Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial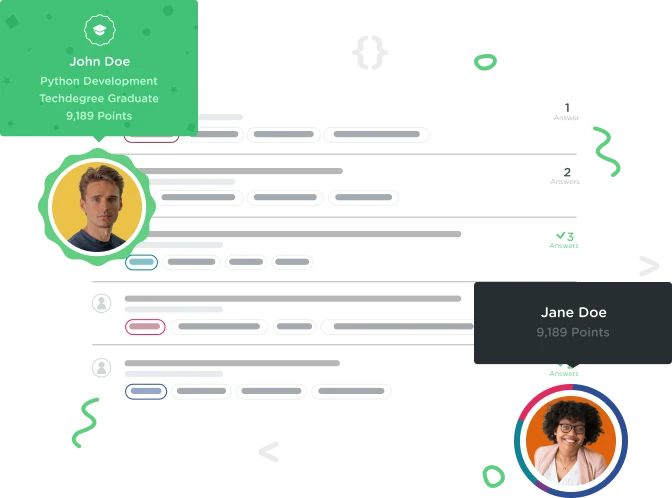
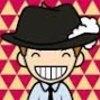
Aaron Selonke
10,323 PointsAdding a unique list item to the DOM
Here I'm following the video, but have stoped to add the functionalty to the canvas app without watching yet how Andrew does it.
You can see I'm adding a list item which adds a new color option to the palat. See the screenshot here http://uploadpie.com/J4vcn
There are two unexpected things that occur when I append the $newColorListItem to the DOM
1) When I use the jQuery formatting
var $newColorListItem = $("<li></li>");
or when I move this statement inside of the the click() event function
I can only add ONE new list item to the palet. It does not add a second or third or so on. ONLY ONE
Why is that?
///// now that the three sliders work and prduce a color and projects to
//// need to be able to add color to the list
/////////////////ADD CUSTOM COLOR TO LIST
$itemCounter = 0;
$itemID = "_"+$itemCounter;
var $newColorListItem = $("<li></li>");
$("#addNewColor").click(function(){
$(".controls ul").append($newColorListItem);
//this is to create a unique ID for the new list item
$itemCounter++;
$itemID = "_"+$itemCounter;
// now add unique ID and background color to $newColorListItem.
$($newColorListItem).attr("ID", $itemID);
console.log($newColorListItem);
});
COMPLETE CODE HERE
JS
////COLOR CONTRLS/////////////////////
//On Click of the color controls:
// Removes selected class from all of the colors
// then adds selected class to the color that was clicked
//variable to hold the color of the selected circle
var $selectedcolor = $(".selected").css("background-color");
$(".controls li").click(function(){
/*
//I used the .each() loop to cycle through the list items
$(".controls li").each(function(){
$(this).removeClass("selected");
});
*/
////Here going to use the siblings method
$(this).siblings().removeClass("selected");
$(this).addClass("selected");
//caches the current color in the $selectcolor variable
$selectedcolor = $(this).css("background-color");
});
/////////////////////////////////////////
//////////NEW COLOR BUTTON/////////////////
$("#revealColorSelect").click(function(){
$("#colorSelect").toggle();
});
//////////GIVE COLOR TO new color span from sliders///////////
//Here changes the color of the tile to red
var $newColor = $("#newColor");
function $updateColor()
{
$newColor.css("background", "rgb(" +$R+ "," +$G+ "," +$B+ ")" );
};
//////Slider Functions
// Returns values of RGB
var $R = 0;
var $G = 0;
var $B = 0;
$("#red").change(function(){
$R = $(this).val();
$updateColor();
});
$("#green").change(function(){
$G = $(this).val();
$updateColor();
});
$("#blue").change(function(){
$B = $(this).val();
$updateColor();
});
///// now that the three sliders work and prduce a color and projects to
//// need to be able to add color to the list
/////////////////ADD CUSTOM COLOR TO LIST
$itemCounter = 0;
$itemID = "_"+$itemCounter;
var $newColorListItem = $("<li></li>");
$("#addNewColor").click(function(){
$(".controls ul").append($newColorListItem);
//this is to create a unique ID for the new list item
$itemCounter++;
$itemID = "_"+$itemCounter;
// now add unique ID and background color to $newColorListItem.
$($newColorListItem).attr("ID", $itemID);
console.log($newColorListItem);
});
HTML
<!DOCTYPE html>
<html>
<head>
<title>Simple Drawing Application</title>
<link rel="stylesheet" href="css/style.css" type="text/css" media="screen" title="no title" charset="utf-8">
</head>
<body>
<canvas width="600" height="400"></canvas>
<div class="controls">
<ul>
<li class="red selected"></li>
<li class="blue"></li>
<li class="yellow"></li>
</ul>
<button id="revealColorSelect">New Color</button>
<div id="colorSelect">
<span id="newColor"></span>
<div class="sliders">
<p>
<label for="red">Red</label>
<input id="red" name="red" type="range" min=0 max=255 value=0>
</p>
<p>
<label for="green">Green</label>
<input id="green" name="green" type="range" min=0 max=255 value=0>
</p>
<p>
<label for="blue">Blue</label>
<input id="blue" name="blue" type="range" min=0 max=255 value=0>
</p>
</div>
<div>
<button id="addNewColor">Add Color</button>
</div>
</div>
</div>
<script src="http://code.jquery.com/jquery-1.11.0.min.js" type="text/javascript" charset="utf-8"></script>
<script src="js/app.js" type="text/javascript" charset="utf-8"></script>
</body>
</html>
CSS
body {
background: #384047;
font-family: sans-serif;
}
canvas {
background: #fff;
display: block;
margin: 50px auto 10px;
border-radius: 5px;
box-shadow: 0 4px 0 0 #222;
cursor: url(../img/cursor.png), crosshair;
}
.controls {
min-height: 60px;
margin: 0 auto;
width: 600px;
border-radius: 5px;
/*OVERFLOW HIDDEN*/
overflow: hidden;
}
ul {
list-style:none;
margin: 0;
float: left;
padding: 10px 0 20px;
width: 100%;
text-align: center;
}
ul li, #newColor {
display:block;
height: 54px;
width: 54px;
border-radius: 60px;
cursor: pointer;
border: 0;
box-shadow: 0 3px 0 0 #222;
}
ul li {
display: inline-block;
margin: 0 5px 10px;
}
.red {
background: #fc4c4f;
}
.blue {
background: #4fa3fc;
}
.yellow {
background: #ECD13F;
}
.selected {
border: 7px solid #fff;
width: 40px;
height: 40px;
}
button {
background: #68B25B;
box-shadow: 0 3px 0 0 #6A845F;
color: #fff;
outline: none;
cursor: pointer;
text-shadow: 0 1px #6A845F;
display: block;
font-size: 16px;
line-height: 40px;
}
#revealColorSelect {
border: none;
border-radius: 5px;
margin: 10px auto;
padding: 5px 20px;
width: 160px;
}
/* New Color Palette */
#colorSelect {
background: #fff;
border-radius: 5px;
clear: both;
margin: 20px auto 0;
padding: 10px;
width: 305px;
position: relative;
display:none;
}
#colorSelect:after {
bottom: 100%;
left: 50%;
border: solid transparent;
content: " ";
height: 0;
width: 0;
position: absolute;
pointer-events: none;
border-color: rgba(255, 255, 255, 0);
border-bottom-color: #fff;
border-width: 10px;
margin-left: -10px;
}
#newColor {
width: 80px;
height: 80px;
border-radius: 3px;
box-shadow: none;
float: left;
border: none;
margin: 10px 20px 20px 10px;
}
.sliders p {
margin: 8px 0;
vertical-align: middle;
}
.sliders label {
display: inline-block;
margin: 0 10px 0 0;
width: 35px;
font-size: 14px;
color: #6D574E;
}
.sliders input {
position: relative;
top: 2px;
}
#colorSelect button {
border: none;
border-top: 1px solid #6A845F;
border-radius: 0 0 5px 5px;
clear: both;
margin: 10px -10px -7px;
padding: 5px 10px;
width: 325px;
}
1 Answer
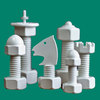
Steven Parker
230,274 PointsWhen you created your new list item, you assigned it to $newColorListItem and you did this outside the function. This makes your new element variable a global. One aspect of global variables is that there can be only one. So you can never add a second element because you never create a second element.
You should continue to the next video and watch how the example code is completed. You'll learn how to handle this issue and several other issues in your current code.