Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial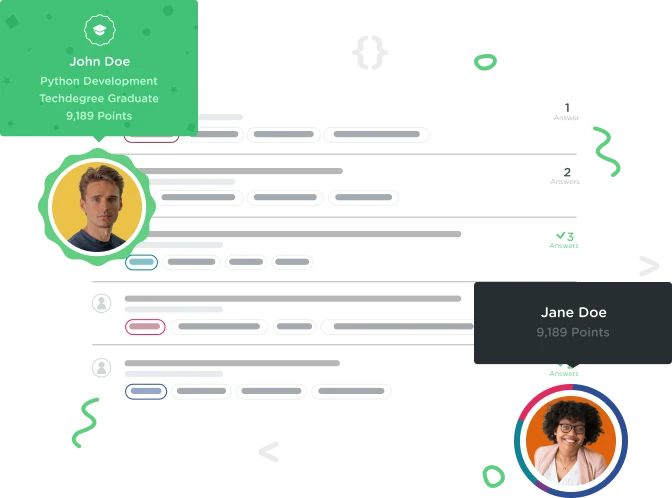
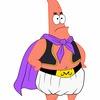
<noob />
17,062 PointsAdding an option to let the user that what they tried to remove is not on their list
def remove_from_list():
show_list()
what_to_remove = input("What would u like to remove?\n> ")
try:
shopping_list.remove(what_to_remove)
except ValueError:
pass
else:
if what_to_remove not in shopping_list:
print("{}, is not in the list, therfore u cant remove it!".format(what_to_remove)
show_list()
i dont understand what im doing wrong here , thanks in advance for help!
3 Answers
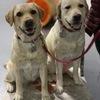
Alex Koumparos
Python Development Techdegree Student 36,887 PointsHi noob developer,
It looks like you're a little confused about the components of a try/else/except(/finally) block.
You've got the try
part right, this is where you write the line that you expect to be able to fail. Here you are removing an item from a list. That item might not be in the list, thus it might fail (with a ValueError).
You then use except
to handle the cases where the attempt from your try actually failed. In the case of removing a non-existent item from a list, this will produce a ValueError and so you handle that here. Instead of handling it, you've just written pass
.
You use else
to handle code that you want to run after the code in your try
was successful (so in this case, the item was successfully removed). This code will not run if the try
was unsuccessful. So if the item had not existed in the list, thus leading to a ValueError, the except
would execute but NOT the else
.
Lastly, and just for completeness, there is a finally
case that executes code regardless of whether the try
piece was successful.
Hope that clears everything up for you,
Cheers
Alex
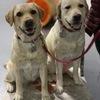
Alex Koumparos
Python Development Techdegree Student 36,887 PointsHi Noob,
You're moving in the right direction. The important thing to remember with the except
branch, is that this will only execute if the thing you wanted to do in the try
statement failed. In your case, you're trying to remove an item from a list. If the item isn't there, you get a ValueError
.
Your except
branch 'catches' that ValueError
. As such, you don't need the if
statement inside your except
. You already know that the item wasn't in the list because you're in the except
branch. Therefore, you can skip straight to the code where you respond to the absence of the item, in this case, by printing the message to the user.
Sarat Chandra is quite right to say you can solve this with a simple if/else, but since you've started down this path I think you'll learn more by seeing it through, and you're soon going to start running into try/except all the time, so the sooner you get comfortable with this construct, the happier you will be.
Cheers
Alex
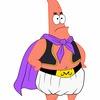
<noob />
17,062 Pointsi think i figure it out!
def remove_from_list():
show_list()
what_to_remove = input("What would u like to remove?\n> ")
try:
shopping_list.remove(what_to_remove)
except ValueError:
print("The item is not available in the list")
show_list()
Thanks for the help alex, i understand it now :D
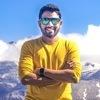
Sarat Chandra
4,898 PointsHello noob developer :) Instead of complicating things with a try block, this can be achieved with simple if and else blocks. Check below. If you have any more questions, leave a comment.
def del_list():
show_list()
del_item=input("Enter the item you wish to to delete from your shopping list. " )
if del_item in shopping_list:
shopping_list.remove(del_item)
print ("Item {} has been deleted from the list".format(del_item))
show_list()
else:
print("Item {} is not present in the list.".format(del_item))
show_help
<noob />
17,062 Points<noob />
17,062 PointsHi alex!, thanks for ur answer, but i still don't understand couple of things so i organized my questions: 1.instead of using the keyword 'pass' what i could do?
Instead of using the else statment and in the else statment using the if statment i could excute the code like this?