Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial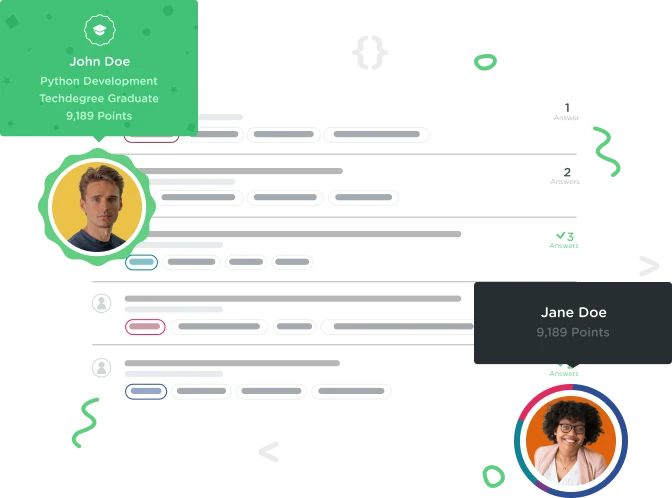

Aaryen Labana
2,586 PointsAdding and subtracting dice in my own game. Please help!
Hi all,
I've decided to make a game involving adding and subtracting dice which are from image views. I cannot think of how to do it so could anyone help me on this? Is there a way to remove the image view entirely and then bring it back, or just change the image view to match the background. thanks
2 Answers
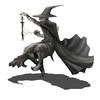
Jesus Cancino
2,494 PointsI just broke this down in playgrounds here. From what I understood. You want to randomize your array of dice. So you have your variables in a array here.
var die1 = 1
var die2 = 1
var die3 = 1
var diceArray = [die1,die2,die3]
and then you have your function that does a for loop that changes the values of each variable in the array.
func diceRandomizer2() {
for item in diceArray.indices {
diceArray[item] = Int.random(in: 1..<7)
}
print(diceArray)
}
Then you want to be able to subtract dice here.
func subtractDice() {
if diceArray.isEmpty {
print("There are no dice! Please add a dice")
} else {
diceArray.removeLast()
print("Last dice has been removed")
}
}
and add dice.
func addDice() {
diceArray.append(1)
print("A die has been added")
}
call the functions to test it out and see if that is what you want to be working. I was thinking of making the dice into a struct and have the add function create a new instance of the struct and append that to the array so your array would read [die1,die2,die3,die4] and etc.
let me know if this helps you out or no.
Thanks for asking! I am still also learning how to better write more efficient code.

Aaryen Labana
2,586 PointsHi Jesus Cancino,
thanks for the reply. However when I put it in I got an error fro. the addDice function, it say: Cannot convert value of type 'Int' to expected argument type 'UIImage'.
Aaryen Labana
2,586 PointsAaryen Labana
2,586 PointsHere is my code: When I press the button, five dice are rolled at random, but I want the option to ba able to increase/decrease the number of dice from 1-5 at most.
import UIKit
class ViewController: UIViewController {
}