Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial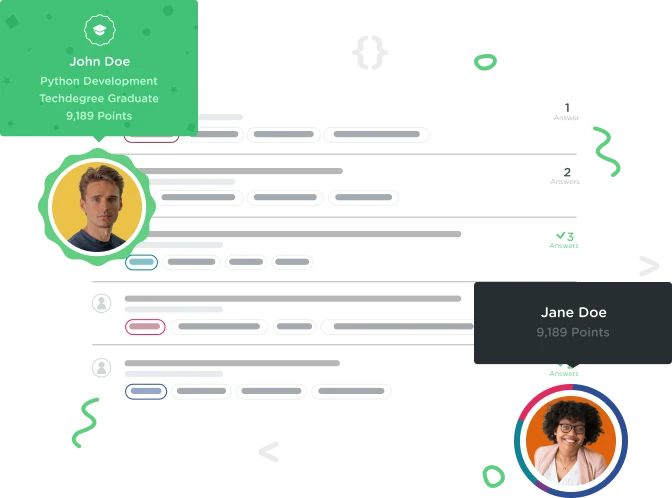

richi richi
552 PointsAdding methods to the class
Hello, I wrote the same code as Ahsley (it seems so) and I have a inquiry.
class Pet { constructor(animal, age, breed, sound){ this.animal = animal; this.age = age; this.breed = breed; this.sound = sound; } //Adding methods to the class speak(){ console.log(this.sound); } }
const ernie = new Pet('dog', 1, 'pug', 'yip yip'); const vera = new Pet('dog', 6, 'border collie', 'woof woof');
//console.log(vera);
ernie.speak(); vera.speak();
Why kind of scope the sound parameter inside the constructor have? Why can be used in a different method than constructor? Thanks guys!
2 Answers
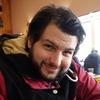
Eric M
11,546 PointsHi Richi,
To start with, let's make this code readable! (I also removed a line that you commented out)
class Pet
{
constructor(animal, age, breed, sound)
{
this.animal = animal;
this.age = age;
this.breed = breed;
this.sound = sound;
}
//Adding methods to the class
speak()
{
console.log(this.sound);
}
}
const ernie = new Pet('dog', 1, 'pug', 'yip yip');
const vera = new Pet('dog', 6, 'border collie', 'woof woof');
ernie.speak(); vera.speak();
Phew, that's nicer. Now I can see what's going on.
Okay, so what kind of scope does the sound parameter have? This is a really good question and its great that you're thinking about how classes work.
Let's change the syntax a bit to avoid confusion. Let's say we're working with this.sound = argument_four
. Here, sound is a property of Pet and its value is being set to the argument passed to the constructor. In short, sound is scoped to the Pet object created by the class.
That's scope, but your question hints other aspects of object oriented programming, privacy and inheritence. In JavaScript (unlike other OOP languages such as Java) class properties are all public! This means I could simply write console.log(vera.sound)
from anywhere that has vera in scope.
There is a (complicated, advanced) way to make JavaScript class properties private, but it requires other tools and code build steps to essentially extend the JavaScript language.
If we were coding in Java instead we would be able to make the class properties one of public, private, or protected. Here, public means they would work the same as JavaScript - they can be accessed however you like. Private means they can only be used by that class.
You might find it interesting that even if this.sound
was private the speak()
method could still access it, because speak()
is a method of class Pet, so it can access Pet's private properties and methods. It would only be other functions and classes that couldn't access Pet's private properties.
Protected means that it's private, unless a subclass wants to access it. Don't worry too much about this (or any of this part about Java really).
I hope that answers your question and isn't an information overload. Let me know if you need any more info :)
Cheers,
Eric

richi richi
552 PointsMany thanks Eric, I'm very sorry for the format of my code. Once I copied and paste it it totally changes... How can I make the code shows in the format you showed us? inside the black box. Thanks!
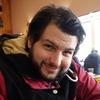
Eric M
11,546 PointsHi Richi,
Below each text box where you compose your post there should be a link to a Markdown Cheatsheet.
Markdown is an inline styling language used for formatting text. It's a popular format that has become the standard for Readme files for github repos, as one example.
To format code, you use three backticks followed by the language name, then your code, then enclose with another three backticks at the end
e.g:
```JavaScript
Your code here
```