Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial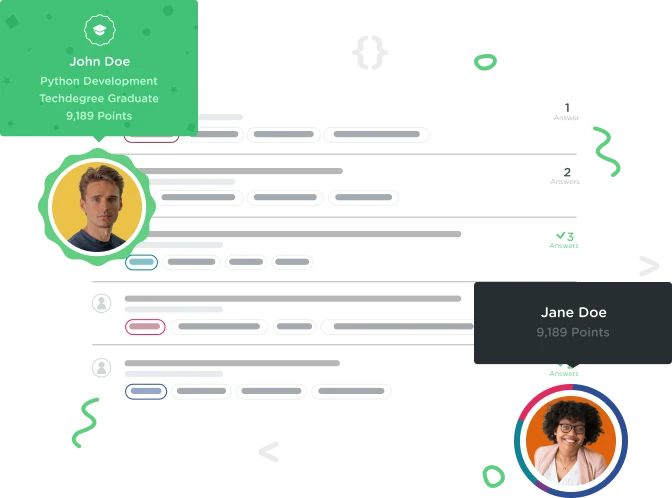

Antonio Coelho
30 PointsAdding Decimals to a Calculator made on xCode6 using swift ( made for iOS8)
Hi, I recently got into programming apps on Xcode using apples latest language swift. I'm currently only trying to make simple apps. I decided to start with a calculator and it went great , it works perfectly apart from one issue it doesn't support decimals so for example when you types in 3 / 2 , where we know the answer should be 1.5 it shows the answer as 1 as it does not understand 1.5 as a number. The rest works fine however I'm looking to adding the decimal functionality , can anyone help me?
2 Answers

Meek D
3,457 PointsI think your function should return a float. For example when you take two integers like 3 and 2 , once it is divided you will get a decimal number. Hope that helps

Meek D
3,457 PointsAlright i just got home sorry ... It is good when you start simple so I will show you an example where we have two UITextField and 4 operations buttons and the display label . So the way this calculator works the user entered two integers then pressed the operation button and the result will be displaying into the UILabel
class ViewController: UIViewController {
@IBOutlet weak var input_two: UITextField!
@IBOutlet weak var displaylabel: UILabel!
@IBOutlet weak var input_one: UITextField!
var convert : Double {
get {
return NSNumberFormatter().numberFromString(input_one.text!)!.doubleValue
}
}
var convert_two :Double {
get {
return NSNumberFormatter().numberFromString(input_two.text!)!.doubleValue
}
}
@IBAction func Addition(sender: AnyObject) {
var Addition = convert + convert_two
displaylabel.text = ("\(Addition)") // cast addition variable to string
}
@IBAction func Multiplication(sender: UIButton) {
var Multiply = convert * convert_two
displaylabel.text = ("\(Multiply)")
}
@IBAction func Subtraction(sender: AnyObject) {
var Subtraction = convert - convert_two
displaylabel.text = ("\(Subtraction)")
}
@IBAction func Division(sender:AnyObject )
{
var Division = convert / convert_two
displaylabel.text = ("\(Division)")
}
func clear_fields(){
displaylabel.text = nil
input_one.text = nil
input_two.text = nil
}
@IBAction func Clear(sender: AnyObject) {
clear_fields()
}
override func viewDidLoad() {
super.viewDidLoad()
}
The code is basically taking both inputs and change it to Double then allow us to do any operation with them . Hope that helps
Antonio Coelho
30 PointsAntonio Coelho
30 PointsHow do I do this though ? How do I make it return a float?
Meek D
3,457 PointsMeek D
3,457 Pointsfunc (input_one : Int , input_two :Int ) ->Float { return input_one + input_two }
Antonio Coelho
30 PointsAntonio Coelho
30 PointsHi, I'm really starting swift so I'm kinda confused. Maybe if I shoe you my code and you tell me what to change? class ViewController: UIViewController {