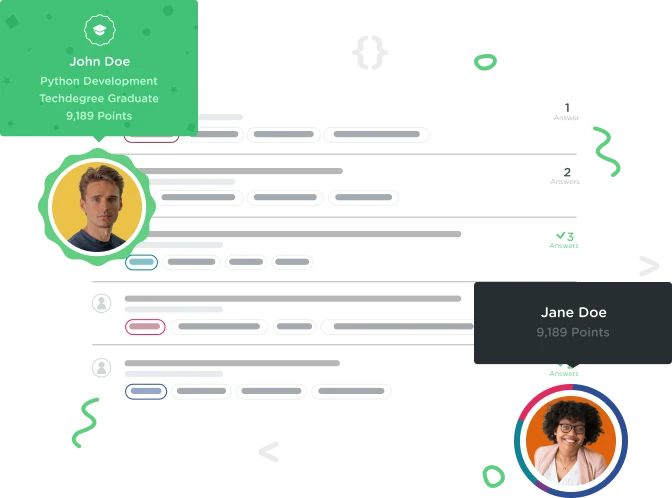
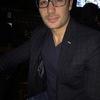
Derek Saunders
5,167 PointsAdding a search field
Would it be possible to add a search box where you could type like soccer, books, music, etc. to then generate a list of users that have the same interest? If so how would you go about doing this?
1 Answer

Geoff Parsons
11,679 PointsFor this specific example it could be achieved fairly easily by first adding a search field to your view that points to a search action in the users controller:
<%= form_for :search, url: search_users_path do |form| %>
<%= form.text_field :query %>
<% end %>
Add a route for the actions:
resources :users do
collection do
post :search
end
end
Then adding an action to the users controller that searches users by interest (assuming Interest has a field "name":
def search
@users = User.include(:interests)
.where('interests.name LIKE ?', "%#{params[:search][:query]%")
.uniq
render :index
end
This will do a partial text search of interest names submitted from the form and return all of the users which have this interested associated. If you'd like to be able to search for multiple interests at the same time you'll have to get a little fancier I'm afraid. Likewise if you plan on being able to search for users based on multiple fields you'll want to consider some full-text search options instead of writing the queries by hand. There are many options available for full-text search in rails, some of which will require other processes to be running to index the database.
Hopefully this helps for your needs though!