Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial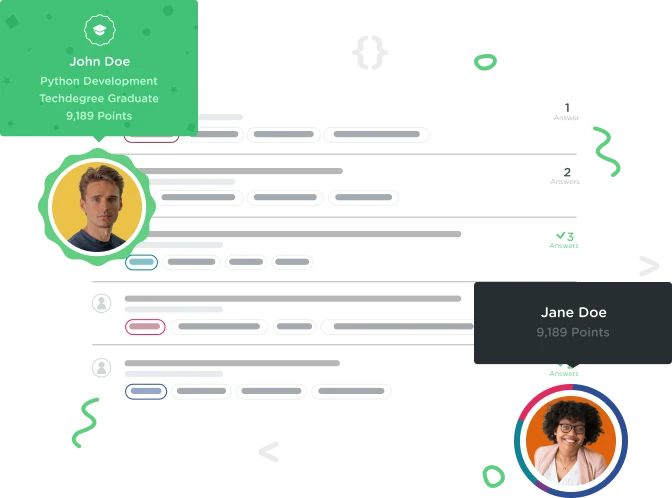

Emin Grbo
13,092 PointsAdding Integers and Strings (converting back and forth)
Ok, so i am on a Swift course and wanted to practice some of my "projects" to see if i got everything down. I ran into an error that i don't quite understand. I also realize that there is probably a much better way to do this, but here it is :)
I have 2 labels which show a random number at the press of a button and a text field that shows a result of adding those two numbers.
Main issue is that i get a String from the random number generator so i can put it inside the label, and then i need to convert it to Int so i can ad it, and then back to String so i can put that in the text field.
I managed to make it work, after xCode suggested i add "!" in two places as you can see below. But i have no idea what it means ? Error before that was: "value of optional type string not unwrapped, use "!" or "?" "
@IBAction func btnPress(_ sender: Any) {
numberLabel.text = randomNum()
numberLabel2.text = randomNum()
result = Int(numberLabel.text!)! + Int(numberLabel2.text!)!
txtField.text = String(result)
}
My random function just in case you need it:
func randomNum() -> String{
// OPTION 1
//let number = GKRandomSource.sharedRandom().nextInt(upperBound: 1000)
let number = arc4random_uniform(6)
return String(number)
}
I tried to search online and get some sense of it, but still not getting it 100%. I hope someone can let me know what it means and when it is used.
Cheers !
3 Answers
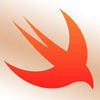
Jeff McDivitt
23,970 PointsHi Erin -
The randomNumber is optional value using your example so you are force unwrapping it by adding the exclamation point. This is not good practice because if you forced unwrap an optional that is nil, a runtime error is thrown and the process or application is terminated. Lets say for some reason your random number did not appear in the first or second label, by using the exclamation point you are force unwrapping a value that may not be there

Emin Grbo
13,092 PointsYes, i found something similar online, but now it is a bit more clearer as well :) Thank you !
Just wondering, why "!" in two places ? I get that i need to use it INSIDE the parenthesis, but it seems every time i want to access the value i need to unwrap it so i use it OUTSIDE as well ?
So once i unwrap it for one method (".text") i need to unwrap it again for Int ? Not sure if this makes sense, but i am just trying to make sure i know how to handle this in the future :)
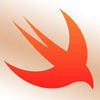
Jeff McDivitt
23,970 PointsPersonally I would not force unwrap anything in my code unless I absolutely new that there was going to be a value there. In your example I would use some error handling to make sure that if there was an error I would catch it

Emin Grbo
13,092 PointsAwesome man, tnx for the info !